Abstract Class vs. Interface in PHP
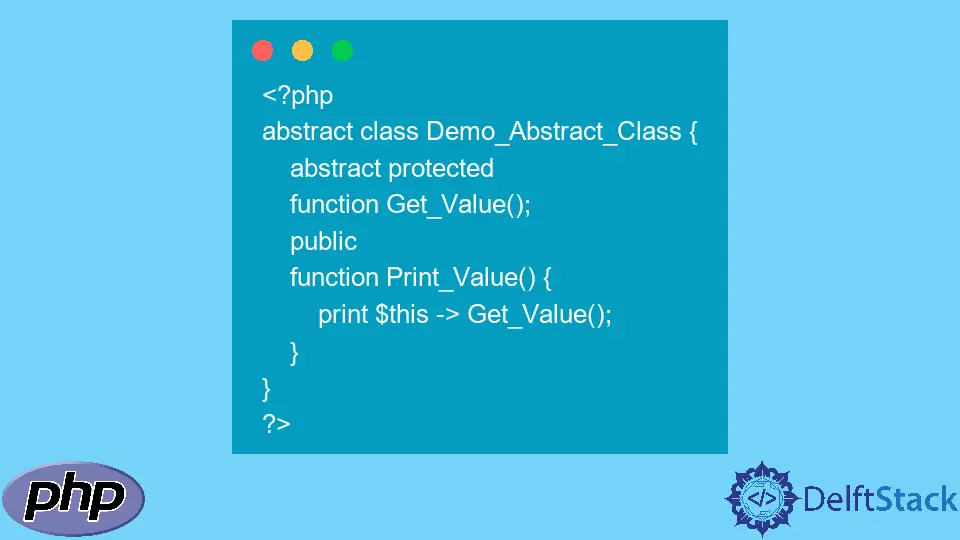
This tutorial demonstrates the difference between abstract class
and interface
in PHP.
Before going into the differences, first, we must understand the concepts of abstract class
and interface
. Here are the important points for both abstract class
and interface
.
Abstract Class in PHP
The abstract class
is partially implemented by developers and contains at least one abstract method, a method without any code. Here are some important points to understand the abstract class
.
- The
abstract class
is under partial abstraction. - We can create variables in the
abstract class
. - Abstract classes can contain abstract and non-abstract methods.
- We can use any access modifier in the
abstract class
. - We can access the
abstract class
features from the derived class using theextends
keyword. - In the
abstract class
, multiple inheritances are possible.
Here is an example of how to create an abstract class
.
<?php
abstract class Demo_Abstract_Class {
abstract protected
function Get_Value();
public
function Print_Value() {
print $this -> Get_Value();
}
}
?>
Interface in PHP
The interface is defined by the interface
keyword, where all the methods are abstract. Here are some important points to understand the interface
.
- Interface is under full abstraction.
- We cannot create variables in the
interface
. - The
interface
only contains abstract methods. - Only the
public
access modifier is used in theinterface
. - We can get the
interface
from a derived class using theimplement
keyword. - Multiple inheritances are possible with
interfaces
.
Here is an example to demonstrate the interface
.
<?php
interface Demo_Interface {
public
function execute();
}
?>
Abstract Class vs. Interface in PHP
Here are the differences between abstract class
and interface
.
Abstract Class | Interface |
---|---|
No Multiple Inheritance. | Supports Multiple Inheritance. |
The abstract class contains a data member. |
The interface does not contain a data member. |
The abstract class supports the containers. |
The interface does not support the containers. |
The abstract class contains complete and incomplete members. |
The interface contains only the complete members, which also refers to the member’s signature. |
The abstract class can have access modifiers with subs, properties, and functions. |
In the interface , everything is considered public, which is why it does not have access modifiers. |
The complete members of the abstract class can be static, while the incomplete members cannot be static. |
No member of the interface can be static. |
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook