How to Copy Objects in JavaScript
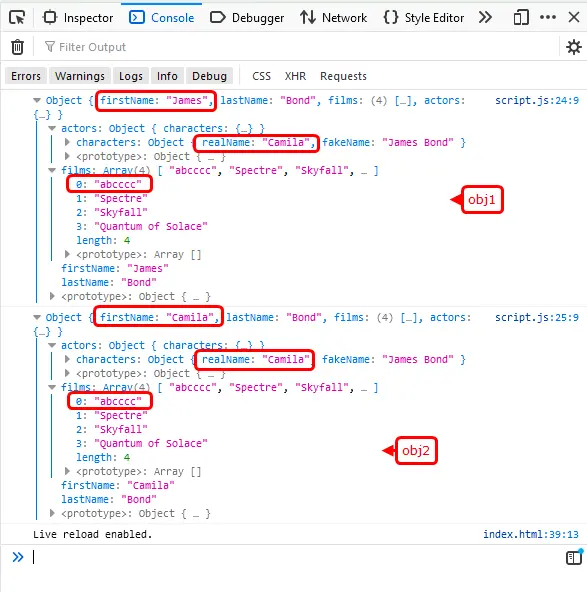
Various programming languages have various data structures that allow you to organize and store the data in memory. Each of the data structures works uniquely. For example, in C++ and Java, we have Hashmap to store the data in a key-value form. Similarly, in JavaScript, we have a data structure called an object that allows you to store the data in a key-value
format.
The objects come in handy when it comes to accessing the data. We can easily access any data within an object using its key. In this article, we will see the various ways in which we can copy objects in JavaScript.
Make a note that the JavaScript objects are mutable and are stored as a reference. If you have already created an object and would like to assign it to some other object, then that object will now hold the address of this object. Since we are storing the object’s reference inside another object, then using this new object, we can easily alter the values present inside the original object.
var a = {'name': 'Adam', 'company': 'Google'}
var b = a;
b.name = 'Sam'
console.log(a.name, b.name);
Output:
Sam Sam
As you can see from the above example, assigning one object to another will not copy the object itself. It will only store the address of the old object into the new object.
There are two ways of copying an object in JavaScript. One way is called shallow copying, and another way is called deep copying. To implement these ways, we can make use of some JavaScript methods as follows.
Shallow Copy an Object in JavaScript
In shallow copy, only the key-value
pairs present at the first level of the object will be copied to the new object. And all the nested elements or properties, like an array or another object inside, will not be copied, and instead, their reference will be stored in this object.
In shallow copy, some of the properties of the original object also get affected. To understand this, please see the below example.
var obj1 = {
'firstName': 'James',
'lastName': 'Bond',
'films': ['No Time To Die', 'Spectre', 'Skyfall', 'Quantum of Solace'],
'actors':
{'characters': {'realName': 'Daniel Craig', 'fakeName': 'James Bond'}}
}
In this example, we have an object called obj1
. To shallow copy this object using JavaScript, we can use Object.assign()
and spread operator ...
. Let’s perform shallow copy on the obj1
using the below methods in JavaScript.
Object.assign()
Method
The Object.assign()
method takes two parameters. The first parameter is the target object which will be returned after the copying is done. The second parameter is the object that we want to copy: obj1
. In our case, we will pass an empty object {}
as the target. This is because all the elements inside the obj1
will be copied to this empty object. After the copying is done, the Object.assign()
will return the new object that we will store inside the obj2
variable.
var obj2 = Object.assign({}, obj1);
Later, we will modify the properties of this object, and we will see practically why this is called a shallow copy.
obj2.firstName = 'Camila';
obj2.films[0] = 'abcccc';
obj2.actors.characters.realName = 'Camila';
console.log(obj1);
console.log(obj2);
Output:
Here, we change three values using the obj2, firstName
, the first film from the films[]
array, and the realName
property from the actors.characters
object. Then we are printing both the objects.
The image above shows that only the firstName
property is not changed in both the objects. The other two properties, films[]
and realName
are changed in both the objects. This is called shallow copy as the firstName
property is unique, whereas the other properties are common for both the objects as we are storing the address here. Here, properties present at the first level are only copied.
- Spread operator (
...
)
The rest operator will copy the properties of the obj1
into obj2
. This is similar to Object.assign()
, where we shallow copy the properties of the objects. To copy an object using the spread operator, you have to write the object name after the spread symbol.
var obj2 = {...obj1};
obj2.firstName = 'Camila';
obj2.films[0] = 'abcccc';
obj2.actors.characters.realName = 'Camila';
You will also get the same output that we got after using the Object.assign()
method.
Deep Copy an Object in JavaScript
In a deep copy, all the key-value
pairs will be copied to the new object. To perform deep copy, we can use JSON.parse()
and JSON.stringify()
methods. Note that the deep copy will not copy the properties present inside the prototype object.
Using the JSON.stringify()
, we will first convert the entire object, in this case obj1
, into a string, and then later, with the help of the JSON.parse()
method, we will parse this string back into the JSON format.
let obj2 = JSON.parse(JSON.stringify(obj1));
Here, you can modify any properties inside the obj2
, which will not affect the properties inside the obj1
.
obj2.firstName = 'Camila';
obj2.films[0] = 'abcccc';
obj2.actors.characters.realName = 'Camila';
Output:
We are modifying the same values that we were previously modifying, and now you can notice that when we are changing the properties of the obj2
, it is not affecting the properties of the obj1
.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn