How to Remove Last Character From a String in Bash
-
Remove Last
n
Characters From a String Using Pattern Replacement in Bash -
Remove Last
n
Characters From a String Using Parameter Substitution in Bash
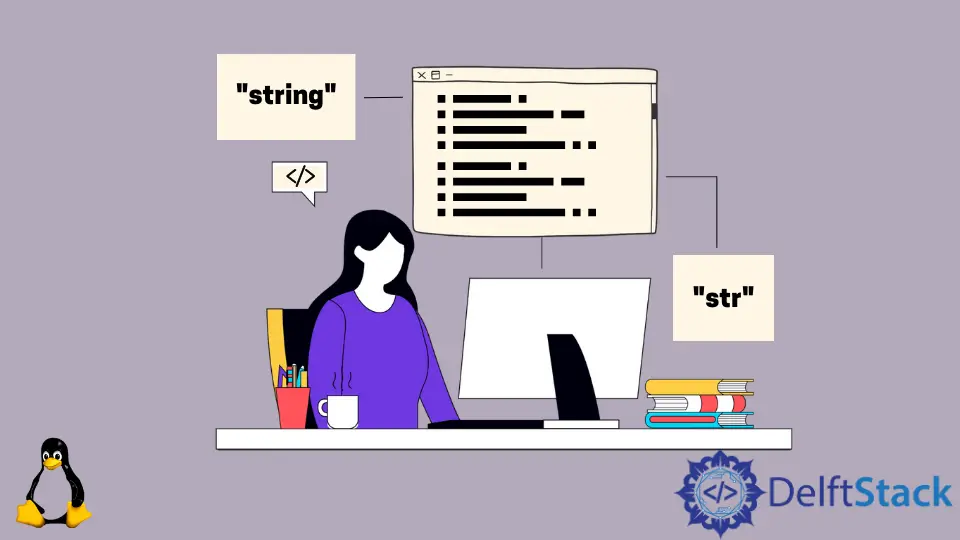
Let’s have a look at different ways of removing the last characters from a string.
Remove Last n
Characters From a String Using Pattern Replacement in Bash
To remove the last characters from a string, type the variable name followed by a %
symbol and a number of ?
symbols equal to the number of characters to be removed.
Example:
variable="verylongstring"
echo ${variable%??????}
Output:
verylong
Remove the Starting Characters
Replace the %
symbol with the #
symbol to remove characters from the starting point.
Example:
variable="verylongstring"
echo ${variable#????????}
Output:
string
Remove Last n
Characters From a String Using Parameter Substitution in Bash
The main idea of this method is to chop from the beginning of the string up to the length - number of characters to remove
. This is done by ${variable_name:starting_index:last_index}
Example:
variable="verylongstring"
length=${#variable}
echo ${variable::length-4}
Output:
verylongst
We begin by determining the string’s length. Chop the string from the beginning index, which in this case is 0 (no need to mention), and up to the length
subtracted with the number of characters to be deleted.
Related Article - Bash String
- How to Remove First Character From String in Bash
- How to Remove Newline From String in Bash
- How to Split String Into Variables in Bash
- How to Get Length of String in Bash
- How to Convert String to Integer in Bash
- String Comparison Operator in Bash