How to Play a Video Using JavaFX
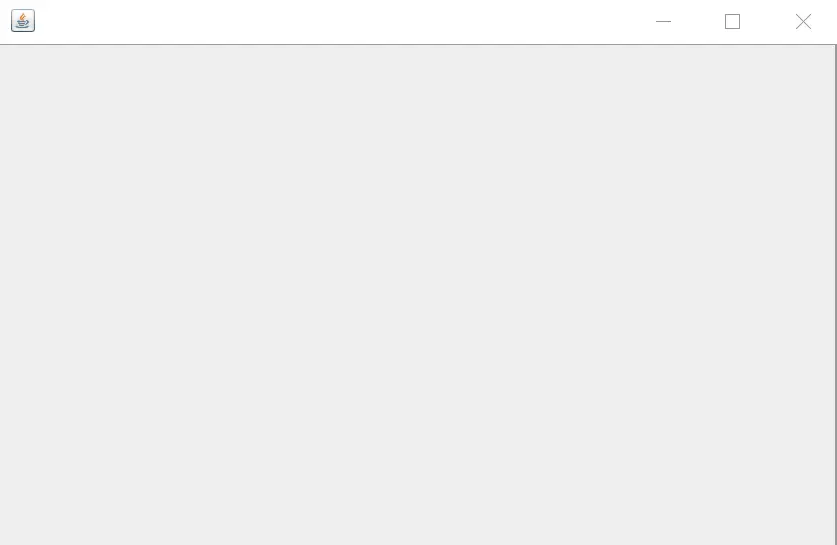
This article will teach us how to make a simple video player in Java.
Here we’ll use an open-source Java-based framework JavaFX
(stands for special effects in Java), which provides a collection of graphics and media packages that contains classes like Media
, MediaPlayer
, MediaView
, and AudioClip
that are used to develop Desktop applications and Rich Internet Application (RIA) which can run across various platforms.
There are some prerequisites to using JavaFX to play a video.
JavaFX
should be installed.SceneBuilder
should be installed.
Play a Video Using JavaFX
To play a video using JavaFX, we’ll use Media
, MediaPlayer
and MediaView
classes. We must follow the steps below to play the video files using JavaFX.
-
Create an object of the
File
class with the location/path of our video file.File path = new File("D://movie.mp4")
-
Create the object of the
Media
class with the file path as its argument to its constructor.Media obj = new Media(path)
-
Create the object of the
MediaPlayer
class and pass theMedia
class created above as its argument to its constructor.Mediaplayer player = new MediaPlayer(obj);
-
Create the object of the
MediaView
class and pass theMediaPlayer
class object created above as its argument to its constructor.
```java
MediaView view = new MediaView(player)
```
-
The last step is configuring the
Scene
and passing theMediaView
object as the argument to thePane
class constructor.Scene scene = new Scene(new Pane(view), 1024, 800); primaryStage.setScene(scene); primaryStage.show();
Full code:
import java.io.*;
import javafx.application.*import javafx.scene.Scene;
import javafx.scene.layout.Pane;
import javafx.scene.media.*;
import javafx.stage.Stage;
public class test extends Application {
public void start(Stage primaryStage) {
File path = new File("" D :\\movie.mp4 "");
Media obj = new Media(path.toURI().toURL().toString());
MediaPlayer player = new MediaPlayer(obj);
MediaView mediaView = new MediaView(player);
Scene scene = new Scene(new Pane(mediaView), 500, 400);
primaryStage.setScene(scene);
primaryStage.show();
mediaPlayer.play();
public static void main(String[] args) {
launch(args);
}
}
Output:
The video will play very slowly; below is the starting screenshot of the player.