JavaScript String.toLowerCase() Method
-
Syntax of JavaScript
string.toLowerCase()
: -
Example Codes: Use the
string.toLowerCase()
Method to Change the Uppercase Letters of a String to Lowercase -
Example Codes: Use the
string.toLowerCase()
Method to Convert the Entire String to Lowercase Except for the First Letter
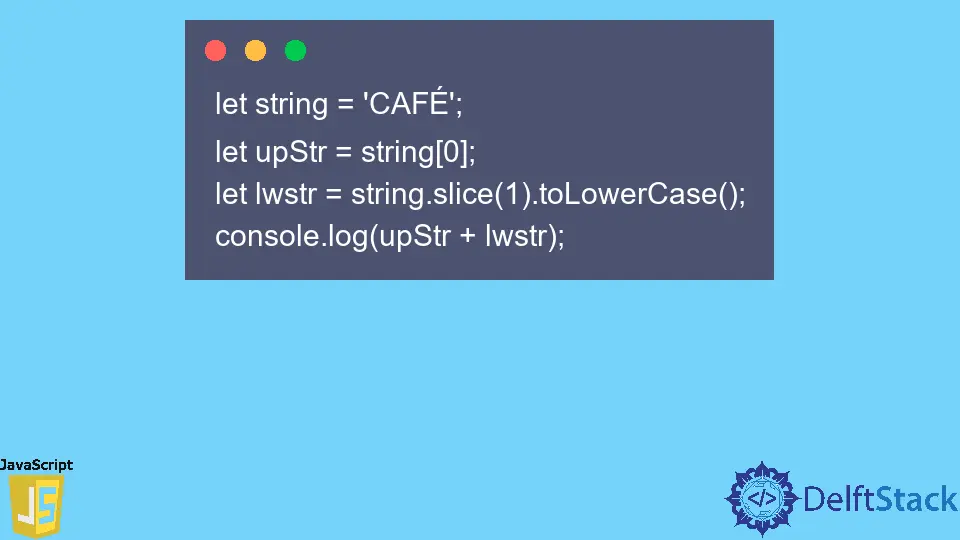
The string.toLowerCase()
method is used to change the uppercase letters of a string into lowercase while creating a new string. In JavaScript, toLowerCase()
is used with other methods, including string.slice()
, to change all the letters into lowercase except the first letter while creating a string.
Syntax of JavaScript string.toLowerCase()
:
refString.toLowerCase();
refString.slice(1).toLowerCase();
Parameter
This method does not take any parameters.
Return
It returns a new string after changing all the letters of a string to lowercase.
Example Codes: Use the string.toLowerCase()
Method to Change the Uppercase Letters of a String to Lowercase
The string.toLowerCase()
method converts all the letters of the given string into lowercase characters while creating a new string without changing the reference string in JavaScript.
In the example below, we have created a toggle case string. While using the toLowerCase()
method to convert all the letters to lowercase of a given string, we have not passed any parameter.
var string = 'Select UpperCase or LowerCase Letters.';
let lwStr = string.toLowerCase();
console.log(lwStr);
Output:
select uppercase or lowercase letters.
Example Codes: Use the string.toLowerCase()
Method to Convert the Entire String to Lowercase Except for the First Letter
Since the string.toLowerCase()
method can convert all the letters of a string into lowercase characters, we can use the string.slice()
method to exclude the first letter. In this example, we have got the first letter with string[0]
and the remaining letters using string.slice().toLowerCase
method.
let string = 'CAFÉ';
let upStr = string[0];
let lwstr = string.slice(1).toLowerCase();
console.log(upStr + lwstr);
Output:
Café
The string.toLowerCase()
method converts the entire string to lowercase. To convert the only substring of the referencestring
, users can use the string.slice()
method with the toLowerCase()
method in JavaScript.