How to Generate Random Colors in Python
- Generate Random Colors in RGB Format in Python
- Generate Random Colors in Hexadecimal Format in Python
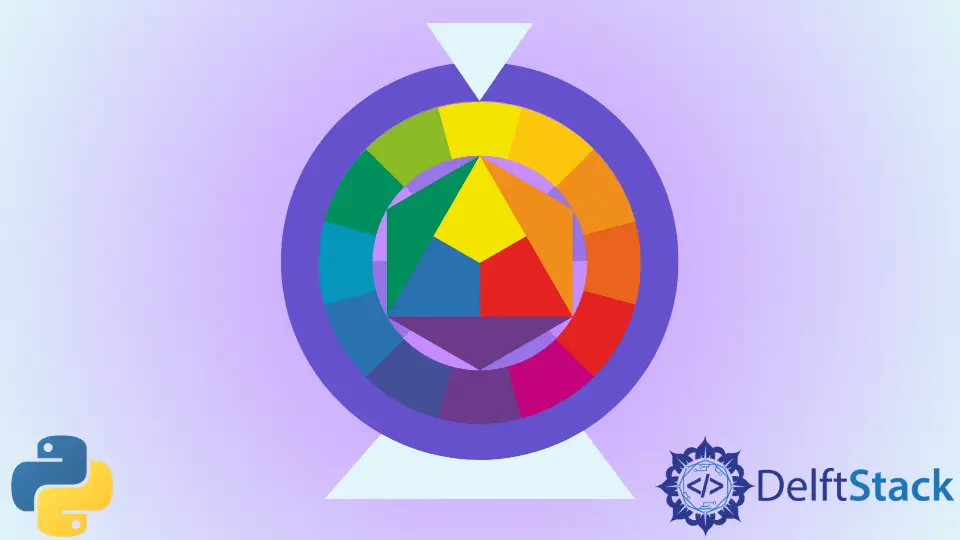
In the digital world, colors are represented in different formats. The RGB, Hexadecimal formats are just a few of the highly used formats.
In this tutorial, we will learn how to generate random colors in Python. When we talk about generating random colors, we will generate a random code that can represent color. Different methods will generate color codes in different formats.
Generate Random Colors in RGB Format in Python
RGB stands for Red, Green, and Blue. Together they represent the color spectrum in the digital world. The Red, Green, and Blue together can represent every color and are of 8 bit each. It means that they have an integer value from 0 to 255.
For generating random colors in RGB format, we will generate a list or tuple of random integers from 0 to 255.
The following code shows how to implement this.
import numpy as np
color = list(np.random.choice(range(256), size=3))
print(color)
Output:
[245, 159, 34]
We generate random integers using the random
from the NumPy
module in the above code. It simply generates a random integer from 0 to 255 three times and stores it in a list. The main focus should be on the logic of the code since random integers can be generated in many other ways.
Generate Random Colors in Hexadecimal Format in Python
In the Hexadecimal, the color is represented in six hexadecimal digits, prefixed by a # sign. The format is in #RRGGBB
where R, G, and B indicate Red
, Green
, and Blue
, respectively, and are hexadecimal numbers.
We can generate random colors in this format using the code as shown below.
import random
color = ["#" + "".join([random.choice("0123456789ABCDEF") for j in range(6)])]
print(color)
Output:
['#BE3559']
In the above code, we pick six random numbers from the specified hexadecimal digits and merge them with a #
sign using the join()
function.
There are many other color formats available and it is very easy to carry out conversions between them.
One thing to remember is that we generated color codes in this tutorial in different formats. To actually see these colors, we would have to produce some graphic or plot some graph using other modules.
For example, in the code below, we will plot a simple dot of the color code we produce using a scatterplot of the Matplotlib
module.
import random
import matplotlib.pyplot as plt
color = ["#" + "".join([random.choice("0123456789ABCDEF") for j in range(6)])]
print(color)
plt.scatter(random.randint(0, 10), random.randint(0, 10), c=color, s=200)
plt.show()
Output:
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn