How to Extract Date From Timestamp in PostgreSQL
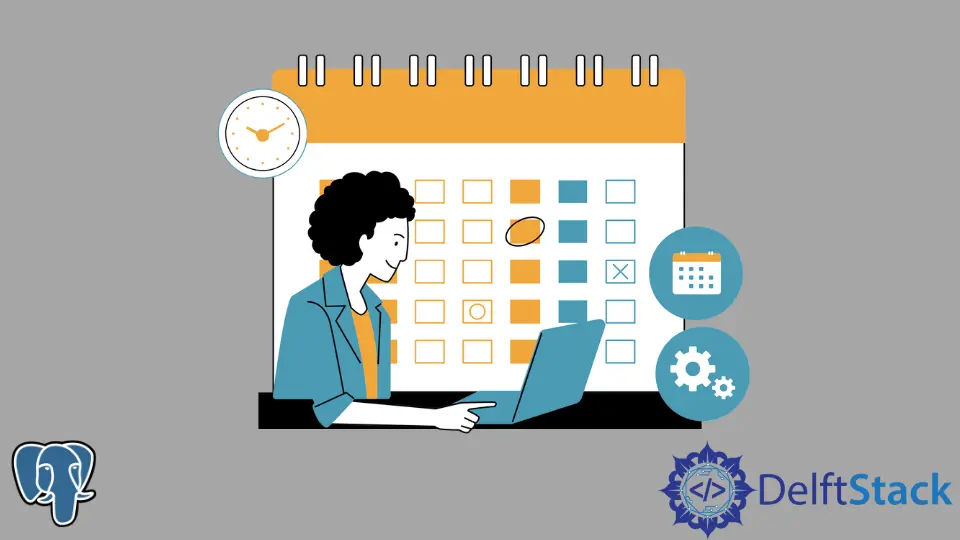
Extracting the date from a timestamp in PostgreSQL is a common task that many developers encounter. Whether you’re working on a data analysis project, building a web application, or managing databases, knowing how to manipulate timestamps is crucial. PostgreSQL provides several methods to extract the date portion from a timestamp, each with its own use case. In this tutorial, we will explore different techniques to achieve this, focusing on practical examples and Python code snippets. By the end of this guide, you will have a solid understanding of how to effectively extract dates from timestamps in PostgreSQL, enhancing your database management skills.
Using the DATE Function
The simplest way to extract the date from a timestamp in PostgreSQL is by using the DATE
function. This function converts a timestamp into a date type, effectively stripping off the time component. Here’s how you can implement it in Python using the psycopg2
library, which allows you to interact with PostgreSQL databases.
import psycopg2
connection = psycopg2.connect(
database="your_database",
user="your_username",
password="your_password",
host="localhost",
port="5432"
)
cursor = connection.cursor()
cursor.execute("SELECT DATE('2023-10-05 14:30:00') AS extracted_date;")
result = cursor.fetchone()
print(result[0])
cursor.close()
connection.close()
Output:
2023-10-05
In this example, we first establish a connection to the PostgreSQL database. We then create a cursor object to execute our SQL query. The query uses the DATE
function to extract the date from a timestamp. Finally, we fetch the result and print it. This method is straightforward and works well for basic date extraction needs.
Using the TO_CHAR Function
Another effective way to extract the date from a timestamp is by using the TO_CHAR
function. This function allows you to format the timestamp in various ways, providing flexibility in how you want to display the date. Here’s how to use it in Python.
import psycopg2
connection = psycopg2.connect(
database="your_database",
user="your_username",
password="your_password",
host="localhost",
port="5432"
)
cursor = connection.cursor()
cursor.execute("SELECT TO_CHAR('2023-10-05 14:30:00'::timestamp, 'YYYY-MM-DD') AS formatted_date;")
result = cursor.fetchone()
print(result[0])
cursor.close()
connection.close()
Output:
2023-10-05
In this code snippet, we again connect to the PostgreSQL database and execute a query. The TO_CHAR
function formats the timestamp according to the specified pattern (‘YYYY-MM-DD’), which gives us the date in a clear format. This method is particularly useful when you need the date in a specific string format for reporting or display purposes.
Using the EXTRACT Function
The EXTRACT
function is another powerful tool for extracting parts of a timestamp. It allows you to retrieve specific components, such as the year, month, or day. Here’s how to use it in Python.
import psycopg2
connection = psycopg2.connect(
database="your_database",
user="your_username",
password="your_password",
host="localhost",
port="5432"
)
cursor = connection.cursor()
cursor.execute("SELECT EXTRACT(YEAR FROM '2023-10-05 14:30:00'::timestamp) AS year, "
"EXTRACT(MONTH FROM '2023-10-05 14:30:00'::timestamp) AS month, "
"EXTRACT(DAY FROM '2023-10-05 14:30:00'::timestamp) AS day;")
result = cursor.fetchone()
print(f"Year: {result[0]}, Month: {result[1]}, Day: {result[2]}")
cursor.close()
connection.close()
Output:
Year: 2023, Month: 10, Day: 5
In this example, we use the EXTRACT
function to retrieve the year, month, and day separately from the timestamp. Each component is extracted with its own call to EXTRACT
, and we print the results in a formatted string. This method is ideal when you need to work with individual date components rather than the full date.
Conclusion
Extracting the date from a timestamp in PostgreSQL can be accomplished through various methods, including using the DATE
, TO_CHAR
, and EXTRACT
functions. Each method has its own advantages, depending on your specific needs. Whether you require a simple date, a formatted string, or individual components, PostgreSQL provides the tools to get the job done efficiently. By implementing these techniques in your Python applications, you can enhance your database interactions and streamline your data processing tasks.
FAQ
-
What is the difference between DATE and TO_CHAR in PostgreSQL?
DATE extracts the date portion from a timestamp, while TO_CHAR formats the timestamp into a specified string format. -
Can I extract the time from a timestamp in PostgreSQL?
Yes, you can use the EXTRACT function to retrieve specific time components like hours, minutes, and seconds. -
Is it necessary to use Python to extract dates from timestamps in PostgreSQL?
No, you can execute SQL queries directly in PostgreSQL without using Python, but using Python can help automate and manage database interactions more effectively. -
What library do I need to connect Python to PostgreSQL?
Thepsycopg2
library is a commonly used library for connecting Python applications to PostgreSQL databases. -
Are there performance differences between these methods?
Generally, the performance difference is negligible for small datasets, but for large datasets, using the most efficient method for your needs is advisable.