How to Find String in List in Python
-
Use the
for
Loop to Find Elements From a List That Contain a Specific Substring in Python -
Use the
filter()
Function to Find Elements From a Python List Which Contain a Specific Substring - Use the Regular Expressions to Find Elements From a Python List Which Contain a Specific Substring
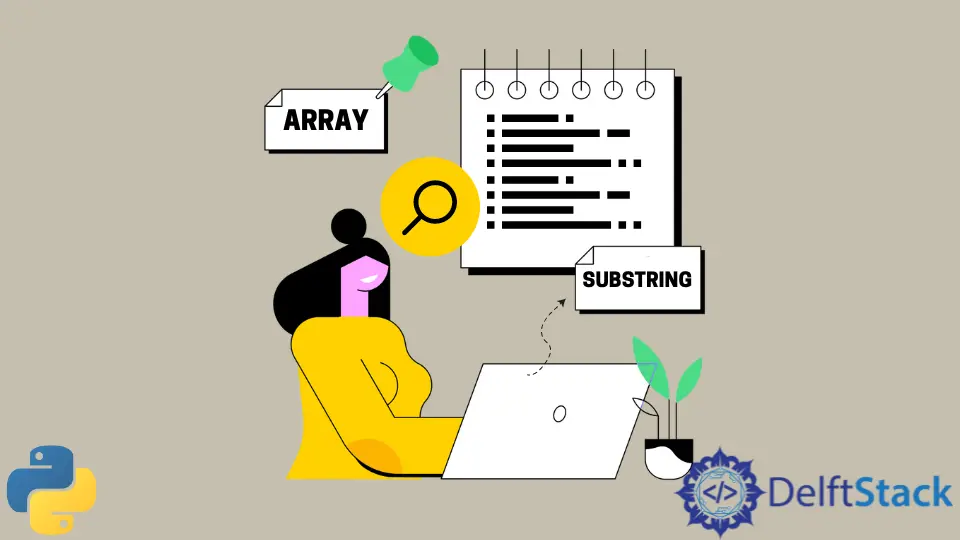
This tutorial will introduce how to find elements from a Python list that have a specific substring in them.
We will work with the following list and extract strings that have ack
in them.
my_list = ["Jack", "Mack", "Jay", "Mark"]
Use the for
Loop to Find Elements From a List That Contain a Specific Substring in Python
In this method, we iterate through the list and check whether the substring is present in a particular element or not. If the substring is present in the element, then we store it in the string. The following code shows how:
str_match = [s for s in my_list if "ack" in s]
print(str_match)
Output:
['Jack', 'Mack']
The in
keyword checks whether the given string, "ack"
in this example, is present in the string or not. It can also be replaced by the __contains__
method, which is a magic method of the string class. For example:
str_match = [s for s in my_list if s.__contains__("ack")]
print(str_match)
Output:
['Jack', 'Mack']
Use the filter()
Function to Find Elements From a Python List Which Contain a Specific Substring
The filter()
function retrieves a subset of the data from a given object with the help of a function. This method will use the lambda
keyword to define the condition for filtering data. The lambda
keyword creates a one-line lambda
function in Python. See the following code snippet.
str_match = list(filter(lambda x: "ack" in x, my_list))
print(str_match)
Output:
['Jack', 'Mack']
Use the Regular Expressions to Find Elements From a Python List Which Contain a Specific Substring
A regular expression is a sequence of characters that can act as a matching pattern to search for elements. To use regular expressions, we have to import the re
module. In this method, we will use the for
loop and the re.search()
method, which is used to return an element that matches a specific pattern. The following code will explain how:
import re
pattern = re.compile(r"ack")
str_match = [x for x in my_list if re.search("ack", x)]
print(str_match)
Output:
['Jack', 'Mack']
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python List
- How to Convert a Dictionary to a List in Python
- How to Remove All the Occurrences of an Element From a List in Python
- How to Remove Duplicates From List in Python
- How to Get the Average of a List in Python
- What Is the Difference Between List Methods Append and Extend
- How to Convert a List to String in Python