JavaScript Array Every() Method
-
Syntax of JavaScript
array.every()
: -
Example Codes: Use the
array.every()
Method to Check a Condition for Each Element in an Array -
Example Codes: Use the
array.every()
Method to Check a Condition for Each Answer in an Array
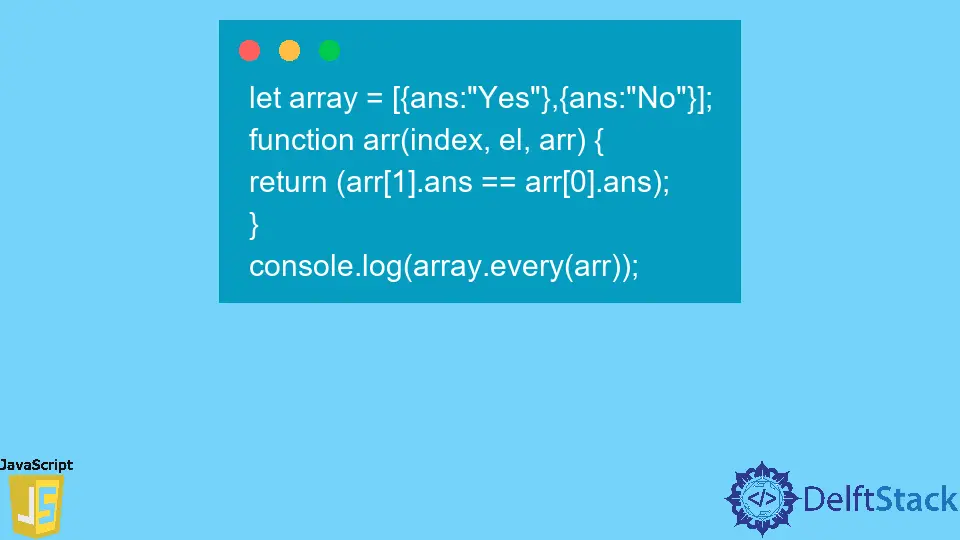
The array.every()
method is used in conjunction with a function in JavaScript. This method checks whether all the elements in the provided array match the condition mentioned in the callback function, which we pass as a parameter.
If the condition is true for all the elements within the array, then the array.every()
method will return true
.
Syntax of JavaScript array.every()
:
refarray.every(function(currentValue));
refarray.every(function(currentValue, index, arr));
Parameters
callback function() |
A function used to check the condition for each element in the given array. |
currentValue |
To use the element’s value and check the condition mentioned in the callback function() . |
index |
To check the condition using the index of the elements. |
arr |
To use the array of the element and check the condition for every element in the array. |
Return
This method returns a boolean value depending on whether each element fulfills the condition mentioned in the callback function()
parameter.
Example Codes: Use the array.every()
Method to Check a Condition for Each Element in an Array
While creating an array, there might be a condition that each element must fulfill. By using the array.every()
method, you can check that each element passed the condition if the method returns true
.
In this example, we have created an array. To check the condition, we have passed a callback function()
in the array.every()
method.
const nums = [32, 16, 40];
function check(num){return num > 15;}
console.log(nums.every(check));
Output:
true
Example Codes: Use the array.every()
Method to Check a Condition for Each Answer in an Array
An array can contain objects, and objects can have different key-value pairs such as number, string, and boolean values. While checking a condition for every answer within an array, we can use the array.every()
method with the callback function.
In the following example, we have passed some parameters to check whether a condition is true for a given array.
let array = [{ans:"Yes"},{ans:"No"}];
function arr(index, el, arr) {
return (arr[1].ans == arr[0].ans);
}
console.log(array.every(arr));
Output:
false
Array.every()
method is commonly used to check various elements’ conditions. Users can add different statements within the function to ensure that each element meets all the mentioned conditions.