How to Use the Switch Statement in a React Component
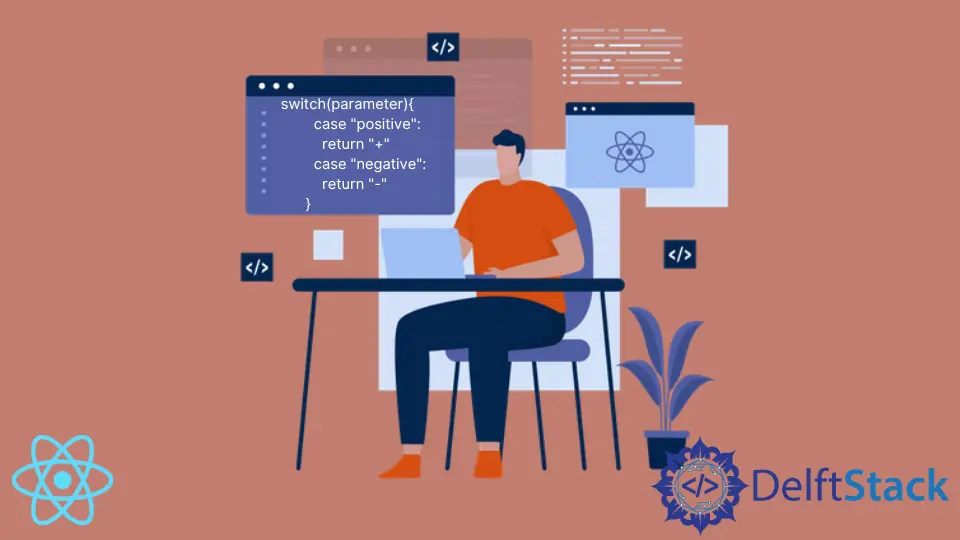
The switch
statement is one of the most useful features of JavaScript.
It is perfect for setting up conditions and returning a specific value depending on whether or not those conditions are met. In React, a switch
statement is one of the best ways to handle conditional rendering.
For instance, you might want to render a specific component based on users’ inputs. You can store the value of input
fields in the state and examine the state value to determine the right component to render.
Most React developers use JSX to define components and invoke them. At first glance, JSX looks a lot like HTML, so it’s easy to read and make changes whenever necessary.
It also allows us to use JavaScript expressions within the JSX code. However, its support is limited to JavaScript expressions and does not extend to statements like for
or switch
.
Read this guide to learn more about the differences between statements and expressions.
This article will discuss multiple ways to use switch()
(or its valid replacements) within JSX.
How to Use switch
in React (JSX)
JSX is a React syntax that allows us to create components and UI elements without using the .createElement()
function. It also has many additional features that HTML doesn’t have.
For instance, you can write regular JavaScript code within JSX. All you must do is put the expressions between curly braces.
Let’s look at an example:
class App extends Component {
render() {
return <h1>Two plus two is {2+2}</h1>;
}
}
Here we have a component that returns a single heading element.
As you can see in the playcode, the 2+2
is treated as a valid JavaScript expression because it is placed between two curly braces. This is a simple demonstration of a compelling feature.
When developing React applications, it’s a good idea to avoid putting too much logic in your JSX structure. This is necessary to avoid cluttering your code.
Instead, you can define a function or variable outside the return
statement and reference it in your JSX code.
Developers can not write switch
statements directly in the JSX. Firstly, it would be too lengthy, and more importantly, statements are not supported inside JSX.
switch
Outside of the return
Statement
Probably the most optimal solution is to take switch()
out of your return
statement entirely. Then you can use standard JavaScript without any restrictions and reference the function that includes the switch
statement.
Let’s look at an example:
class App extends Component {
render() {
const functionWithSwitch = (parameter) => {
switch(parameter){
case "positive":
return "+"
case "negative":
return "-"
default:
return "neutral"
}
}
return <h1>{functionWithSwitch("positive")}</h1>;
}
}
In this example, we have a simple functionWithSwitch()
function that includes the switch
statement and returns three different values based on the parameter value. Instead of defining all of this within JSX, we do it separately, reference the function, and pass it to the desired value.
Inline switch
We previously mentioned that it’s impossible to use switch
directly within JSX, but there are ways to work around that rule.
For some people, inline solutions are easier to read and understand what’s going on. You don’t have to move your attention back and forth to the function definition and call to figure out what’s going on.
Technically, this solution is not a switch, but it performs exactly the same way. Let’s look at an example:
class App extends Component {
render() {
return <h1>
{
{
'positive': '+',
'negative': '-'
}[param]
}
</h1>;
}
}
Here we utilize a plain JavaScript object to render a string conditionally. In this case, it has two properties with two values. Ultimately, you’ll get a value of whichever property you reference as a param
.
To set up a default case with this approach, you need to use the logical OR
(||
) operator.
Self-Invoking Function
When necessary, you can also put all of the switch
-related code in a function and automatically invoke it within JSX. This is a slightly lengthy solution, but it might be necessary on some occasions.
Let’s look at an example:
class App extends Component {
render() {
return <h1>{( () => {
switch(parameter){
case "positive":
return "+"
case "negative":
return "-"
default:
return "neutral"
}
})()}</h1>;
}
}
We took the previous function example and immediately invoked it to return a value. Again, there are better ways to use switch
statements within JSX, but this is still a good solution.
Custom Element Switch
The popularity of React can be largely attributed to its reusable components.
Creating a custom Switch
component will save you the trouble of writing complex inline logic. Also, you won’t have to jump back and forth to understand what a function does.
The custom <Switch />
component will take a prop, and depending on its value; it will render one of the children components placed between the opening and closing tags of the Switch
component.
Let’s look at an example:
let SwitchComponent = props => {
const {condition, childrenElements} = props
return childrenElements.find(element => {
return element.props.value === condition
})
}
We can invoke a <Switch>
component and add children with custom values in the parent component. This is similar to how you’d write different cases
in the switch
statement.
Let’s look at one example, based on the switch
statement we wrote before:
<Switch test="positive">
<p value={"positive"}>+</p>
<p value={"negative"}>-</p>
</Switch>
In this case, because of the value of the test
prop, the Switch
component will only render the paragraph element with a positive
value.
Alternatives to switch()
in React
React developers often use a ternary operator for simple conditional logic, which takes a condition and returns either one of the components based on the condition. The ternary operator can be written directly between curly braces inside JSX; it is simple, easily readable, and useful.
Let’s look at our example and write the same condition using the ternary operator:
<h1>{condition === "positive" ? "+" : "-"}</h1>
This is a relatively simple solution. Of course, it has its limitations and wouldn’t work with more complex logic.
Summary
This article described many different ways to render a component conditionally. All of these solutions are valid options, and your choice should ultimately depend on your own or your team’s preferences.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn