The super Function in Python
- Understanding the Purpose of super()
- Method 1: Basic Usage of super()
- Method 2: Using super() with Multiple Inheritance
- Method 3: super() in Class Methods
- Conclusion
- FAQ
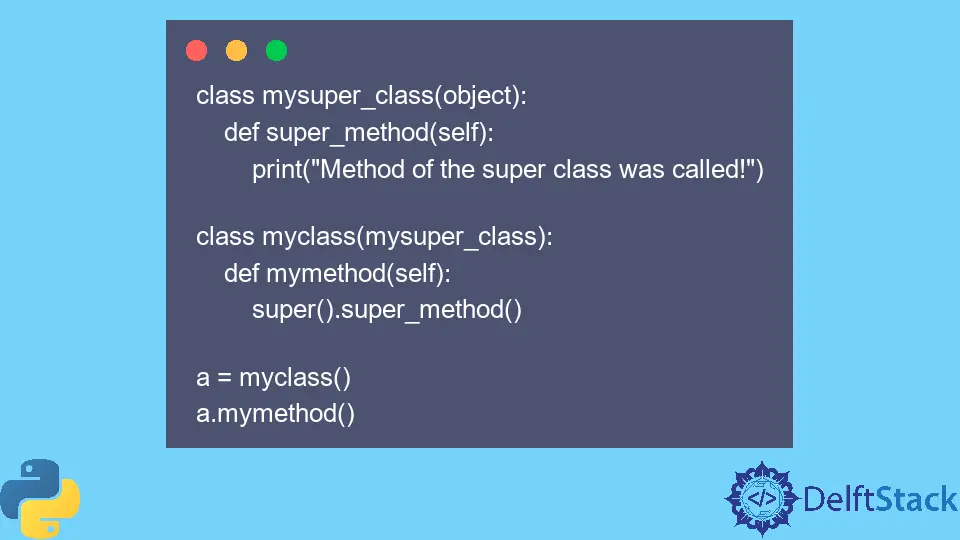
In Python, the super()
function is a powerful tool that allows you to call methods from a parent class in a child class. This built-in function is particularly useful in situations involving inheritance, where you want to extend the functionality of a base class without completely overriding its behavior. Understanding how to effectively use super()
can significantly improve your object-oriented programming skills in Python.
In this tutorial, we will delve into the purpose and usage of the super()
function, providing clear examples and explanations to help you grasp its importance in your coding journey.
Understanding the Purpose of super()
The primary purpose of the super()
function is to provide a way to access methods from a parent class. When working with inheritance, you may want to leverage the functionality of a base class while also adding or modifying behaviors in a derived class. This is where super()
shines. It allows you to call the parent class’s methods without needing to explicitly reference the parent class’s name, making your code more maintainable and easier to read.
Using super()
can also help avoid issues related to multiple inheritance, where a class inherits from more than one parent class. By using super()
, Python ensures that the method resolution order (MRO) is respected, allowing for a more predictable and manageable inheritance structure.
Let’s explore how to use the super()
function in various scenarios.
Method 1: Basic Usage of super()
To illustrate the basic usage of super()
, let’s consider a simple example involving two classes: a Vehicle
class and a Car
class that inherits from it. We will use super()
to call a method from the Vehicle
class within the Car
class.
class Vehicle:
def __init__(self, brand):
self.brand = brand
def display_info(self):
return f"This is a vehicle of brand {self.brand}."
class Car(Vehicle):
def __init__(self, brand, model):
super().__init__(brand)
self.model = model
def display_info(self):
parent_info = super().display_info()
return f"{parent_info} It's a {self.model} model."
car = Car("Toyota", "Corolla")
print(car.display_info())
Output:
This is a vehicle of brand Toyota. It's a Corolla model.
In this example, the Vehicle
class has an __init__
method that initializes the brand
attribute and a display_info
method that returns a string with information about the vehicle. The Car
class inherits from Vehicle
and adds an additional model
attribute. By using super().__init__(brand)
, we ensure that the brand
attribute is initialized correctly in the parent class. The display_info
method in the Car
class calls the parent method using super().display_info()
, allowing us to extend the output with model-specific information.
Method 2: Using super() with Multiple Inheritance
Multiple inheritance can introduce complexity, but super()
helps manage this complexity effectively. Let’s consider an example with multiple base classes: Engine
and Wheels
. The Car
class will inherit from both.
class Engine:
def start(self):
return "Engine started."
class Wheels:
def roll(self):
return "Wheels are rolling."
class Car(Engine, Wheels):
def drive(self):
engine_status = super().start()
wheels_status = super().roll()
return f"{engine_status} {wheels_status}"
car = Car()
print(car.drive())
Output:
Engine started. Wheels are rolling.
In this scenario, we have two base classes, Engine
and Wheels
, each with their respective methods. The Car
class inherits from both. By calling super().start()
and super().roll()
, we can access the methods of both parent classes. This demonstrates how super()
can help navigate the method resolution order, ensuring that the correct methods from the appropriate base classes are called.
Method 3: super() in Class Methods
The super()
function can also be used in class methods, which are methods that belong to the class rather than an instance. This can be particularly useful for factory methods or other class-level operations. Let’s see how this works.
class Animal:
species = "Unknown"
@classmethod
def identify(cls):
return f"This is an animal of species {cls.species}."
class Dog(Animal):
species = "Dog"
@classmethod
def identify(cls):
parent_info = super().identify()
return f"{parent_info} It's a domestic {cls.species}."
print(Dog.identify())
Output:
This is an animal of species Dog. It's a domestic Dog.
In this example, we define a class method identify
in both the Animal
and Dog
classes. The Dog
class overrides the identify
method but still calls the parent class’s method using super().identify()
. This allows us to build on the existing functionality while providing specific details relevant to the Dog
class.
Conclusion
The super()
function in Python is an invaluable tool for any programmer working with object-oriented programming and inheritance. It simplifies method calls to parent classes, enhances code readability, and helps manage complexities, especially in multiple inheritance scenarios. By mastering super()
, you can write cleaner, more maintainable code that leverages the full power of Python’s inheritance model. So, the next time you find yourself in a situation involving class inheritance, remember to utilize super()
for a smoother coding experience.
FAQ
-
What is the purpose of the super() function in Python?
The super() function allows you to call methods from a parent class in a child class, facilitating code reuse and enhancing maintainability. -
Can super() be used with multiple inheritance?
Yes, super() can effectively manage method resolution in multiple inheritance scenarios, ensuring the correct methods are called from parent classes.
-
How does super() improve code readability?
By using super(), you avoid explicitly referencing parent class names, making the code easier to read and understand, especially in complex inheritance hierarchies. -
Can super() be used in class methods?
Yes, super() can be used in class methods, allowing you to call methods from parent classes at the class level rather than the instance level. -
Is super() specific to Python?
While many programming languages have similar concepts, the implementation and usage of super() in Python are unique to its object-oriented programming model.