MongoDB Aggregate Sort
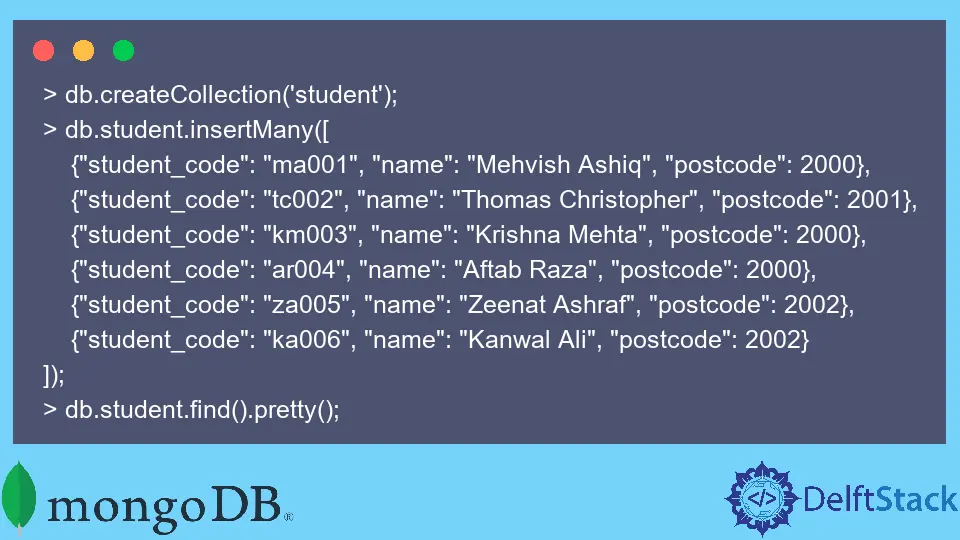
It is necessary to have enough knowledge of aggregation pipeline stages to do aggregation operations using MongoDB.
This tutorial will explore the $sort
stage with an example to perform the MongoDB aggregate sort.
MongoDB Aggregate Sort
Let’s have a scenario to practice the code examples. We have a collection named student
that has basic information.
You can also use the following queries to be on the same page with us.
Example Code:
> db.createCollection('student');
> db.student.insertMany([
{"student_code": "ma001", "name": "Mehvish Ashiq", "postcode": 2000},
{"student_code": "tc002", "name": "Thomas Christopher", "postcode": 2001},
{"student_code": "km003", "name": "Krishna Mehta", "postcode": 2000},
{"student_code": "ar004", "name": "Aftab Raza", "postcode": 2000},
{"student_code": "za005", "name": "Zeenat Ashraf", "postcode": 2002},
{"student_code": "ka006", "name": "Kanwal Ali", "postcode": 2002}
]);
> db.student.find().pretty();
OUTPUT:
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e97b"),
"student_code" : "ma001",
"name" : "Mehvish Ashiq",
"postcode" : 2000
}
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e97c"),
"student_code" : "tc002",
"name" : "Thomas Christopher",
"postcode" : 2001
}
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e97d"),
"student_code" : "km003",
"name" : "Krishna Mehta",
"postcode" : 2000
}
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e97e"),
"student_code" : "ar004",
"name" : "Aftab Raza",
"postcode" : 2000
}
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e97f"),
"student_code" : "za005",
"name" : "Zeenat Ashraf",
"postcode" : 2002
}
{
"_id" : ObjectId("629afe5a0a3b0e96fe92e980"),
"student_code" : "ka006",
"name" : "Kanwal Ali",
"postcode" : 2002
}
Next, we want to determine the postcode
that houses the maximum number of students but in sorted form. To do that, check the following example code.
Example Code:
> db.student.aggregate([
{ $group: { _id: '$postcode', students: { $sum: 1 } } },
{ $sort: {_id: 1} }
]);
OUTPUT:
{ "_id" : 2000, "students" : 3 }
{ "_id" : 2001, "students" : 1 }
{ "_id" : 2002, "students" : 2 }
Here, we are using the aggregate()
function that calculates the aggregate values for data in the specified collection or a view. Inside the aggregate()
method, we group the documents considering the postcode
field.
Further, we return the postcode
as _id
, and the students count in every postcode
as students
. Here, we use the $sum
to get the number of students for every postcode
.
The $sum
calculates and returns the numeric value’s collective sum by ignoring the non-numeric values.
Finally, we use the $sort
stage to sort them according to the project needs in ascending or descending order. The 1
is used for ascending order while -1
is used for descending order.