JavaScript Math.pow() Method
-
Syntax of
Math.pow()
-
Example 1: Use the
Math.pow()
Method in JavaScript -
Example 2: Use the
Math.pow()
Method With Exception -
Example 3: Create Your Own
Math.pow()
Function
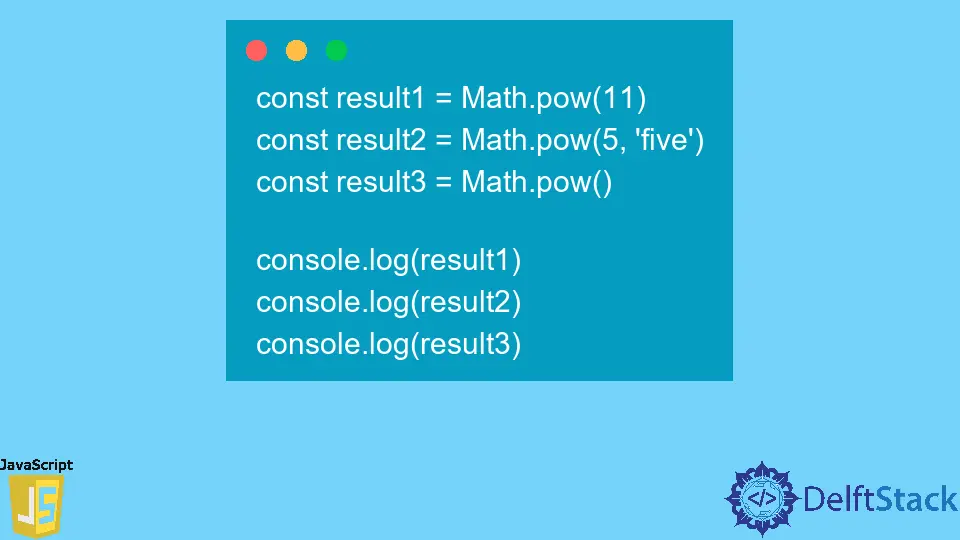
The Math.pow()
method only deals with the integers. It accepts base and exponent as two parameters and calculates the base’s power with the exponent.
Syntax of Math.pow()
Math.pow(base, exponent)
Parameters
base |
The parameter base refers to a numeric value. It is required. |
exponent |
The parameter exponent refers to a numeric value. It is required. |
Return
The Math.pow()
method returns the power of a number. This method returns NaN
if the base or exponent does not contain an integer value.
Example 1: Use the Math.pow()
Method in JavaScript
const result1 = Math.pow(2, 5)
const result2 = Math.pow(-5, 2)
console.log(result1)
console.log(result2)
Output:
32
25
First, we take 2
as the base and 5
as the exponent. This method returns 32
as a result.
Second, we calculate -5
to power 2
. It returns a positive number even though we are computing it with a negative number.
A simple mathematical reason is that a square always returns a positive number.
Example 2: Use the Math.pow()
Method With Exception
const result1 = Math.pow(11)
const result2 = Math.pow(5, 'five')
const result3 = Math.pow()
console.log(result1)
console.log(result2)
console.log(result3)
Output:
NaN
NaN
NaN
This method returns NaN
if one parameter is missing, or not a number provides, or no value is passed in the parameter.
Example 3: Create Your Own Math.pow()
Function
function myPowfunction (base, exponent){
return base ** exponent
}
console.log(myPowfunction(2,5))
console.log(myPowfunction(-5,2))
Output:
32
25
We have created our power function and named it myPowfunction
. Our created function behaves exactly like the Math.pow()
method.
The purpose is to realize that there is no rocket science behind this. One with basic JavaScript knowledge may be able to understand what’s happening behind the scene.
Niaz is a professional full-stack developer as well as a thinker, problem-solver, and writer. He loves to share his experience with his writings.
LinkedIn