How to Add Header Row to a Pandas DataFrame
-
Add Pandas
DataFrame
header
Row (Pandas DataFrame Column Names) by Directly Passing It inDataFrame
Method -
Add Pandas
DataFrame
header
Row (Pandas DataFrame Column Names) by Usingdataframe.columns
-
Add Pandas
DataFrame
header
Row (Pandas DataFrame Column Names) Without Replacing Currentheader
-
Add Pandas DataFrame
header
Row (Pandas DataFrame Column Names) toDataFrame
When Reading CSV Files
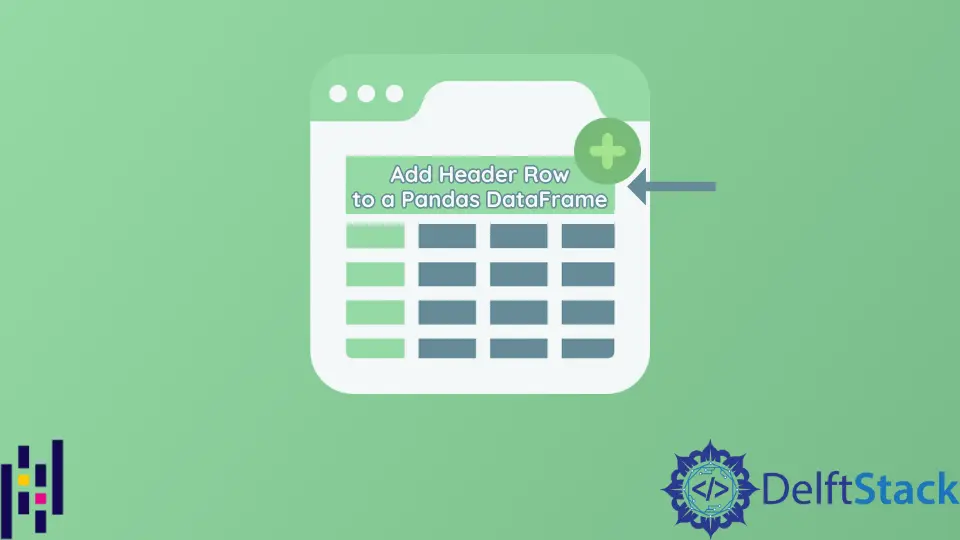
We have introduced how to add a row to Pandas DataFrame but it doesn’t work if we need to add the header row. We will introduce the method to add a header row to a pandas DataFrame
, and options like by passing names
directly in the DataFrame
or by assigning the column names directly in a list to the dataframe.columns
method.
We will also introduce how to add Pandas DataFrame
header without replacing the current header. In other words, we will shift the current header down and add it to the DataFrame
as one row.
We will also look at the example of how to add a header row to a DataFrame
while reading csv files.
Add Pandas DataFrame
header
Row (Pandas DataFrame Column Names) by Directly Passing It in DataFrame
Method
We will directly pass a header
to DataFrame
by using the columns
argument.
Example Codes:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
print(df)
Output:
a b c d
0 4 4 4 0
1 8 1 2 5
2 3 0 4 3
3 3 7 2 4
4 8 3 1 8
5 6 7 5 9
Add Pandas DataFrame
header
Row (Pandas DataFrame Column Names) by Using dataframe.columns
We can also add header
row to DataFrame
by using dataframe.columns
.
Example Codes:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)))
df.columns = ["a", "b", "c", "d"]
print(df)
Output:
a b c d
0 5 2 6 7
1 4 5 9 0
2 8 3 0 4
3 6 3 1 1
4 9 3 4 8
5 7 5 0 6
Add Pandas DataFrame
header
Row (Pandas DataFrame Column Names) Without Replacing Current header
Another option is to add the header row as an additional column index level to make it a MultiIndex. This approach is helpful when we need an extra layer of information for columns.
Example Codes:
# python 3.x
import pandas as pd
import numpy as np
df = pd.DataFrame(data=np.random.randint(0, 10, (6, 4)), columns=["a", "b", "c", "d"])
df.columns = pd.MultiIndex.from_tuples(zip(["A", "B", "C", "D"], df.columns))
print(df)
Output:
A B C D
a b c d
0 2 6 4 6
1 5 0 5 1
2 9 6 6 1
3 8 9 7 4
4 6 5 6 6
5 3 9 1 5
Add Pandas DataFrame header
Row (Pandas DataFrame Column Names) to DataFrame
When Reading CSV Files
We can use names
directly in the read_csv
, or set header=None
explicitly if a file has no header.
Example Codes:
# python 3.x
import pandas as pd
import numpy as np
df = pd.Cov = pd.read_csv("path/to/file.csv", sep="\t", names=["a", "b", "c", "d"])