How to Close a Tkinter Window With a Button
-
root.destroy()
Class Method to Close the Tkinter Window -
destroy()
Non-Class Method to Close the Tkinter Window -
Associate
root.destroy
Function to thecommand
Attribute of the Button Directly -
root.quit
to Close the Tkinter Window
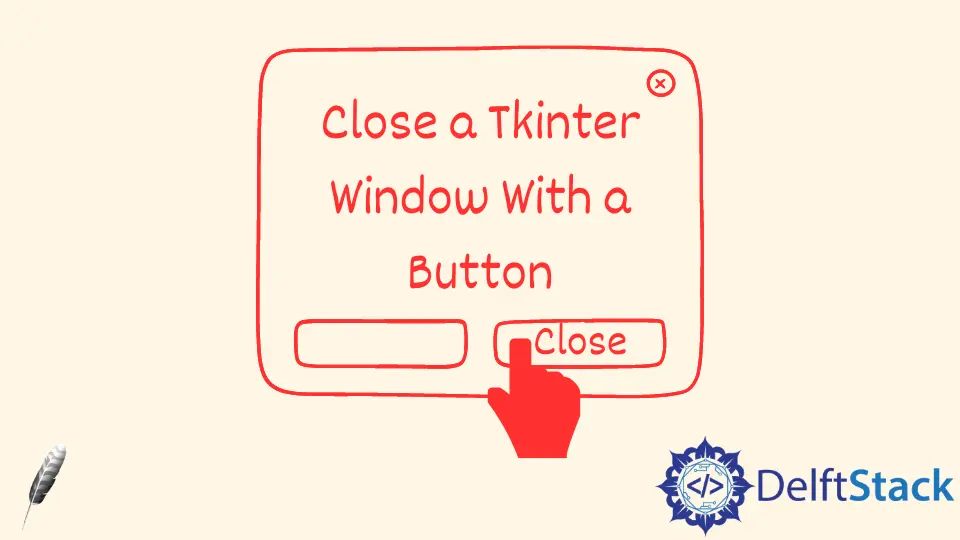
We can use a function or command attached to a Tkinter button in the Tkinter GUI to close the Tkinter window when the user clicks it.
root.destroy()
Class Method to Close the Tkinter Window
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("100x50")
button = tk.Button(self.root, text="Click and Quit", command=self.quit)
button.pack()
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
destroy()
method destroys or closes the window. We make a separate quit
method and then bind it to the command
of the button.
We could also directly set the command
argument to be self.root.destroy
as below.
try:
import Tkinter as tk
except:
import tkinter as tk
class Test:
def __init__(self):
self.root = tk.Tk()
self.root.geometry("100x50")
button = tk.Button(self.root, text="Click and Quit", command=self.root.destroy)
button.pack()
self.root.mainloop()
def quit(self):
self.root.destroy()
app = Test()
destroy()
Non-Class Method to Close the Tkinter Window
try:
import Tkinter as tk
except:
import tkinter as tk
root = tk.Tk()
root.geometry("100x50")
def close_window():
root.destroy()
button = tk.Button(text="Click and Quit", command=close_window)
button.pack()
root.mainloop()
Associate root.destroy
Function to the command
Attribute of the Button Directly
We could directly bind root.destroy
function to the button command
attribute without defining the extra function close_window
any more.
try:
import Tkinter as tk
except:
import tkinter as tk
root = tk.Tk()
root.geometry("100x50")
button = tk.Button(text="Click and Quit", command=root.destroy)
button.pack()
root.mainloop()
root.quit
to Close the Tkinter Window
root.quit
quits not only the Tkinter Window but, more precisely, the whole Tcl interpreter.
It could be used if your Tkinter app is not initiated from Python IDLE
. We don’t recommend to use root.quit
if your Tkinter app is called from IDLE
because quit
will kill not only your Tkinter app but also the IDLE
because IDLE
is also a Tkinter application.
try:
import Tkinter as tk
except:
import tkinter as tk
root = tk.Tk()
root.geometry("100x50")
button = tk.Button(text="Click and Quit", command=root.quit)
button.pack()
root.mainloop()
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook