Matlab Foreach Loop
-
the
foreach
Loop Functionality -
Execute a
foreach
Loop in MATLAB Usingfor
-
Execute a
foreach
Loop in MATLAB Using awhile
Loop - Conclusion
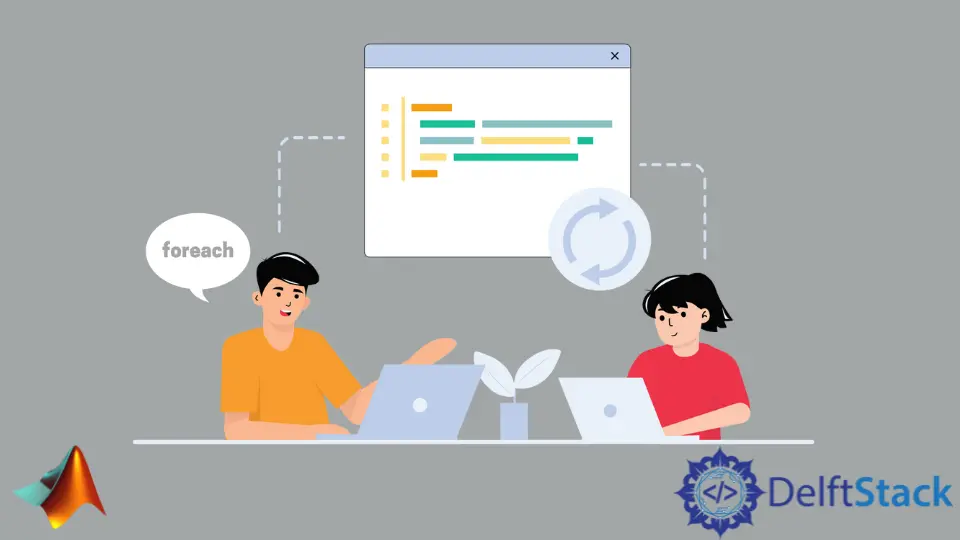
In this tutorial, we’ll explore various ways to utilize the foreach
condition to implement loops and conditions on data within MATLAB.
the foreach
Loop Functionality
To help you grasp the concept of the foreach
function in MATLAB and deepen your understanding, we’ll provide different examples along with their corresponding outputs. It’s important to note that in languages like Java and possibly others, using foreach
loops can result in undefined behavior when modifying data during iterations.
When we want to modify the data structure, we must create an Iterator
instance, allowing us to insert and remove objects from the collection. The foreach
loop in MATLAB offers an efficient, accessible, and easily readable way to handle loops.
For instance, we can effortlessly iterate through the elements of an array without the need for brackets to index the cells, providing us with more flexibility compared to hard coding.
We can streamline nested loops into a single for
-loop using the foreach
concept, enabling iteration across [M-N] array elements of any dimension. This is particularly useful in addressing various issues that arise when dealing with vectors.
By employing the foreach
concept, we can seamlessly loop through multiple vector combinations and devise our iteration algorithms for any array.
Execute a foreach
Loop in MATLAB Using for
In MATLAB, achieving a foreach
-like behavior can be accomplished using a for
loop. While MATLAB doesn’t have a built-in foreach
loop, we can utilize the for
loop to iterate over elements in a collection and perform operations on each element.
The basic syntax of a for
loop in MATLAB is as follows:
for variable = range
% This is the code to be executed in each iteration
end
variable
: This is the loop variable that will take on each value in the specifiedrange
during each iteration.range
: This specifies the range of values the loop variable will take. It could be an array, a vector, or a predefined range using the colon operatorstart:step:end
.
However, in MATLAB, the for
loop has a static nature. Unlike some programming languages where you can modify loop variables during iterations, MATLAB does not allow variable modification between iterations within the loop.
This characteristic implies that the for
loop in MATLAB is akin to a foreach
loop in terms of behavior.
To illustrate this concept, consider the following example:
%FOREACH LOOP SIMULATION IN MATLAB:
input = 5:3:25;
for output = input
% Perform operations on each element
disp(output);
end
Output:
output
5
8
11
14
17
20
23
In this example, we’ve simulated a foreach
loop by using a for
loop in MATLAB. First, we set up some sample data called input
using the colon operator, creating a sequence starting at 5
, incrementing by 3
, and stopping at 25
.
Then, we used a for
loop where we introduced a loop variable called output
. This loop goes through each element in the input
collection, and with each iteration, output
takes on the value of the current element in input
.
Inside the loop, we displayed the value of output
using the disp
function. The output we see is simply each element of the input
collection being displayed one after the other.
Essentially, it’s like a foreach
loop where we can perform actions on each item in the input
collection.
Execute a foreach
Loop in MATLAB Using a while
Loop
Another way we can achieve a foreach
-like behavior is by using a while
loop. The while
loop is a versatile construct that repeatedly executes a block of code as long as a specified condition remains true.
This characteristic makes the while
loop efficient for iterating over elements in a manner similar to a foreach
loop, especially when you need to modify data objects throughout the iterations.
In contrast to the for
loop, where the iteration count is predefined, the while
loop dynamically evaluates the condition at each iteration, allowing for potential adjustments to loop variables and conditions.
The basic syntax of a while
loop in MATLAB is as follows:
while condition
% This is the code to be executed while the condition is true
end
condition
: This is the logical expression or condition that is evaluated before each iteration.
If the condition is true, the code inside the loop will execute. If the condition becomes false, the loop exits and the program continues with the next statement after the end
keyword.
It is essential to ensure that the condition eventually becomes false; otherwise, you may end up in an infinite loop, which can cause your program to hang or crash.
To illustrate this concept, consider the following example:
%FOREACH LOOP SIMULATION IN MATLAB USING A WHILE LOOP:
input = [10, 25, 12, 6, 18];
output = zeros(size(input));
i = 1;
while i <= numel(input)
% Modify data structure during each iteration
output(i) = input(i) * 2;
i = i + 1;
end
disp('Modified data structure:')
disp(output);
Output:
Modified data structure:
20 50 24 12 36
In this MATLAB code, we first set up a sample data collection called input
, which is an array containing some predefined numbers. We also create an array called output
and set it initially to zeros, making sure it has the same size as input
.
Now, we use a while
loop, starting with a counter variable i
set to 1
. This loop keeps going as long as i
is less than or equal to the number of elements in the input
array.
Inside the loop, we modify elements of the output
array by doubling the corresponding elements in the input
array. We increment the counter i
with each iteration to process all elements in the input
array.
Once all elements have been processed, we display the modified output
array, revealing the outcome of doubling each element from the input
array. So, in the end, we get an array with each value being twice that of its respective value in the input
array.
Conclusion
In this tutorial, we explored how to utilize the foreach
condition in MATLAB-like behavior using for
and while
loops. Understanding these constructs provides flexibility in handling loops and conditions on data within MATLAB, similar to a foreach
loop in other programming languages.
Mehak is an electrical engineer, a technical content writer, a team collaborator and a digital marketing enthusiast. She loves sketching and playing table tennis. Nature is what attracts her the most.
LinkedIn