Python NumPy numpy.sort() Function
-
Syntax of
numpy.sort()
-
Example Codes:
numpy.sort()
-
Example Codes:
numpy.sort()
to Sort a Multi-Dimensional Array -
Example Codes:
numpy.sort()
to Sort a Multi-Dimensional Array Along a Specified Axis -
Example Codes:
numpy.sort()
to Sort Different Types of Arrays
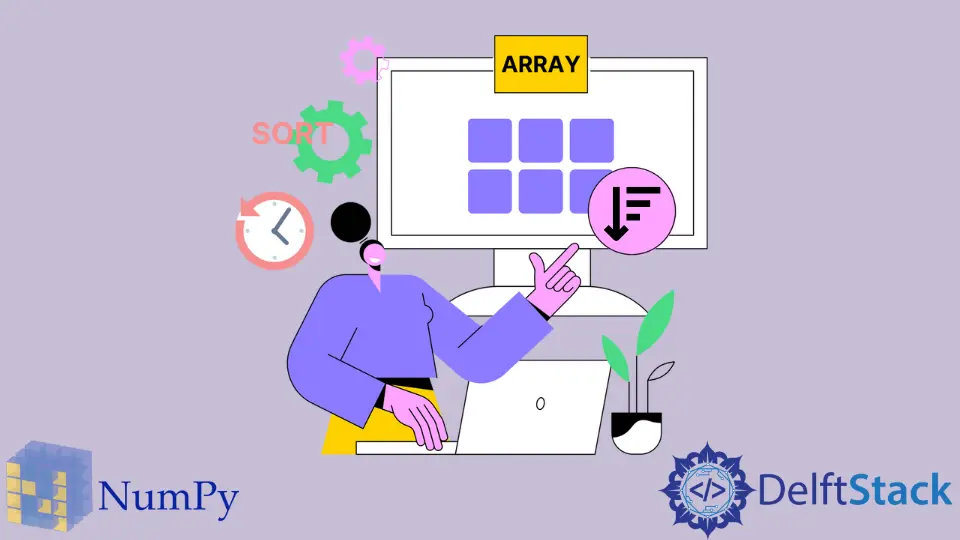
Python NumPy numpy.sort()
function sorts an N-dimensional array of any data type. The function sorts the array in ascending order by default.
Syntax of numpy.sort()
numpy.sort(a, axis=-1, kind=None, order=None)
Parameters
a |
It is an array-like structure. It is the input array to sort. |
axis |
It is an integer. It represents the axis along which the function will sort the array. Its default value is -1 which means that the function will sort the array along the last axis i.e in ascending order. If it is None , the function will convert the multi-dimensional array to one-dimensional before sorting. If it is 0, the function will sort the array along the first axis i.e in descending order. |
kind |
It is a string. It represents the name of the sorting algorithm. The sorting algorithm names accepted by this function are quicksort , mergesort , heapsort , and stable . To read more about the time complexities of these sorting algorithms, click here. |
order |
It is a string or a list of strings. When the fields of an array are defined, this parameter is used to specify the field to compare first. |
Return
It returns a sorted array of the same type and shape as the input array.
Example Codes: numpy.sort()
The parameter a
is mandatory. If we execute this function on a one-dimensional array, it generates the following output.
import numpy as np
a = np.array([89, 34, 56, 87, 90, 23, 45, 12, 65, 78, 9, 34, 12, 11, 2, 65, 78, 82, 28, 78])
sorted_array = np.sort(a)
print('The sorted array is:')
print(sorted_array)
Output:
The sorted array is:
[2 9 11 12 12 23 28 34 34 45 56 65 65 78 78 78 82 87 89 90]
It has returned an array sorted in ascending order.
Example Codes: numpy.sort()
to Sort a Multi-Dimensional Array
We will pass a multi-dimensional array now.
import numpy as np
a = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
sorted_array = np.sort(a)
print("The sorted array is:")
print(sorted_array)
Output:
The sorted array is:
[[ 5 11 12]
[ 6 10 15]
[ 8 10 12]
[ 8 12 15]
[34 78 90]]
The function has sorted the array in an ascending order i.e along the last axis as the default value for the axis = -1
.
Example Codes: numpy.sort()
to Sort a Multi-Dimensional Array Along a Specified Axis
We will set the value of the axis
parameter to None
.
import numpy as np
a = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8], [34, 78, 90]])
sorted_array = np.sort(a, axis=None)
print("The sorted array is:")
print(sorted_array)
Output:
The sorted array is:
[5 6 8 8 10 10 11 12 12 12 15 15 34 78 90]
Note that the function has converted the array to a one-dimensional array first and then it has sorted it.
Now, we will sort our array along the first axis.
import numpy as np
a = np.array([[11, 12, 5], [15, 6, 10], [10, 8, 12], [12, 15, 8]])
sorted_array = np.sort(a, axis=0)
print("The sorted array is:")
print(sorted_array)
Output:
The sorted array is:
[[10 6 5]
[11 8 8]
[12 12 10]
[15 15 12]]
The function has sorted the array along the first axis i.e in descending order.
Example Codes: numpy.sort()
to Sort Different Types of Arrays
We can use this function to sort arrays of different data types like an array of strings, a boolean array, etc.
import numpy as np
a = np.array([["z", "x"], ["b", "a"], ["g", "l"], ["k", "d"]])
sorted_array = np.sort(a)
print("The sorted array is:")
print(sorted_array)
Output:
The sorted array is:
[['x' 'z']
['a' 'b']
['g' 'l']
['d' 'k']]
Note that it has sorted the array in increasing alphabetical order. Now, we will pass an array of boolean values.
import numpy as np
a = np.array([[True, False, True], [False, False, True], [False, True, True]])
sorted_array = np.sort(a)
print("The sorted array is:")
print(sorted_array)
Output:
The sorted array is:
[[False True True]
[False False True]
[False True True]]