JavaScript boolean.toString() Method
-
Syntax of JavaScript
boolean.toString()
Method -
Example 1: Use
boolean.toString()
to GetTrue
orFalse
Statement as a String -
Example 2: Use
boolean.toString()
to Use the Statement With Another String -
Example 3: Create Boolean Object With Integer Value and Use the
Boolean.toString()
Method - Example 4: Create a Boolean Object With Undefined Value
- Example 5: Create a Boolean Object With a Non-integer or Non-string Value
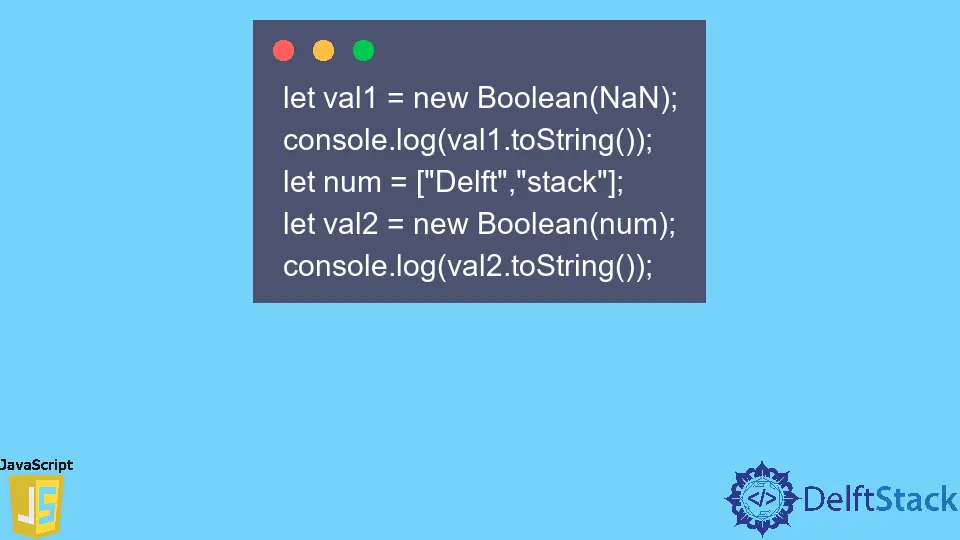
In JavaScript, the boolean.toString()
method allows us to use the Boolean value as HTML text after converting it into a string.
Syntax of JavaScript boolean.toString()
Method
boolean.toString();
Parameters
This method doesn’t contain any parameters.
Return
This method returns the string representation of the Boolean
object.
Example 1: Use boolean.toString()
to Get True
or False
Statement as a String
In JavaScript, the boolean.toString()
method converts the value into a string and allows us to use it as HTML text. In this example below, we have created a variable that got the text as a string using the boolean.toString()
method.
let boolean = true;
let text = boolean.toString();
console.log(text);
Output:
true
Example 2: Use boolean.toString()
to Use the Statement With Another String
In JavaScript, the boolean.toString()
method changes a true
or false
statement into a string that can be used with another string. In this below example, we have created a string and added the value for the output using the boolean.toString()
method.
let ques ="Question 1:";
let ans = true;
console.log(ques + ans.toString());
Output:
Question 1:true
Example 3: Create Boolean Object With Integer Value and Use the Boolean.toString()
Method
When we create the Boolean
object with integer values, it returns true
or false
according to the value of the integer. For 0, the Boolean
object returns false
and all other numeric values; it returns true
.
In this example below, we have created two different Boolean
objects and applied the Boolean.toString()
method, which returns a true
or false
string value.
let val1 = new Boolean(1);
console.log(val1.toString());
let val2 = new Boolean(0);
console.log(val2.toString());
Output:
true
false
Example 4: Create a Boolean Object With Undefined Value
When users create the Boolean
object with the undefined
value and use the toString()
method by taking that object as a reference, it always returns a false
string.
let val1 = new Boolean(undefined);
console.log(val1.toString());
let num;
let val2 = new Boolean(num);
console.log(val2.toString());
Output:
false
false
Example 5: Create a Boolean Object With a Non-integer or Non-string Value
When we create the Boolean
object using non-string values, it returns true
if the parameter element is not empty or contains some values and false
for undefined
, NaN
, and null
values.
In this example below, we created the array and the Boolean
object using it. Afterward, we used the toString()
method on the array, returning the true
string value.
let val1 = new Boolean(NaN);
console.log(val1.toString());
let num = ["Delft","stack"];
let val2 = new Boolean(num);
console.log(val2.toString());
Output:
false
true
The boolean.toString()
method is supported in all browsers of the current version.