How to Flush Print Output in Python
-
Flush Print Output in Python Using the
flush
Parameter in theprint()
Function -
Flush Print Output in Python Using the
sys.stdout.flush()
Method -
Flush Print Output in Python Using the
-u
Flag
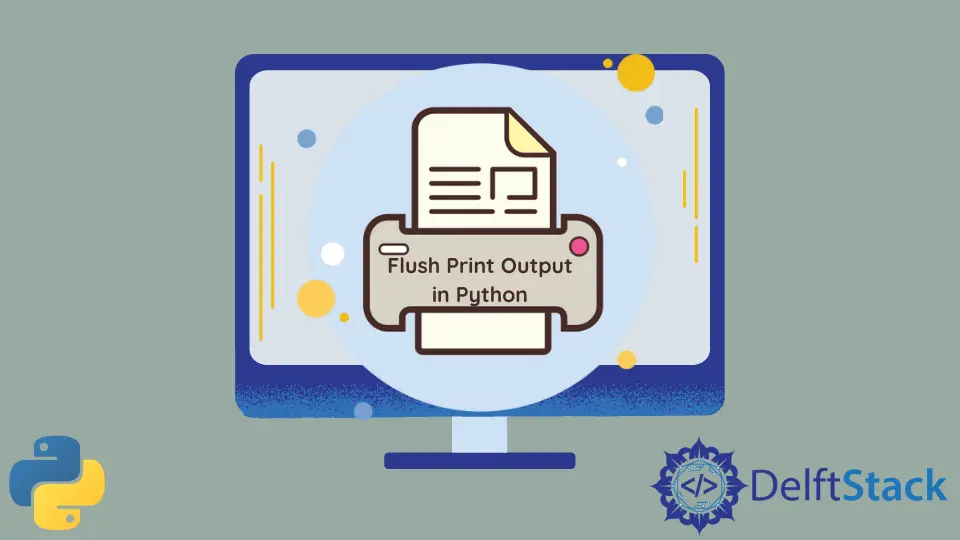
In this tutorial, we will discuss the various ways to flush the output of the print functions like print()
and sys.stdout.write()
to the screen in Python. Generally, the input and output functions store the data into a buffer to improve the program’s performance. Therefore, to lower the number of system calls, the data is stored in a buffer first and then written on the screen, instead of writing it to the screen or file, character by character, etc.
This tutorial will explain the multiple methods to set the print functions to forcibly flush the data on each call instead of buffering it.
Flush Print Output in Python Using the flush
Parameter in the print()
Function
The flush
argument of the print()
function can be set to True
to stop the function from buffering the output data and forcibly flush it. If the flush
argument is set to True
, the print()
function will not buffer the data to increase the efficiency and will keep flushing it on each call.
The example code below demonstrates how to make the print()
function to forcibly flush the print output in Python.
print("This is my string", flush=True)
Flush Print Output in Python Using the sys.stdout.flush()
Method
The other way to flush the output data while printing it is by using the sys.stdout.flush()
of Python’s sys
module. The sys.stdout.flush()
will force the print functions that can be print()
or sys.stdout.write()
to write the output on the screen or file on each call and not buffer it.
The following code example demonstrates how to use the sys.stdout.flush()
method to flush the output data of the print:
import sys
sys.stdout.write("This is my string")
sys.stdout.flush()
Flush Print Output in Python Using the -u
Flag
We can pass the -u
flag to the interpreter while running the .py
file, it will force stdin
, stdout
, and stderr
to not buffer and flush the data while executing the .py
file.
The below example demonstrates how to use the -u
flag to flush the print output in Python.
python -u mycodefile.py