How to Remove Duplicate Values From an Array in PHP
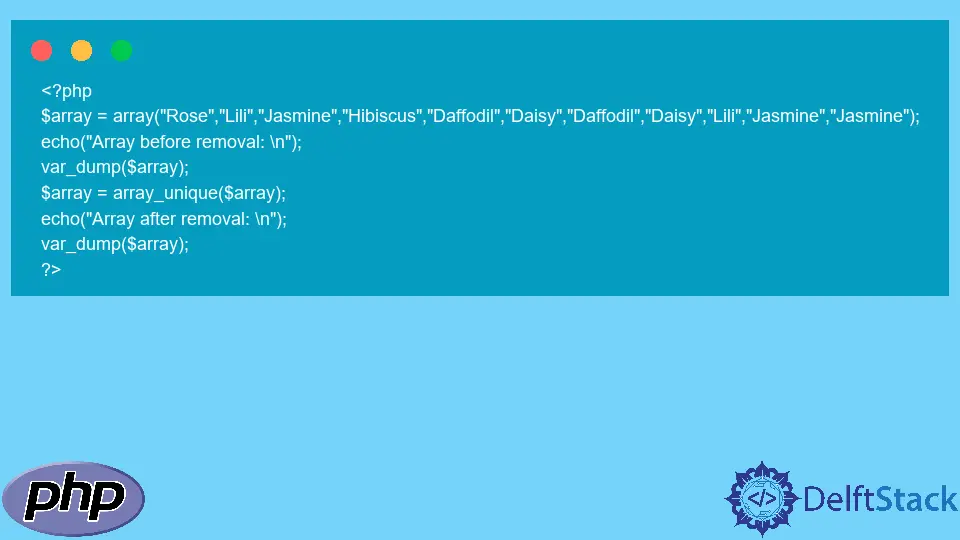
This article will introduce a method to remove duplicate values from an array in PHP.
Use the array_unique()
Function to Remove Duplicate Values From an Array in PHP
We can use the array_unique()
function to remove duplicate values from an array. The array_unique()
function is a specialized function for removing the duplicate values from an array. The correct syntax to use this function is as follows.
array_unique($array, $flags);
The array_unique()
function has two parameters. The details of its parameters are as follows.
Variables | Description |
---|---|
$array |
It is the array from which we want to remove the duplicate values. |
$flags |
It specifies the sorting pattern for the array. The sorting flags are of five types. SORT_REGULAR compares items normally SORT_NUMERIC compares items numerically SORT_STRING compares items as strings SORT_LOCALE_STRING compares items as strings, based on the current locale. |
This function returns the array free of duplicate values. The program below shows the ways by which we can use the array_unique()
function to remove duplicate values from an array in PHP.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy","Daffodil","Daisy","Lili","Jasmine","Jasmine");
echo("Array before removal: \n");
var_dump($array);
$array = array_unique($array);
echo("Array after removal: \n");
var_dump($array);
?>
Output:
Array before removal:
array(11) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
[8]=>
string(4) "Lili"
[9]=>
string(7) "Jasmine"
[10]=>
string(7) "Jasmine"
}
Array after removal:
array(6) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
}
The function has returned the filtered array.
Now, if we pass the $flags
parameter, the output will be changed.
<?php
$array = array("Rose","Lili","Jasmine","Hibiscus","Daffodil","Daisy","Daffodil","Daisy","Lili","Jasmine","Jasmine");
echo("Array before removal: \n");
var_dump($array);
$array = array_unique($array, SORT_NUMERIC);
echo("Array after removal: \n");
var_dump($array);
?>
Output:
Array before removal:
array(11) {
[0]=>
string(4) "Rose"
[1]=>
string(4) "Lili"
[2]=>
string(7) "Jasmine"
[3]=>
string(8) "Hibiscus"
[4]=>
string(8) "Daffodil"
[5]=>
string(5) "Daisy"
[6]=>
string(8) "Daffodil"
[7]=>
string(5) "Daisy"
[8]=>
string(4) "Lili"
[9]=>
string(7) "Jasmine"
[10]=>
string(7) "Jasmine"
}
Array after removal:
array(1) {
[0]=>
string(4) "Rose"
}
The function has now sorted the array numerically.