How to Use jQuery With Node.js
-
Install jQuery and
jsdom
in a Node.js Project - Require the jQuery Module in a Node.js Script
-
Make HTTP Requests With jQuery’s
$.ajax
Function - Use jQuery’s DOM Manipulation Functions in Node.js
- Handle Events With jQuery and Node.js
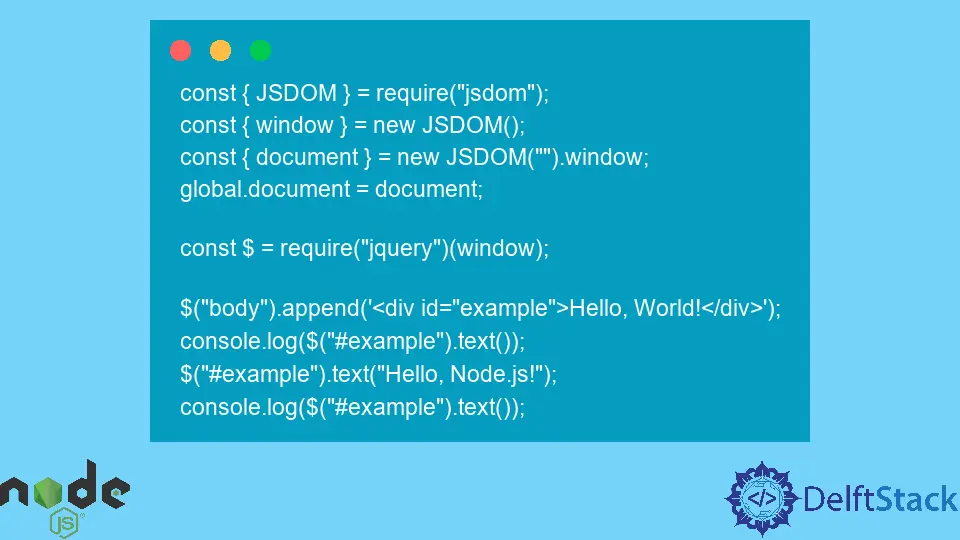
jQuery is a popular JavaScript library widely used for building web applications. It provides a rich set of APIs for interacting with the DOM, making HTTP requests, and handling events, among other things.
Node.js is a JavaScript runtime that allows developers to execute JavaScript code outside a web browser. It provides a rich set of APIs for building server-side applications and is commonly used for building web servers, APIs, and other backend systems.
One interesting aspect of Node.js is that it allows developers to use the same language (JavaScript) for both frontend and backend development. This means that you can also use libraries like jQuery, typically used in the front end, in a Node.js environment.
In this article, we will explore how to use jQuery with Node.js. We will cover the following topics:
- Installing jQuery in a Node.js project.
- Requiring the jQuery module in a Node.js project.
- Making HTTP requests with jQuery’s
$.ajax
function. - Using jQuery’s DOM manipulation functions in a Node.js project.
- Handling events with jQuery in a Node.js project.
Install jQuery and jsdom
in a Node.js Project
The first step in using jQuery in a Node.js project is to install jQuery and jsdom
packages from npm
. To do this, open a terminal window and go to your project directory.
Then, run the following command.
npm install jquery
npm install jsdom
This will install jQuery and jsdom
packages in your project and add them to your package.json
file.
Require the jQuery Module in a Node.js Script
Once you have installed the jQuery package, you can require it in your Node.js script using the require
function.
const $ = require('jquery');
This will create a constant $
that you can use to access jQuery’s functions. Note that when using jQuery in a Node.js environment, the $
object represents the jQuery module rather than the global $
object available in the browser.
Therefore, you will need to use require('jquery')
to access jQuery’s functions rather than relying on the global $
object.
Make HTTP Requests With jQuery’s $.ajax
Function
One of the most useful features of jQuery is its $.ajax
function, which allows you to make HTTP requests to a server. You can use $.ajax
to send GET
, POST
, PUT
, DELETE
, and other requests, and you can also specify the data you want to send with the request.
Here is an example of how you can use $.ajax
to send a GET
request to an API endpoint.
const {JSDOM} = require('jsdom');
const {window} = new JSDOM();
const {document} = new JSDOM('').window;
global.document = document;
const $ = require('jquery')(window);
$.ajax({
url: 'https://jsonplaceholder.typicode.com/todos/1',
type: 'GET',
success: function(data) {
console.log(data);
},
});
Output:
In this example, we send a GET
request to the https://api.example.com/endpoint
URL. If the request is successful, the success callback function will be called with the response data as an argument.
$.ajax
also supports many other options that you can use to customize the behavior of the request. For example, you can specify a timeout to abort the request if it takes too long or a beforeSend
callback to execute some code before the request is sent.
Use jQuery’s DOM Manipulation Functions in Node.js
You can use jQuery to select elements in the virtual DOM and manipulate their properties. For example, you can change the text of an element using the text()
method.
const {JSDOM} = require('jsdom');
const {window} = new JSDOM();
const {document} = new JSDOM('').window;
global.document = document;
const $ = require('jquery')(window);
$('body').append('<div id="example">Hello, World!</div>');
console.log($('#example').text());
$('#example').text('Hello, Node.js!');
console.log($('#example').text());
Output:
Handle Events With jQuery and Node.js
You can use jQuery to bind event handlers to elements in the virtual DOM. For example, you can attach a click event handler to a button element.
const {JSDOM} = require('jsdom');
const {window} = new JSDOM();
const {document} = new JSDOM('').window;
global.document = document;
const $ = require('jquery')(window);
$('body').append('<div id="example">Hover over me!</div>');
$('#example').mouseenter(function() {
$(this).css('background-color', 'yellow');
});
$('#example').mouseleave(function() {
$(this).css('background-color', 'white');
});
console.log('button pressed');
Output:
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn