How to Set Image Width in Percent in React Native
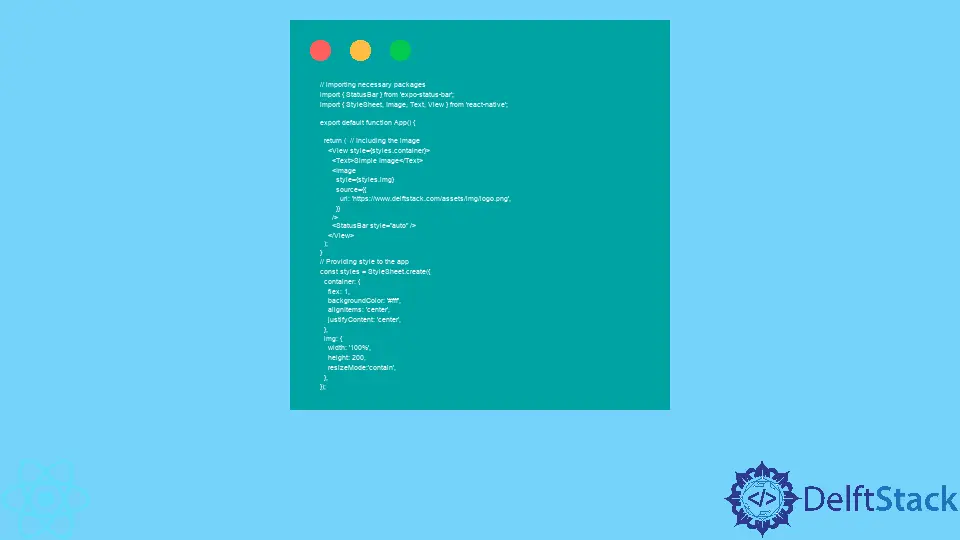
This article will show how we can set the width in percentage format in a React-Native app. Also, we’ll discuss the topic using necessary examples and explanations to make the topic easier.
Set Image Width in Percent in React Native
Not all device contains the same size. In a responsive design, it is necessary that an element change its size based on the device screen size.
The solution to this problem is to define the size of the element in a percentage format. In this way, the size of the element will automatically change based on the device screen size.
Our example below demonstrates how we can provide the width value in a percentage format. The code snippet for our example will be as follows.
// importing necessary packages
import {StatusBar} from 'expo-status-bar';
import {Image, StyleSheet, Text, View} from 'react-native';
export default function App() {
return ( // Including the image
<View style={styles.container}>
<Text>Simple Image</Text>
<Image
style={styles.img}
source={{
uri: 'https://www.delftstack.com/assets/img/logo.png',
}
}
/>
<StatusBar style="auto" / > <
/View>
);
}
/ / Providing style to the app
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center',
},
img: {
width: '100%',
height: 200,
resizeMode: 'contain',
},
});
We already commanded the purpose of all necessary lines regarding the steps shared above in the example.
<Image style={styles.img} source={{ uri: 'https://www.delftstack.com/assets/img/logo.png', }} />
After including the above code line image, we set the properties below on the style sheet.
For the containers
:
Properties | Definition |
---|---|
flex: 1 |
Define the container as flex. |
backgroundColor: '#fff' |
Set the background color two white. |
alignItems: 'center' |
Set horizontal align to center. |
justifyContent: 'center' |
Set vertical-align to center. |
For the image
:
Properties | Definition |
---|---|
width: '100%' |
Set the image width to 100%. |
height: 200 |
Set the image height to 200. |
resizeMode:'contain' |
Don’t crop the image. |
When you run the above example code, you’ll get the output below.
Output:
Note that the code shared above is created in React-Native, and we used the Expo-CLI
to run the app. Also, you need the latest version of Node.js.
If you don’t have Expo-CLI
in your environment, install it first.
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedInRelated Article - React Native
- How to Align Text Vertically in React-Native
- How to Set Font Weight in React Native
- How to Align Text in React-Native
- How to Animate SVG in React Native
- How to Set the Border Color in React-Native
- React Native Foreach Loop