How to Remove All Classes With jQuery
-
Use the
.removeClass()
Method to Remove Classes in jQuery -
Use the
.removeAttr()
Method to Remove Classes in jQuery -
Use the
.attr()
Method to Remove Classes in jQuery -
Use the JavaScript
className
Property to Remove Classes in jQuery
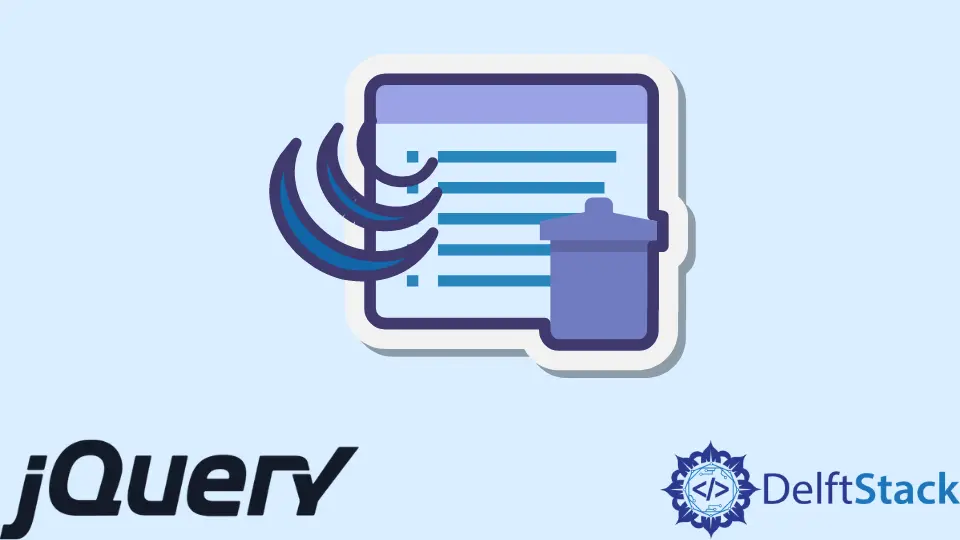
To remove all classes with jQuery, you can use three jQuery methods and one JavaScript property. The jQuery methods are .removeClass()
, .removeAttr()
, and .attr()
, while the JavaScript property is the className
property.
This article teaches you how to use them all with practical code examples.
Use the .removeClass()
Method to Remove Classes in jQuery
The .removeClass()
method allows you to remove a CSS class from an element using jQuery. But if you don’t pass it any specific class name, it’ll remove all the CSS classes from that element.
That’s what the following example is all about; you’ll remove the CSS classes from the paragraph when you press the button.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>01-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #1a1a1a;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.underline {
text-decoration: underline;
text-underline-position: under;
}
.bold {
font-weight: bold;
}
.font-size-3em {
font-size: 3em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="underline bold font-size-3em">
This paragraph has three CSS styles. Click the button above to remove them!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the removeClass method
// in jQuery.
$("main p").removeClass();
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
Output:
Use the .removeAttr()
Method to Remove Classes in jQuery
The .removeAttr()
method is specific in what it removes from an element. Here, you can pass the class
attribute from the element, and all the classes will get deleted.
In the following, we updated the jQuery code to use the .removeAttr()
to remove four CSS classes from the paragraph. You can click the newly styled button and see the results in your web browser.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>02-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #ff6347;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.italic {
font-style: italic;
}
.color-blue {
color: #1560bd;
}
.trebuchet-ms-font {
font-family: "Trebuchet MS";
}
.font-size-3em {
font-size: 3em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="italic color-blue trebuchet-ms-font font-size-3em">
Styled with CSS classes. Don't want them? Click the button above!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the removeAttr
// method in jQuery.
$("main p").removeAttr('class');
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
Output:
Use the .attr()
Method to Remove Classes in jQuery
The .attr()
method works like the .removeAttr()
method to remove CSS classes from an element. But this time, you’ll use .attr()
to set the class
property to an empty string.
The result is the removal of all classes from the matched element. The following shows you how to do it; what follows is the result in Firefox 106.0.1
.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>03-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #c069ff;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.font-style-italic {
font-style: italic;
}
.writing-mode-vertical-lr {
writing-mode: vertical-lr;
}
.font-size-2em {
font-size: 2em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="font-style-italic writing-mode-vertical-lr font-size-2em">
Weird horizontal text. Click the button to reset it to normal view!
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using the attr
// method in jQuery.
$("main p").attr('class', '');
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
Output:
Use the JavaScript className
Property to Remove Classes in jQuery
You can set the JavaScript className
property to an empty string to delete CSS classes from an element. Yes, this is a JavaScript property, but you’ll use jQuery to select the element.
The following has the updated jQuery code that will use the className
property for the removal. Also, the paragraph now has five classes that you’ll remove.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>04-jQuery-remove-all-classes</title>
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.1/jquery.min.js" integrity="sha512-aVKKRRi/Q/YV+4mjoKBsE4x3H+BkegoM/em46NNlCqNTmUYADjBbeNefNxYV7giUp0VxICtqdrbqU7iVaeZNXA==" crossorigin="anonymous" referrerpolicy="no-referrer"></script>
<style>
#btn_remove_all_classes {
padding: 1.2em;
background-color: #1560bd;
color: #ffffff;
cursor: pointer;
border-radius: 20px;
}
/* CSS styles for the paragraph that'll be
* removed using jQuery.
*/
.background-yellow {
background-color: #fed000;
}
.color-white {
color: #ffffff;
}
.text-align-center {
text-align: center;
}
.line-height-1618 {
line-height: 1.618
}
.font-size-2em {
font-size: 1.5em;
}
</style>
</head>
<body>
<main>
<button id="btn_remove_all_classes">Remove all classes from paragraph</button>
<p class="background-yellow color-white text-align-center line-height-1618 font-size-2em">
Just some random text with CSS styling.
</p>
</main>
<script>
$(document).ready(function() {
$("#btn_remove_all_classes").click(function() {
// Delete the CSS classes using JavaScript className
// property.
$("main p")[0].className = '';
// EXTRA: Remove the click event.
$("#btn_remove_all_classes").off('click');
});
});
</script>
</body>
</html>
Output:
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn