How to Filter Pandas DataFrame With Multiple Conditions
- Filter Entries of a DataFrame Based on Multiple Conditions Using the Indexing
-
Filter Entries of a DataFrame Based on Multiple Conditions Using the
query()
Method
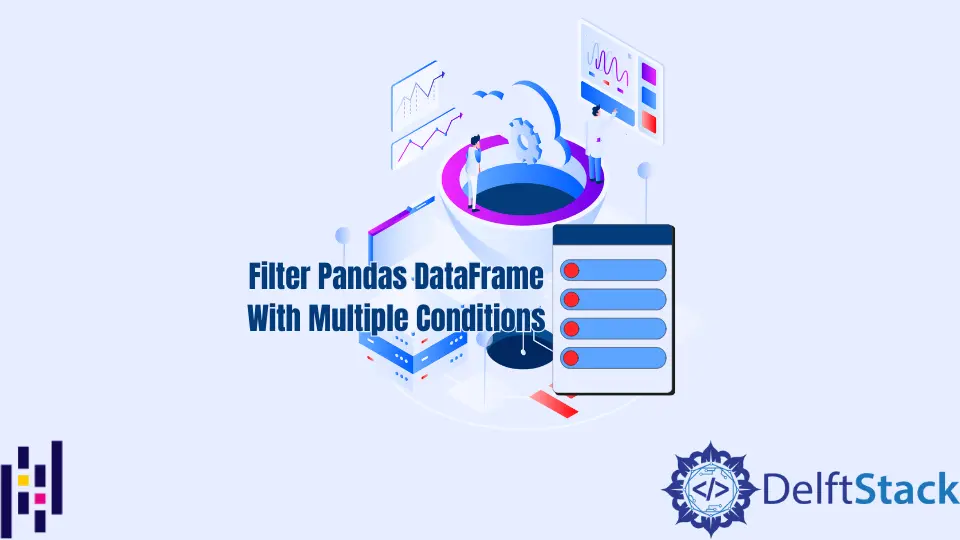
This tutorial explains how we can filter entries from a DataFrame based on multiple conditions.
We will use the below DataFrame in this article.
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Tesla", "Moderna Inc", "Facebook", "Boeing"],
"Price": [835, 112, 267, 209],
"Sector": ["Technology", "Health Technology", "Technology", "Aircraft"],
}
)
print(stocks_df)
Output:
Stock Price Sector
0 Tesla 835 Technology
1 Moderna Inc 112 Health Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
Filter Entries of a DataFrame Based on Multiple Conditions Using the Indexing
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Tesla", "Moderna Inc", "Facebook", "Boeing"],
"Price": [835, 112, 267, 209],
"Sector": ["Technology", "Health Technology", "Technology", "Aircraft"],
}
)
print("Stocks DataFrame:")
print(stocks_df, "\n")
reqd_stocks = stocks_df[(stocks_df.Sector == "Technology") & (stocks_df.Price < 500)]
print("The stocks of technology sector with price less than 500 are:")
print(reqd_stocks)
Output:
Stocks DataFrame:
Stock Price Sector
0 Tesla 835 Technology
1 Moderna Inc 112 Health Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
The stocks of technology sector with price less than 500 are:
Stock Price Sector
2 Facebook 267 Technology
It filters all the entries in the stocks_df
, whose value of the Sector
column is Technology
and the value of the Price
column is less than 500.
We specify the conditions inside []
connecting the conditions using the &
or the |
operator to index the values based on multiple conditions. The &
operator represents the logic and
meaning both the conditions must be true to select an entry. The |
operator represents the logic or
meaning if any condition is satisfied to select the entry.
Filter Entries of a DataFrame Based on Multiple Conditions Using the query()
Method
We pass the multiple conditions connected by the &
or the |
operator as an argument to the query()
method.
import pandas as pd
stocks_df = pd.DataFrame(
{
"Stock": ["Tesla", "Moderna Inc", "Facebook", "Boeing"],
"Price": [835, 112, 267, 209],
"Sector": ["Technology", "Health Technology", "Technology", "Aircraft"],
}
)
print("Stocks DataFrame:")
print(stocks_df, "\n")
reqd_stocks = stocks_df.query("Sector == 'Technology' & Price <500")
print("The stocks of technology sector with price less than 500 are:")
print(reqd_stocks)
Output:
Stocks DataFrame:
Stock Price Sector
0 Tesla 835 Technology
1 Moderna Inc 112 Health Technology
2 Facebook 267 Technology
3 Boeing 209 Aircraft
The stocks of technology sector with price less than 500 are:
Stock Price Sector
2 Facebook 267 Technology
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn