JavaScript Array.reduce() Method
-
Syntax of JavaScript
array.reduce()
: -
Example Code: Use the
array.reduce()
Method to Get a Single Value After Executing a Reducer Function -
Example Code: Use the
array.reduce()
Method to Join the Elements and Get a Single Sentence -
Example Code: Use the
initialValue
Parameter With thearray.reduce()
Method -
Example Code: Possible
TypeError
When Using thearray.reduce()
Method
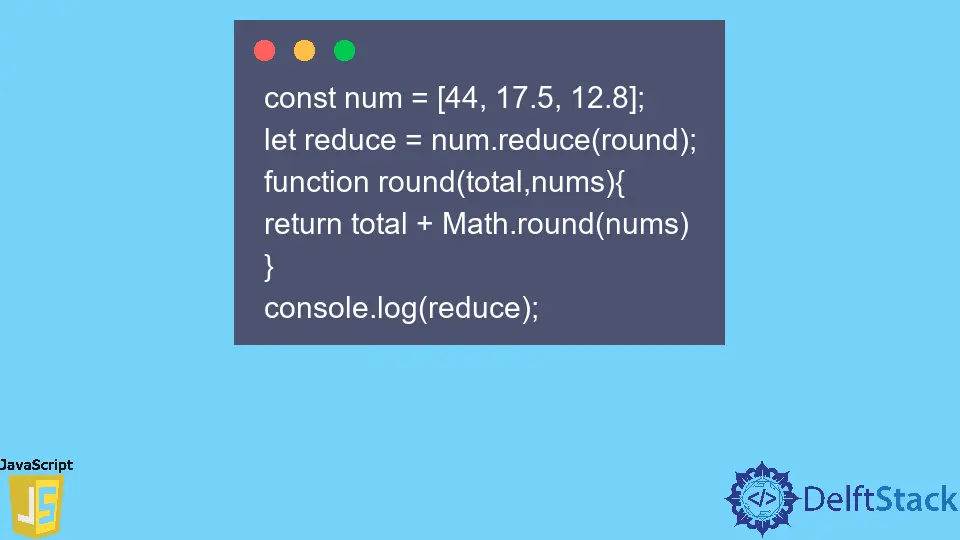
The array.reduce()
method is used to get a single value after running a reducer function for the array element. This method can also be used as a shorthand approach instead of creating a for
loop method.
Syntax of JavaScript array.reduce()
:
array.reduce(function(total,currentValue));
array.reduce(function(total,currentValue,currentIndex,arr){ /* ... */},initialValue);
Parameters
callback function() |
The function that will run for each element of an array to return a single value. |
total |
The initial value or the output previously returned by running the function. |
currentValue |
The current element’s value will be required to reduce and get a single value. |
currentIndex |
Index of the current element. |
arr |
Array of the elements. |
initialValue |
When callback function() is called for the first time, total will be initialized by the initial value. |
Return
This method returns a single value after running a specified function on the array.
Example Code: Use the array.reduce()
Method to Get a Single Value After Executing a Reducer Function
We can utilize the array.reduce()
method to get a single value after running a reducer function. In the example, we have created an array of different numbers.
In the array.reduce()
method, we have called a function and added total
and currentValue
as parameters. In the output, we got a single value using the array.reduce()
method.
const num = [44, 17.5, 12.8];
let reduce = num.reduce(round);
function round(total,nums){
return total + Math.round(nums)
}
console.log(reduce);
Output:
75
Example Code: Use the array.reduce()
Method to Join the Elements and Get a Single Sentence
In every array, different elements get separated by commas. We can employ the array.reduce()
method to join the elements and get a single sentence.
We have created an array with different words as elements in the example. We will use the array.reduce()
method to get a single sentence.
const sent = ["JavaScript", "is", "fun."];
let reduce = sent.reduce(single);
function single(total,currentValue,currentIndex,arr){
return total + " " + currentValue;}
console.log(reduce);
Output:
JavaScript is fun.
Example Code: Use the initialValue
Parameter With the array.reduce()
Method
In the example below, users can see that we have created the subtract
function, which subtracts all array elements one by one from the total
value. Also, we have used the initialValue
parameter of the array.reduce()
method.
When the subtract()
function is called for the first time, total
will be initialized with the initialValue
, and the array.reduce()
method will return a single element after subtracting all array elements from the total
value.
const num = [30, 70, 80, 90];
let initialValue = 500;
let reduce = num.reduce(subtract,initialValue);
function subtract(total,nums){
return total - nums;
}
console.log(reduce);
Output:
230
Example Code: Possible TypeError
When Using the array.reduce()
Method
While using the array.reduce()
method, an error occurs if the user’s array doesn’t contain any single element and doesn’t provide the initialValue
as a parameter.
In the example below, we have taken the empty array to reduce and haven’t passed the initialValue
parameter. So, an exception occurs, and it returns the TypeError
.
const values = [];
let updatedValue = values.reduce(subtract);
function subtract(total,nums){
return total - nums;
}
console.log(updatedValue);
Output:
TypeError: Reduce of empty array with no initial value
The array.reduce()
method is supported in all browsers of the current version. In the .reduce()
method, the total
parameter is considered the first element, and currentValue
is the other element of the given array.