JavaScript Array.from() Method
-
Syntax of JavaScript
Array.from()
: -
Example Code: Use the
Array.from()
Method to Create the Shallow Copy of the Array -
Example Code: Use the
Array.from()
Method to Create the New Array From Array-Like Object -
Example Code: Use the
Array.from()
Method to Create an Array of Characters From the String -
Example Code: Use the
Array.from()
Method WithMapFunction()
-
Example Code: Use the
Array.from()
Method WithMapFunction()
andthisarg
Parameter
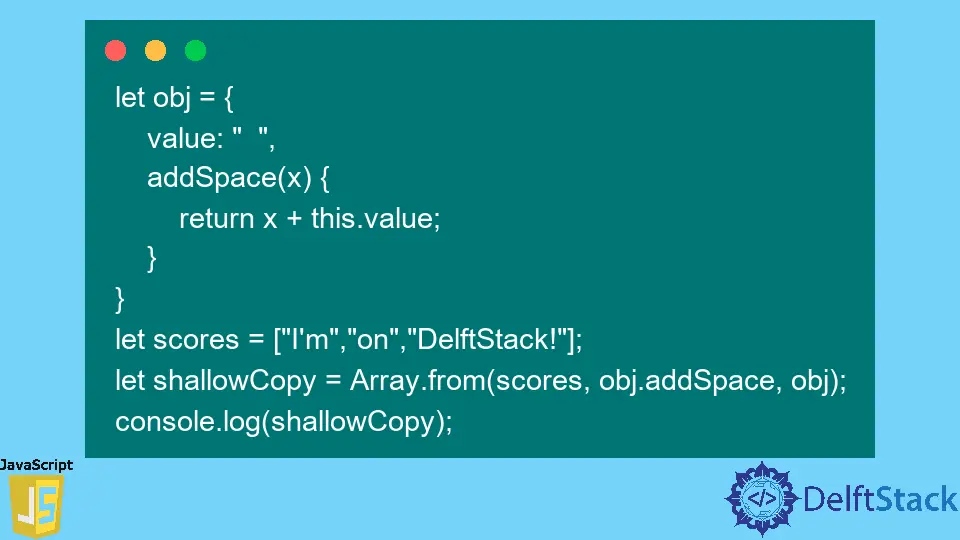
In JavaScript, we can use the array.from()
method to create the shallow-copy of the array from any other iterable or array-like object. To manually make the new array from the other array, we have to iterate through every element of the array and append it to the new array, but the array.from()
method does that with a single line of code.
Syntax of JavaScript Array.from()
:
Array.from(iterableObject);
Array.from(iterableObject,mapFunction);
Array.from(iterableObject,mapFunction,thisArg);
Array.from(iterableObject,function mapFunction(ele,index){/* function body */},thisArg);
Parameters
iterableObject |
This is the iterable or array-like object to create a new array. |
mapFunction() |
This is the function to call every element on the array and return the resultant element for a new array related to the current element. |
thisArg |
This refers to the value to use as this while executing the mapFunction() . |
ele |
This is the current element of the array. |
index |
This is the current element’s index of the array. |
Return
The method returns the shallow copy of the iterableObject
after invoking the mapFunction()
on every element.
Example Code: Use the Array.from()
Method to Create the Shallow Copy of the Array
In the following example, we have created the arr
of strings and numbers. After that, we used the Array.from()
method to create the newArray
from the arr
.
In the output, you can see that arr
and newArray
is the same.
let arr = [70,50,"Delft","stack"];
let newArray = Array.from(arr);
console.log(arr);
console.log(newArray);
Output:
[ 70, 50, 'Delft', 'stack' ]
[ 70, 50, 'Delft', 'stack' ]
Example Code: Use the Array.from()
Method to Create the New Array From Array-Like Object
The array-like object contains the key-value pairs and the object’s length, which has the total key-value pairs in the object. We have created the new array from the arr_like
using the Array.from
method.
const arr_like = {0: 'Welcome', 1: 'to', 2: 'DelftStack', length: 3};
let shallowCopy = Array.from(arr_like);
console.log(shallowCopy);
Output:
[ 'Welcome', 'to', 'DelftStack' ]
Example Code: Use the Array.from()
Method to Create an Array of Characters From the String
In this example, we have created two different strings. We have applied the Array.from()
method to generate the new array of characters from the string.
const string = "DelftStack";
const string1 = "Hello World!"
let shallowCopy = Array.from(string);
console.log(shallowCopy);
console.log(Array.from(string1));
Output:
[ 'D', 'e', 'l', 'f', 't', 'S', 't', 'a', 'c', 'k' ]
[ 'H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd', '!' ]
Example Code: Use the Array.from()
Method With MapFunction()
We have added the mapFuncton()
to the Array.from()
method as a parameter. The MapFunction()
adds the index
to every array element and returns the new element for the new array.
const refArray = [40, 50, 20]
let shallowCopy = Array.from(refArray, function mapFunction(ele,index){
return ele + index
});
console.log(shallowCopy);
Output:
[ 40, 51, 22 ]
Example Code: Use the Array.from()
Method With MapFunction()
and thisarg
Parameter
Here, we have created the obj
object, which contains some variables and methods. In this example, we are using the addSpace()
method as a mapFunction()
and obj
as a this argument.
Now, whenever a user uses the this
keyword inside the addSpace()
function, it will refer to the obj
object.
We are adding the two spaces to every element of the string array in this example using the thisarg
and mapFunction()
parameters.
let obj = {
value: " ",
addSpace(x) {
return x + this.value;
}
}
let scores = ["I'm","on","DelftStack!"];
let shallowCopy = Array.from(scores, obj.addSpace, obj);
console.log(shallowCopy);
Output:
[ 'I\'m ', 'on ', 'DelftStack! ' ]