JavaScript array.constructor Property
-
Syntax of
array.constructor
Property in JavaScript -
Example 1: Return Function That Created the Array Using
array.constructor
-
Example 2: Use
array.constructor
to See if Two Objects Are of the Same Type -
Example 3: Use the
array.constructor
Property to Retrieve the Type of anArray
Object -
Example 4: Use
array.constructor
Property Withname
Property to Retrieve the Class Name of an Array Object
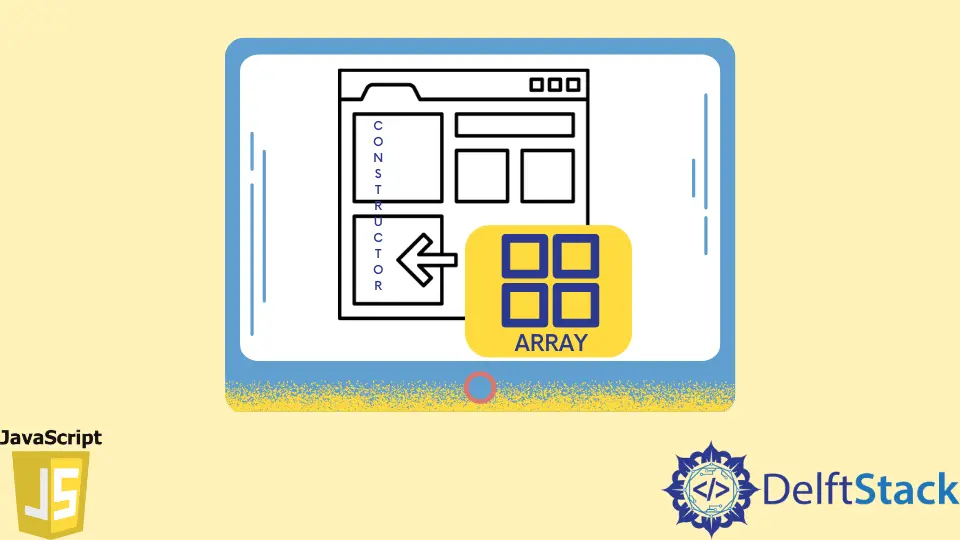
The Array
object has an array.constructor
property. The array.constructor
property gives back an array’s constructor
function that created the array
object.
The array.constructor
property inherits from the prototype of the array
object.
Syntax of array.constructor
Property in JavaScript
constructor = arrayObj.constructor
Parameters
The JavaScript array.constructor
does not take any parameter because it is not a function but a property.
Return
The array.constructor
property returns the reference to the function which created the array
object.
Example 1: Return Function That Created the Array Using array.constructor
Except for undefined
and null
, all values in JavaScript have a constructor
property. This property gives the constructor
function of an object.
Below is an example of the array.constructor
property used to get an array-type object’s constructor
function.
let alphabets = ["a", "b", "c"];
let return_function = alphabets.constructor;
console.log('return function: ', return_function)
The class of the object is returned by the array function Object() { [native code] }
in JavaScript. Because the object used in this case is of type Array
.
The constructor
property returns the function that formed the object.
Output:
return function: function Array() { [native code] }
Example 2: Use array.constructor
to See if Two Objects Are of the Same Type
var arr1 = new String("delf");
var arr2 = new String("stack");
console.log(arr1.constructor === arr2.constructor)
Above, if both objects have the same type, the comparison will return true
.
Output:
true
Here, it returned true
as both objects arr1
and arr2
were of array type.
Example 3: Use the array.constructor
Property to Retrieve the Type of an Array
Object
Below is an example showing the alternative to the typeof
method in JavaScript. This approach can reduce the number of if-else
statements if the type of the objects needs to be categorized.
Here, we will only classify an object to see if it is an array-type object or something else.
myObject = ['d', 'e', 'l', 'f', 't'] //Array
if (myObject.constructor === Array) {
// code executed when myVariable is a number
console.log(`the ${myObject} type is not an array.`);
} else {
console.log(`the ${myObject} type is not an array.`)
}
Output:
the d,e,l,f,t type is not an array.
Now, if myObject
changed to a string type, it gives a different output.
myObject = 'sdf' //string
if (myObject.constructor === Array) {
// code executed when myVariable is a number
console.log(`the ${myObject} type is not an array.`);
} else {
console.log(`the ${myObject} type is not an array.`)
}
Output:
the sdf type is not an array.
Example 4: Use array.constructor
Property With name
Property to Retrieve the Class Name of an Array Object
The array.constructor
property also has a property of name
, if used after the constructor
property of the object, tells the name of the class. The below example tells the class of the array-type object.
myObject = ['d', 'e', 'l', 'f', 't']
console.log(myObject.constructor.name)
Output:
Array