Done Callback in MochaJS
-
Use the
Done()
Callback in MochaJS -
Pass Test Case Code Using
Done()
Callback in MochaJS -
Fail Test Case Code Using
Done()
Callback in MochaJS
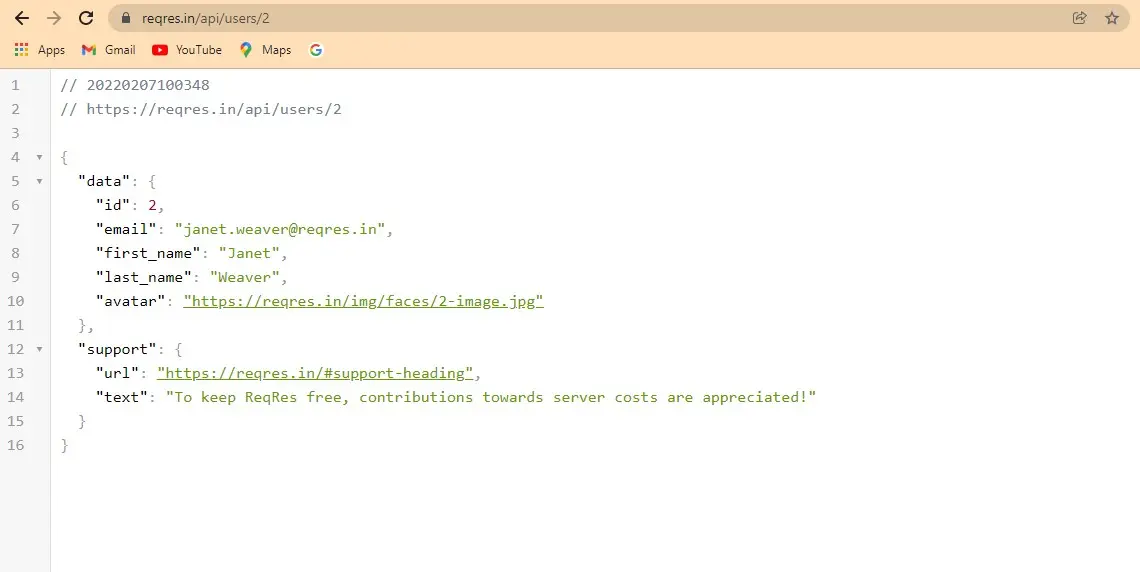
In NodeJS, the code runs asynchronously. MochaJS
is a testing framework of nodeJS
.
Due to this nature, it performs both synchronously and asynchronously. We run a code asynchronously in NodeJS
and test in MochaJS
.
Then our code is called asynchronous due to the control
pass to the calling or main files. The done()
callback means our work is done.
We hand over executing control to file (normally NodeJS
file).
Use the Done()
Callback in MochaJS
const expect = require('chai').expect
const axios = require('axios')
describe('Async suit 1', () => {
it('Async calling done base way',(done)=>{
axios.get('https://reqres.in/api/users/2').then(res=>{
expect(res.data.data.email).to.be.equal('janet.weaver@reqres.in')
done()
}).catch(err=>{
done(err)
})
});
})
In the above example, we wrote a MochaJS
code for testing our API's
output. Initially, we import the expect
package from chaiJS
.
We must import this because we equal our output with our API
. Then we import the axios
package, which is an HTTP Client
.
In the describe
function, we will structure our test/tests because it
can not directly tell that I am a test case
, so we will use describe()
in which we pass the 1st argument as the name of function or function test suit.
In the second parameter, we pass an anonymous function in which we pass a single test
or a group of tests
.
For the it
callback, we will give two arguments first is test name, and the second is function call with any parameter name like abc or any but usually with done.
We will use axiosJS
inside an anonymous function and call its get
method. In the get()
method, we will pass the argument, which is url
.
Then call the then()
method to get the response
from the API
. Inside it, we will extract the data, which is our email
and its equal to our given email and at the end of this, then function.
We will call a done()
callback and tell our system that our work is done. Below it, we will check if any error occurs then we will print it.
Output:
Pass Test Case Code Using Done()
Callback in MochaJS
const expect = require('chai').expect
const axios = require('axios')
describe('Async suit 1', () => {
it('Async calling done base way',(done)=>{
axios.get('https://reqres.in/api/users/2').then(res=>{
expect(res.data.data.email).to.be.equal('janet.weaver@reqres.in')
done()
}).catch(err=>{
done(err)
})
});
})
Output:
Fail Test Case Code Using Done()
Callback in MochaJS
const expect = require('chai').expect
const axios = require('axios')
describe('Async suit 1', () => {
it('Async calling done base way',(done)=>{
axios.get('https://reqres.in/api/users/2').then(res=>{
expect(res.data.data.email).to.be.equal('janet.weaver@reqres.int')
done()
}).catch(err=>{
done(err)
})
});
})
Output: