How to Declare and Use Variables in SQLite
- Variables in SQLite
- Types of Variables in SQLite
- Declare Variables in SQLite
- Use the Declared Variables in SQLite
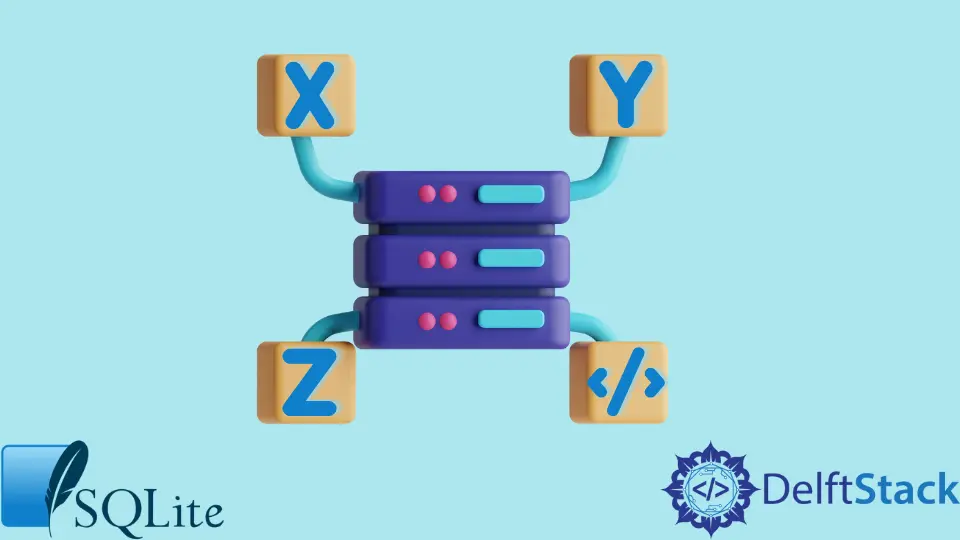
Creating and using functions can prove to be incredibly beneficial when you’re constructing queries on SQLite. A part of these functions and procedures is using variables within SQLite.
Variables in SQLite
Variables can prove to be incredibly beneficial if used correctly. It is best to explain to them while using an example.
Suppose you create a procedure or function to add two numbers. This can easily be achieved by using the right operator.
However, if we were to make it a little more complex and say that you would get both of these numbers as input from the user, it won’t be as simple.
You can’t hardcode the values because the user can input any number. This is when a variable comes in handy.
It will take the input the user gave and store it into a variable that will stay until it is overwritten or the program terminates. Now, instead of adding two random values, you can add the variables that hold the two values selected by the user.
This can be stored in a third variable or of the existing two if they are not to be used later on. With variables, you can easily store and manipulate any value of multiple data types within your procedure.
This makes them crucial to any function, as hardcoded values are impossible to rely on.
Types of Variables in SQLite
There are multiple types of variables in SQLite. This means they have different data types to tell the system what data will be stored in that variable.
Here are some of the data types available in SQLite:
NULL
: This type adds a null value to the variable.INTEGER
: This is for signed integers. All positive and negative numbers are in 0, 1, 2, 3, 4, 6, or 8 bytes.REAL
: This is for a floating point variable which means a positive or negative number with a decimal point. This is stored as an 8-byte number.TEXT
: As the name suggests,TEXT
will include a text string. This is often stored using a database encoding such as UTF-8, UTF-16BE, or UTF-16LE.BLOB
: This is a special variable in which data is stored the same way it was input, adapting to whatever data type was used.
It is important to note that if you ever used an incorrect data type or wish to change it somewhere in the middle of the code, you can always change it using the CAST
expression. This will alter the data type and contents, so use it carefully; you might end up with corresponding ASCII values where you don’t need them.
Declare Variables in SQLite
To be able to use variables in SQLite, you will need to declare them first. The declaration process is fairly simple, depending on the type of variable that you’re trying to create; however, it is slightly different than the native syntax.
In SQLite, you will have to go through the process virtually using an in-memory temp table. Here is an example of how it can be done:
BEGIN;
/* This tells your system to use in-memory */
PRAGMA temp_store = 2;
/* creating the temp table with the variables you need */
CREATE TEMP TABLE Vars(text_val TEXT PRIMARY KEY, real_val REAL, integer_val INTEGER, blob_value BLOB, text_val TEXT);
/* The declaration part */
INSERT INTO Vars (text_val)
VALUES ('Any value');
DROP TABLE Vars;
END;
By using the code above, you can work your way around declaring a variable indirectly. Here, you must create an in-memory temp table first; using this, you can add columns with your preferred data types and then use the INSERT
clause to store values in them.
The BEGIN
and END
clauses help to identify where the procedure begins and ends, so they must be included while using this method. After this, you can manipulate and use the data however you prefer.
This has been explained in greater detail below.
Use the Declared Variables in SQLite
You now know how to declare variables, but learning how to use them is just as important. Without this, you won’t be able to get any use out of the variables you have declared.
Let’s do so by using an updated version of the same code used above.
BEGIN;
/* Add codes from above to use in memory,
create the temp table,
and declaration first*/
/* Assignment (select a storage class according to your requirements) */
UPDATE Vars SET integer_val= ...
WHERE text_val= 'Any value';
/* Get the value of the variable that is to be used in the expression) */
...
(SELECT coalesce(real_val, integer_val, blob_value, text_val)
FROM Vars
WHERE text_val= 'Any value' LIMIT 1) ...
DROP TABLE Vars;
END;
This code has a few additions allowing you to allocate variables’ values. First, you must assign values to your variables and then link them back to use them in an expression.
Finally, the table is dropped once the function is complete. You can always add additional lines of code to allow variables to behave according to your requirements.
However, dropping the table removes all the stored data, so be sure to do so once you’re done using everything.
Another method is the usage of the WITH
clause:
...
WITH const AS (SELECT 'any value' AS text_val, 10 AS int_val)
SELECT table.cost, (table.cost + const.int_val) AS cost2
FROM table, const
WHERE table.text_val= const.text_val
Here, two variables are added and stored in one of them.
Note: You could always replace this with the union of a series of classes after they are inserted into a table. The logic may vary, but the concept remains the same.
That was everything you need to know about variables in SQLite. Now, you can easily create and use them according to your preferences.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub