How to Copy Pandas DataFrame
-
pandas.DataFrame.copy()
Method Syntax -
Copy Pandas DataFrame Using the
pandas.DataFrame.copy()
Method - Copy Pandas DataFrame by Assigning the DataFrame to a Variable
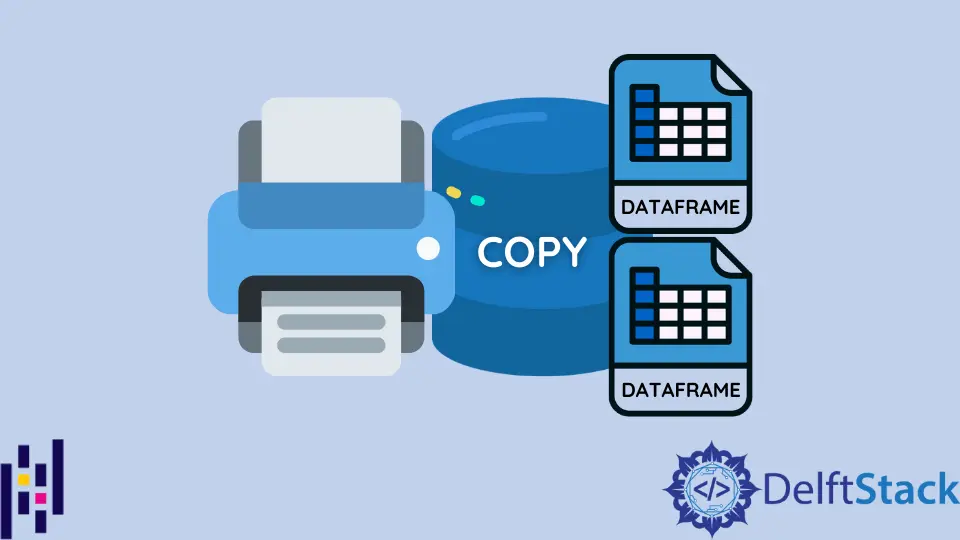
This tutorial will introduce how we can copy a DataFrame object using the DataFrame.copy()
method.
import pandas as pd
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
print(items_df)
Output:
Id Cost
0 302 300
1 504 400
2 708 350
We will use the above example to demonstrate the use of the DataFrame.copy()
method in Pandas.
pandas.DataFrame.copy()
Method Syntax
DataFrame.copy(deep=True)
It returns a copy of the DataFrame
. deep
is by default True
, which means that any change made in the copy will not be reflected in the original DataFrame. However, if we set deep=False
, any changes made in the copy will also be reflected in the original DataFrame.
Copy Pandas DataFrame Using the pandas.DataFrame.copy()
Method
import pandas as pd
import numpy as np
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
deep_copy = items_df.copy()
print("Original DataFrame before changing value in copy DataFrame:")
print(items_df, "\n")
print("Copy DataFrame before changing value in copy DataFrame:")
print(deep_copy, "\n")
deep_copy.loc[0, "Cost"] = np.nan
print("Original DataFrame after changing value in copy DataFrame:")
print(items_df, "\n")
print("Copy DataFrame after changing value in copy DataFrame:")
print(deep_copy, "\n")
Output:
Original DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Copy DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Original DataFrame after changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Copy DataFrame after changing value in copy DataFrame:
Id Cost
0 302 NaN
1 504 400
2 708 350
It creates a copy of the DataFrame items_df
as deep_copy
. If we change any value of the copy deep_copy
, there is no change in the original DataFrame items_df
. We set the value of the Cost
column of the 1st row to NaN
in deep_copy
but items_df
keeps unmodified.
Copy Pandas DataFrame by Assigning the DataFrame to a Variable
import pandas as pd
import numpy as np
items_df = pd.DataFrame(
{
"Id": [302, 504, 708],
"Cost": ["300", "400", "350"],
}
)
copy_cost = items_df["Cost"]
print("Cost column of Original DataFrame before changing value in copy DataFrame:")
print(items_df, "\n")
print("Cost column of Copied DataFrame before changing value in copy DataFrame:")
print(copy_cost, "\n")
copy_cost[0] = np.nan
print("Cost column of Original DataFrame after changing value in copy DataFrame:")
print(copy_cost, "\n")
print("Cost column of Copied DataFrame after changing value in copy DataFrame:")
print(copy_cost, "\n")
Output:
Cost column of Original DataFrame before changing value in copy DataFrame:
Id Cost
0 302 300
1 504 400
2 708 350
Cost column of Copied DataFrame before changing value in copy DataFrame:
0 300
1 400
2 350
Name: Cost, dtype: object
Cost column of Original DataFrame after changing value in copy DataFrame:
0 NaN
1 400
2 350
Name: Cost, dtype: object
Cost column of Copied DataFrame after changing value in copy DataFrame:
0 NaN
1 400
2 350
Name: Cost, dtype: object
It creates a copy of the Cost
column of the DataFrame items_df
as copy_cost
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn