How to Implement Scrollbar Feature in React
- Understanding Scrollbars in React
- Method 1: Using CSS for Custom Scrollbars
- Method 2: Using React Scrollbars Library
- Method 3: Implementing a Custom Scrollbar with React Hooks
- Conclusion
- FAQ
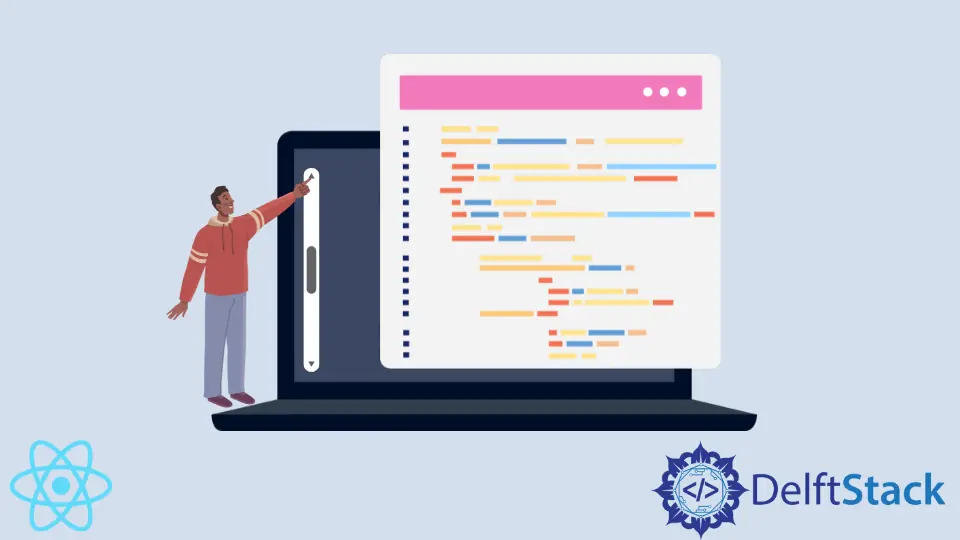
React is a powerful JavaScript library for building user interfaces, and one of the common UI components that developers often need to implement is a scrollbar. Scrollbars are essential for enhancing user experience, especially when dealing with lengthy content or data. This guide will show you how to implement a scrollbar feature for individual components in React. Whether you’re looking to create a custom scrollbar or simply want to style the existing one, we’ve got you covered. You’ll learn various methods to achieve this, complete with code examples and explanations. Let’s dive in!
Understanding Scrollbars in React
Before we jump into the implementation, it’s important to understand what a scrollbar is and why it’s relevant in React applications. A scrollbar allows users to navigate through content that exceeds the viewable area of a component. In React, you can control scrollbars using CSS and JavaScript, which makes it easy to customize their appearance and behavior.
React provides a way to manage state and props, making it easier to create dynamic and responsive scrollbars. By leveraging these features, you can create scrollbars that not only look good but also enhance usability.
Method 1: Using CSS for Custom Scrollbars
One of the simplest ways to implement a scrollbar in React is by using CSS. This method allows you to customize the appearance of the scrollbar without any additional libraries. Here’s how you can do it.
.custom-scrollbar {
width: 300px;
height: 200px;
overflow-y: scroll;
scrollbar-width: thin;
scrollbar-color: #888 #f1f1f1;
}
.custom-scrollbar::-webkit-scrollbar {
width: 8px;
}
.custom-scrollbar::-webkit-scrollbar-thumb {
background: #888;
border-radius: 10px;
}
.custom-scrollbar::-webkit-scrollbar-track {
background: #f1f1f1;
}
In this CSS code, we define a class called .custom-scrollbar
. The overflow-y: scroll;
property ensures that a vertical scrollbar appears when the content exceeds the height of the box. The scrollbar-width
and scrollbar-color
properties are used to customize the scrollbar for Firefox. The ::-webkit-scrollbar
pseudo-elements allow us to style the scrollbar for WebKit browsers like Chrome and Safari.
To use this class in a React component, simply apply it to a div:
import React from 'react';
import './styles.css';
const ScrollableComponent = () => {
return (
<div className="custom-scrollbar">
{/* Your content goes here */}
</div>
);
};
export default ScrollableComponent;
This approach is straightforward and effective for simple scrollbar customization. You can adjust the colors and dimensions as needed to match your application’s design.
Output:
A scrollable component with a customized scrollbar.
Using CSS for scrollbars is a quick solution, but it has its limitations. It may not provide the level of control you need for more complex applications. However, for many use cases, this method is sufficient and easy to implement.
Method 2: Using React Scrollbars Library
If you need more advanced features for your scrollbar, consider using a library like react-scrollbars
. This library provides a customizable scrollbar component that integrates seamlessly with React applications. To get started, you first need to install the library.
npm install react-scrollbars
Once installed, you can use it in your component as follows:
import React from 'react';
import { Scrollbars } from 'react-scrollbars';
const ScrollableComponent = () => {
return (
<Scrollbars style={{ width: 300, height: 200 }}>
{/* Your content goes here */}
</Scrollbars>
);
};
export default ScrollableComponent;
In this code, we import the Scrollbars
component from the react-scrollbars
library. We then wrap our content inside the Scrollbars
component and provide it with a width and height. The library takes care of rendering the scrollbar for us, allowing for easy customization.
You can also customize the scrollbar further by passing additional props. For example, you can change the style of the scrollbar using the thumbStyle
and trackStyle
props.
Output:
A scrollable component with an advanced scrollbar.
Using a library like react-scrollbars
offers several advantages, including better performance and more customization options. It’s an excellent choice for applications that require complex scrolling behavior or a polished look.
Method 3: Implementing a Custom Scrollbar with React Hooks
If you want complete control over the scrollbar behavior, you can implement a custom scrollbar using React hooks. This method gives you the flexibility to create a scrollbar that behaves exactly as you want. Here’s how you can do it.
First, we need to set up a component that tracks the scroll position. We’ll use the useRef
and useEffect
hooks for this purpose:
import React, { useRef, useEffect, useState } from 'react';
const CustomScrollbar = () => {
const contentRef = useRef(null);
const [scrollHeight, setScrollHeight] = useState(0);
const [scrollTop, setScrollTop] = useState(0);
useEffect(() => {
const handleScroll = () => {
const { scrollHeight, clientHeight, scrollTop } = contentRef.current;
setScrollHeight(scrollHeight - clientHeight);
setScrollTop(scrollTop);
};
const element = contentRef.current;
element.addEventListener('scroll', handleScroll);
return () => {
element.removeEventListener('scroll', handleScroll);
};
}, []);
return (
<div style={{ position: 'relative', width: 300, height: 200, overflowY: 'scroll' }} ref={contentRef}>
{/* Your content goes here */}
<div style={{ height: '600px' }}>Long content here...</div>
<div
style={{
position: 'absolute',
right: 0,
top: `${(scrollTop / scrollHeight) * 100}%`,
width: '8px',
height: '50px',
backgroundColor: '#888',
}}
/>
</div>
);
};
export default CustomScrollbar;
In this example, we create a custom scrollbar that tracks the scroll position of the content. The useRef
hook allows us to reference the scrolling element, while useEffect
sets up an event listener for the scroll event. The scrollbar’s position is calculated based on the current scroll position and the total scroll height.
Output:
A custom scrollbar that tracks the scroll position.
This method is more complex but gives you complete control over the scrollbar’s behavior and appearance. It’s especially useful for applications that require unique scrolling features or interactions.
Conclusion
Implementing a scrollbar feature in React can significantly enhance your application’s usability. Whether you choose to use simple CSS, a third-party library, or create a custom solution with React hooks, each method has its own advantages. The right choice depends on your specific needs and the complexity of your application. By following the methods outlined in this guide, you can create scrollbars that not only look good but also improve the overall user experience.
FAQ
-
What is the easiest way to create a scrollbar in React?
Using CSS is the easiest way to create a scrollbar in React. You can customize it with simple styles. -
Can I use third-party libraries for scrollbars in React?
Yes, libraries like react-scrollbars provide advanced features and customization options for scrollbars in React applications. -
How do I create a custom scrollbar using React hooks?
You can use the useRef and useEffect hooks to track the scroll position and render a custom scrollbar based on the content’s scroll height. -
Are there performance considerations when using custom scrollbars?
Yes, custom scrollbars can impact performance, especially if they involve complex calculations or render updates. It’s important to optimize your implementation. -
Can I style the scrollbar for different browsers?
Yes, you can use CSS properties specific to WebKit browsers and Firefox to style scrollbars differently across various platforms.
Irakli is a writer who loves computers and helping people solve their technical problems. He lives in Georgia and enjoys spending time with animals.
LinkedIn