How to Use of $Match (Aggregation) Stage in MongoDB
-
Use of
$match
(Aggregation) Stage in MongoDB -
Use
$match
With Comparison Operator in MongoDB -
Use
$match
With$project
Stage in MongoDB -
Use
$match
With$and
Operator in MongoDB -
Use
$match
With$or
Operator in MongoDB -
Use
$match
Aggregation With$group
in MongoDB -
Use
$match
Aggregation With$month
and$year
in MongoDB
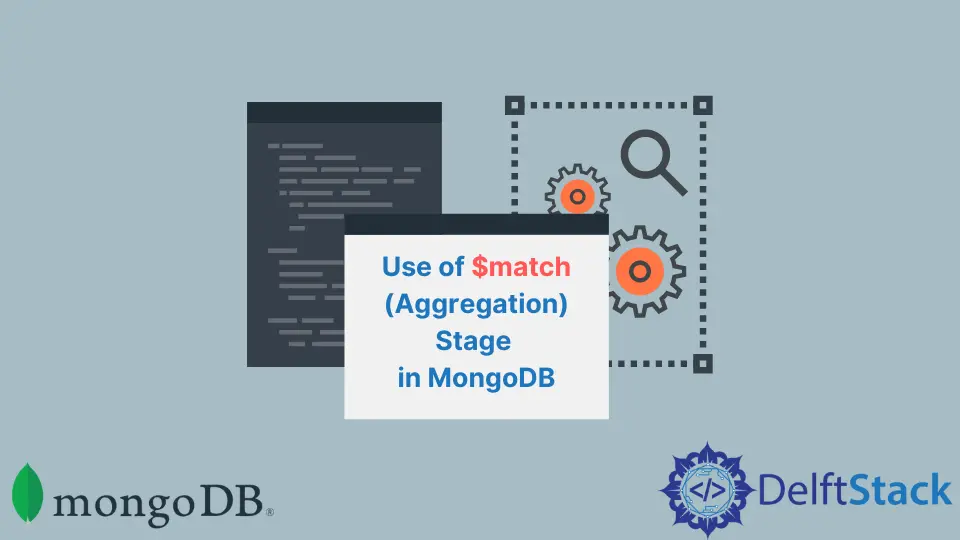
This tutorial briefly discusses the $match
aggregation stage and demonstrates using the $match
stage considering different scenarios.
Use of $match
(Aggregation) Stage in MongoDB
The $match
stage lets us filter the documents (records) that match the specified condition (or set of conditions). Only matching documents will be proceeded to the next stage of a pipeline.
Let’s prepare a collection containing a few documents to learn via code examples.
Example Code:
db.createCollection('employee');
db.employee.insertMany([
{
"emp_code": "ma001",
"emp_age": 30,
"emp_grade": 18,
"emp_joindate": ISODate('2012-08-16T00:00:00Z')
},
{
"emp_code": "tc002",
"emp_age": 40,
"emp_grade":19,
"emp_joindate": ISODate('2011-08-16T00:00:00Z')
},
{
"emp_code": "km003",
"emp_age": 45,
"emp_grade":18,
"emp_joindate": ISODate('2012-08-26T00:00:00Z')
},
{
"emp_code": "ar004",
"emp_age": 32,
"emp_grade":20,
"emp_joindate": ISODate('2014-10-06T00:00:00Z')
},
{
"emp_code": "za005",
"emp_age": 45,
"emp_grade":20,
"emp_joindate": ISODate('2014-03-11T00:00:00Z')
},
{
"emp_code": "ka006",
"emp_age": 35,
"emp_grade": 22,
"emp_joindate": ISODate('2018-06-16T00:00:00Z')
}
]);
db.employee.find().pretty();
Output:
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a0"),
"emp_code" : "ma001",
"emp_age" : 30,
"emp_grade" : 18,
"emp_joindate" : ISODate("2012-08-16T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a1"),
"emp_code" : "tc002",
"emp_age" : 40,
"emp_grade" : 19,
"emp_joindate" : ISODate("2011-08-16T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a2"),
"emp_code" : "km003",
"emp_age" : 45,
"emp_grade" : 18,
"emp_joindate" : ISODate("2012-08-26T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a3"),
"emp_code" : "ar004",
"emp_age" : 32,
"emp_grade" : 20,
"emp_joindate" : ISODate("2014-10-06T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a4"),
"emp_code" : "za005",
"emp_age" : 45,
"emp_grade" : 20,
"emp_joindate" : ISODate("2014-03-11T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a5"),
"emp_code" : "ka006",
"emp_age" : 35,
"emp_grade" : 22,
"emp_joindate" : ISODate("2018-06-16T00:00:00Z")
}
We can move ahead and use the $match
aggregation stage to learn once we are done with creating a collection and inserting documents in it. Considering various situations, let’s use the $match
stage to learn better.
Use $match
With Comparison Operator in MongoDB
Example Code:
db.employee.aggregate([
{
$match:{ "emp_age": { $gt:35 }}
}
]).pretty();
Output:
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a1"),
"emp_code" : "tc002",
"emp_age" : 40,
"emp_grade" : 19,
"emp_joindate" : ISODate("2011-08-16T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a2"),
"emp_code" : "km003",
"emp_age" : 45,
"emp_grade" : 18,
"emp_joindate" : ISODate("2012-08-26T00:00:00Z")
}
{
"_id" : ObjectId("629c376ca4cfbd782e90d5a4"),
"emp_code" : "za005",
"emp_age" : 45,
"emp_grade" : 20,
"emp_joindate" : ISODate("2014-03-11T00:00:00Z")
}
Here, we get only those documents where emp_age
(employee’s age) is greater than 35. The pretty()
function does nothing but displays the output in an organized manner.
Use $match
With $project
Stage in MongoDB
Example Code:
db.employee.aggregate([
{
$match: {
"emp_age": { $gt:35 }
}
},
{
$project:{
"_id": 0,
"emp_code": 1,
"emp_age": 1
}
}
]);
Output:
{ "emp_code" : "tc002", "emp_age" : 40 }
{ "emp_code" : "km003", "emp_age" : 45 }
{ "emp_code" : "za005", "emp_age" : 45 }
This code snippet is similar to the previous example with the additional use of the $project
stage that specifies what fields should be returned from the query. Using 1
and 0
in the $project
stage denotes the field’s inclusion and suppression, respectively.
Remember that the documents returned by the first stage will only be processed at the next stage. We get the emp_code
and emp_age
from all those documents where the emp_age
(employee’s age) is greater than 35.
Use $match
With $and
Operator in MongoDB
Example Code:
db.employee.aggregate([
{
$match:{
$and: [
{ "emp_age": {$gte:32 }},
{ "emp_grade": {$gt: 20}}
]
}
},
{
$project:{
"_id": 0,
"emp_age": 1,
"emp_grade": 1
}
}
]);
Output:
{ "emp_age" : 35, "emp_grade" : 22 }
This example returns the emp_age
and emp_grade
from those documents where the emp_age
is greater than or equal to 32, and emp_grade
is greater than 20. Remember, the resulted documents must meet both conditions.
Use $match
With $or
Operator in MongoDB
Example Code:
db.employee.aggregate([
{
$match:{
$or: [
{ "emp_age": {$gte:32 }},
{ "emp_grade": {$gt: 20}}
]
}
},
{
$project:{
"_id": 0,
"emp_age": 1,
"emp_grade": 1
}
}
]);
Output:
{ "emp_age" : 40, "emp_grade" : 19 }
{ "emp_age" : 45, "emp_grade" : 18 }
{ "emp_age" : 32, "emp_grade" : 20 }
{ "emp_age" : 45, "emp_grade" : 20 }
{ "emp_age" : 35, "emp_grade" : 22 }
This code snippet is similar to the previous one, but we use the $or
operator here. Here, the resulted documents must meet at least one condition or both.
Use $match
Aggregation With $group
in MongoDB
Example Code:
db.employee.aggregate([
{
$match:{
$or: [
{ "emp_age": {$gte:32 }},
{ "emp_grade": {$gt: 20}}
]
}
},
{
$group:{
_id: '$emp_grade',
Employees: { $sum: 1 } }
}
]);
Output:
{ _id: 18, Employees: 1 }
{ _id: 20, Employees: 2 }
{ _id: 19, Employees: 1 }
{ _id: 22, Employees: 1 }
First, we get those documents where the emp_age
is greater than or equal to 32, emp_grade
is greater than 20, or both. The document satisfying the condition(s) will also be included in the result set.
These resulted documents will be moved to the next stage, the $group
that we are using to group the documents w.r.t. the employee’s grade. In the $group
stage, we save the value of emp_grade
in _id
and the count for that specific grade in the Employees
variable.
Use $match
Aggregation With $month
and $year
in MongoDB
Example Code:
db.employee.aggregate([
{
$match: {
"emp_joindate" : {
$gte:ISODate('2012-01-01'),
$lt: ISODate('2014-12-30')
}
}
},
{
$project:{
"_id": 0,
"emp_code": 1,
"emp_age": 1,
"emp_grade": 1,
"new_created": {
"year" : {"$year" : "$emp_joindate"},
"month" : {"$month" : "$emp_joindate"}
}
}
},
{
$group:{
_id: "$new_created",
EmployeesCount:{ $sum:1 }
}
}
]);
Output:
{ _id: { year: 2012, month: 8 }, EmployeesCount: 2 }
{ _id: { year: 2014, month: 10 }, EmployeesCount: 1 }
{ _id: { year: 2014, month: 3 }, EmployeesCount: 1 }
This final code snippet contains all the concepts explained previously in this tutorial. Here, we are using three stages that are explained below.
First, we use the $match
aggregation stage to get all those documents where the emp_joindate
is greater than or equal to the 2012-01-01
and less than 2014-12-30
. As far as the ISODate()
function is concerned, it is a helper function used to wrap the native JavaScript DATE
object.
Whenever we use the ISODate()
constructor on the Mongo shell, it returns the JavaScript’s DATE
object. The resulted documents of the $match
stage are sent to the $project
stage.
At the $project
aggregation stage, we specify what fields of the documents should be returned. Also, we extract the year and month from the emp_joindate
using $year
and $month
, respectively.
Then, we save the extracted month and year in the new_created
variable. The results at this stage are passed to the $group
aggregation stage.
We group the document at the $group
stage to know how many employees were appointed in a specific month and year.