Global Variable in React
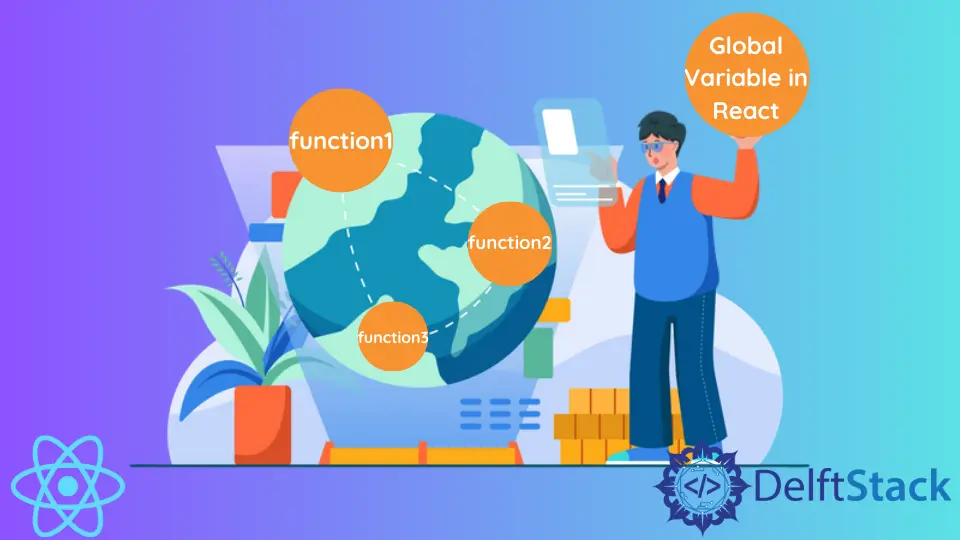
We will introduce how to create and use a global variable in React applications.
Create a Global Variable to Use in Multiple Functions in React
When multiple functions need to access the data or write to an object, global variables are used.
With management systems, we need access to certain data in multiple functions to create a global variable that can be accessed in all functions.
This tutorial will explain creating a global variable in React and using it in multiple functions or files.
Let’s create a React application using the command below.
# react
npx create-react-app my-app
After creating our new application in React, we will go to our application directory using this command.
# react
cd my-app
Now, let’s run our app to check if all dependencies are installed correctly.
# react
npm start
We will create a new file, GlobalVar.js
, to define a constant globalVar
and assign it a value. After this, we will export the variable.
So our code in GlobalVar.js
will look like below.
# react
const globeVar = "I am a Global Variable";
export default globeVar;
Now that we have exported our variable, it will work as a global variable. We can import this file into any file and use it as a global variable.
So, let’s import this file in App.js
and use the global variable.
# react
import "./styles.css";
import globeVar from "./GlobalVar";
export default function App() {
return (
<div className="App">
<h2>{globeVar}</h2>
</div>
);
}
Output:
Now, let’s use this global variable in multiple functions to check if we can easily access it or not.
We will create a new function changeValue()
in App.js
, where we will create a new variable newVar
and concatenate the globeVar
in it with a new string.
After that, we will console.log
the newVar
.
# react
function changeValue() {
this.newVar = globeVar + " with Changed Value";
console.log(this.newVar);
}
Once we have created a function, we will return a button with the onClick
method. So our code in App.js
will look like below.
# react
import "./styles.css";
import globeVar from "./GlobalVar";
export default function App() {
function changeValue() {
this.newVar = globeVar + " with Changed Value";
console.log(this.newVar);
}
return (
<div className="App">
<h2>{globeVar}</h2>
<button onClick={changeValue}>Button</button>
</div>
);
}
Output:
In this way, we can declare a global variable and use it to display or use it in a function or multiple functions.
You can check the full code here.
Rana is a computer science graduate passionate about helping people to build and diagnose scalable web application problems and problems developers face across the full-stack.
LinkedIn