How to Shift or Rotate an Array in Python
-
Use the
collections.deque
Class to Shift Array in Python -
Use the
numpy.roll()
Method to Shift Array in Python - Use Array Slicing to Shift Array in Python
- Use Manual Rotation to Shift Array in Python
- Conclusion
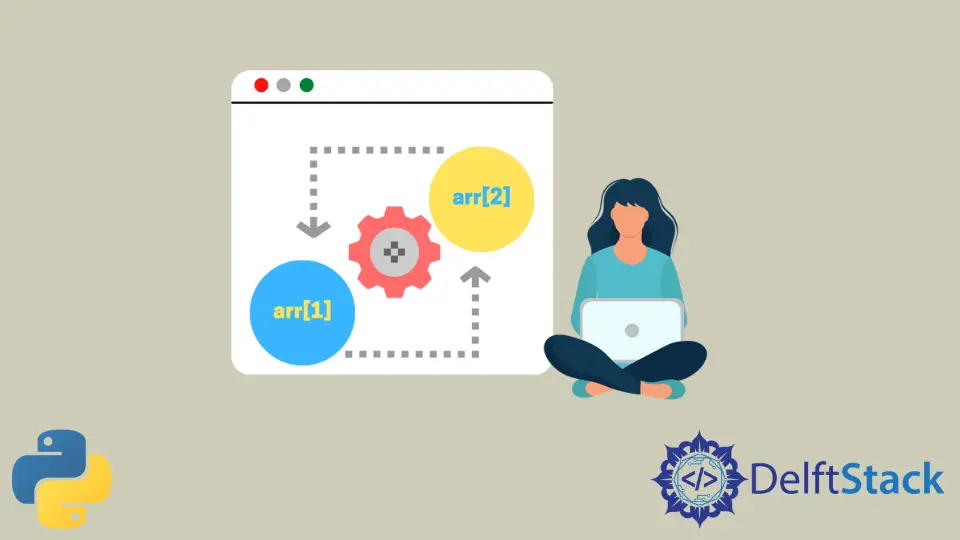
Array rotation, also known as array shifting, is a common operation in Python that involves moving the elements of an array to the left or right by a certain number of positions. This operation is valuable in various scenarios, such as data manipulation, algorithm implementation, and array-based applications.
In this guide, we’ll explore different methods to achieve array rotation in Python, covering both specialized library functions and manual techniques.
Use the collections.deque
Class to Shift Array in Python
Rotating an array in Python can be accomplished using various methods, and one efficient way is by leveraging the collections.deque
class. The deque
class provides a rotate
method that allows us to easily shift or rotate the elements of an array in either the left or right direction.
This method is particularly useful when we want to perform circular shifts efficiently.
Syntax of collections.deque.rotate
:
deque.rotate(n)
Here, deque
is the deque object we want to rotate, and n
is the number of positions by which we want to shift the elements.
The sign of n
determines the direction of rotation. Positive values rotate the deque to the right, while negative values rotate it to the left.
The rotate
method effectively shifts the elements of the deque by n
positions. The rotation is circular, meaning the rightmost elements that are shifted out during rotation reappear at the left end of the deque, and vice versa for leftward rotation.
Now, let’s illustrate the syntax and functionality with a code example.
from collections import deque
my_array = deque([1, 2, 3, 4, 5])
my_array.rotate(2)
print(list(my_array))
my_array.rotate(-3)
print(list(my_array))
Output:
[4, 5, 1, 2, 3]
[2, 3, 4, 5, 1]
In this example, we start by importing the deque
class from the collections
module. We then create a deque named my_array
with an initial set of elements [1, 2, 3, 4, 5]
.
The first rotation is performed using my_array.rotate(2)
, shifting the elements to the right by 2
positions. The result is [4, 5, 1, 2, 3]
.
Next, we rotate the array to the left by 3
positions with my_array.rotate(-3)
. This action shifts the elements to the left, resulting in [2, 3, 4, 5, 1]
.
Finally, we convert the deque back to a list for printing. The output of the two rotations demonstrates the circular nature of the shift, showcasing how the rightmost elements wrap around to the left and vice versa.
Use the numpy.roll()
Method to Shift Array in Python
Another powerful method for shifting or rotating arrays in Python is by utilizing the numpy.roll
function from the NumPy library. NumPy provides a versatile and efficient way to manipulate arrays, and the roll
function allows us to easily achieve circular shifts.
Syntax of numpy.roll
:
numpy.roll(array, shift, axis=None)
Where:
array
: The input array to be rotated.shift
: The number of positions by which elements are to be shifted. Positive values shift elements to the right, while negative values shift them to the left.axis
: The axis or axes along which elements are rolled. If not specified, the array is flattened before rolling.
The numpy.roll
function shifts the elements of an array along the specified axis by a given number of positions. The circular nature of the shift ensures that elements shifted beyond the array boundary reappear at the opposite end.
The direction of the shift is determined by the sign of the shift
parameter.
Now, let’s delve into a practical example to demonstrate the syntax and functionality of numpy.roll
.
import numpy as np
my_array = np.array([1, 2, 3, 4, 5])
new_array = np.roll(my_array, 2)
print(new_array)
new_array = np.roll(my_array, -2)
print(new_array)
Output:
[4 5 1 2 3]
[3 4 5 1 2]
In this example, we begin by importing NumPy as np
. We then create a NumPy array named my_array
with an initial set of elements [1, 2, 3, 4, 5]
.
The first rotation is achieved with np.roll(my_array, 2)
, where the array is shifted to the right by 2
positions. The resulting array is [4, 5, 1, 2, 3]
.
Subsequently, we rotate the array to the left by 2
positions using np.roll(my_array, -2)
. This operation shifts the elements to the left, producing the array [3, 4, 5, 1, 2]
.
Use Array Slicing to Shift Array in Python
Array slicing is a straightforward and native method in Python for shifting or rotating arrays. While it may not be as optimized as some specialized functions, it provides a clear and concise way to achieve circular shifts.
The syntax for rotating an array using array slicing is simple:
result = input_array[n:] + input_array[:n]
Here, input_array
is the array we want to rotate, and n
represents the number of positions by which we want to shift the elements. The slicing notation [:]
extracts elements from the beginning to the end.
The array slicing method operates by separating the input array into two parts: one starting from the n
-th position to the end (input_array[n:]
) and another from the beginning to the n
-th position (input_array[:n]
).
By concatenating these two slices, we effectively achieve the rotation. The rightmost elements that move beyond the array boundary during slicing reappear at the left end, creating a circular shift.
Now, let’s dive into a practical example.
def rotate_array(input_array, n):
return input_array[n:] + input_array[:n]
my_array = [1, 3, 5, 7, 9]
rotated_left = rotate_array(my_array, 2)
print(rotated_left)
rotated_right = rotate_array(my_array, -2)
print(rotated_right)
Output:
[5, 7, 9, 1, 3]
[7, 9, 1, 3, 5]
In this example, we define a function rotate_array
that takes an input array (input_array
) and the number of positions to rotate (n
). The function returns the rotated array using array slicing.
We then create an array named my_array
with an initial set of elements [1, 3, 5, 7, 9]
. The first rotation is performed by calling rotate_array(my_array, 2)
, resulting in the array [5, 7, 9, 1, 3]
.
Similarly, the second rotation is achieved with rotate_array(my_array, -2)
, yielding the array [7, 9, 1, 3, 5]
.
Use Manual Rotation to Shift Array in Python
For scenarios where specialized libraries or methods are not available, manual rotation is a viable option to shift or rotate arrays in Python. Although it may be less concise compared to other methods, it provides a clear understanding of the underlying mechanism.
The syntax for manually rotating an array involves using array slicing and reassigning values:
def rotate_array(input_array, n):
input_array = input_array[-n:] + input_array[:-n]
return input_array
Here, input_array
is the array to be rotated, and n
represents the number of positions to shift the elements.
The slicing notation [-n:]
extracts the last n
elements from the array, and [:-n]
extracts all elements except the last n
. By concatenating these slices, we achieve the rotation.
The manual rotation method simulates the rotation by explicitly swapping array elements.
The input array is divided into two parts: the last n
elements and the remaining elements. These two parts are then swapped and recombined, resulting in a rotated array.
This process emulates the circular shift characteristic of array rotation.
Now, let’s see a practical example to demonstrate how manual rotation works.
def rotate_array(input_array, n):
input_array = input_array[-n:] + input_array[:-n]
return input_array
my_array = [1, 3, 5, 7, 9]
rotated_left = rotate_array(my_array, 2)
print(rotated_left)
rotated_right = rotate_array(my_array, -2)
print(rotated_right)
Output:
[7, 9, 1, 3, 5]
[5, 7, 9, 1, 3]
In this example, we define a function rotate_array
that takes an input array (input_array
) and the number of positions to rotate (n
).
Inside the function, the array is manually rotated by using array slicing to extract the last n
elements and all elements except the last n
. These slices are then concatenated to form the rotated array.
We create an array named my_array
with an initial set of elements [1, 3, 5, 7, 9]
. The first rotation is performed with rotate_array(my_array, 2)
, resulting in the array [5, 7, 9, 1, 3]
.
Similarly, the second rotation is achieved with rotate_array(my_array, -2)
, yielding the array [7, 9, 1, 3, 5]
.
While manual rotation may not be as concise as some other methods, it provides insight into the mechanics of array rotation and serves as a practical solution when specialized functions are not available or necessary. This approach is particularly useful for educational purposes or in situations where a simple, self-contained solution is preferred.
Conclusion
Array rotation is a fundamental operation with various applications in Python programming. Whether you opt for the simplicity of array slicing, the efficiency of specialized library functions like numpy.roll
, or the flexibility of manual rotation, understanding these techniques equips you to handle array manipulation tasks effectively.
Choose the method that best suits your needs based on performance requirements, library availability, and the specific characteristics of your Python project.