How to Convert String to Boolean in Java
-
Convert a String to
boolean
orBoolean
UsingBoolean.parseBoolean(string)
in Java -
Convert a String to
boolean
orBoolean
UsingBoolean.valueOf(string)
in Java -
Convert a String to
boolean
andBoolean
UsingBooleanUtils.toBoolean(string)
in Java
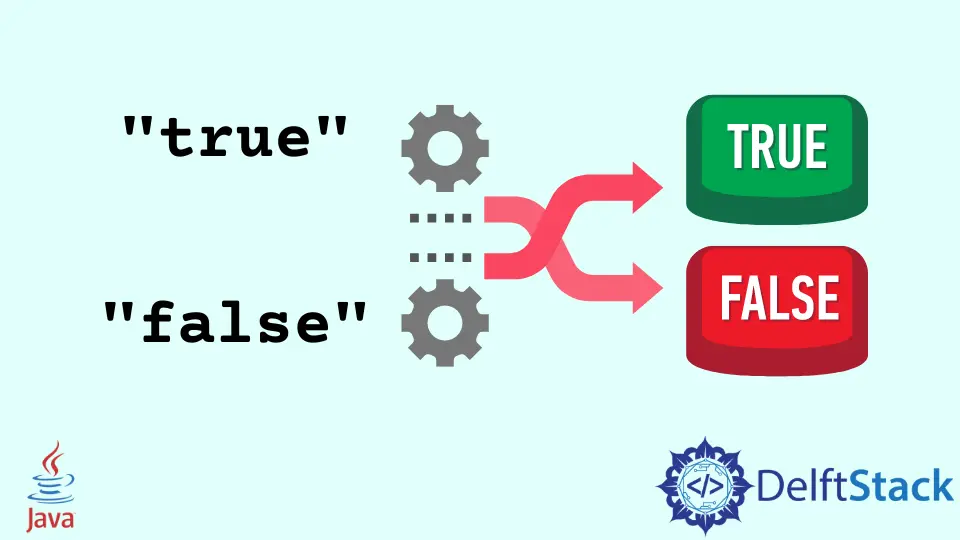
We have introduced how to convert boolean to string in Java in another article. Today, we will look at three methods that help us to convert a Java string to a boolean. We can use a boolean value with a primitive boolean
or Boolean
object. Although the Boolean
class wraps the primitive boolean
, we will see the cases of both in the following examples.
Convert a String to boolean
or Boolean
Using Boolean.parseBoolean(string)
in Java
The first example uses the parseBoolean(string)
method of the Boolean
class. It directly parses or converts a given string to return a primitive boolean
representing the string. One important thing to note is that this method will return false
when a string value other than true
or false
is passed.
As we know that parseBoolean()
returns a boolean
, we can use the returned value as a boolean bool
and Boolean boolObj
because the Boolean
class has the primitive boolean
in it.
public class StringToBoolean {
public static void main(String[] args) {
String exampleString = "false";
boolean bool = Boolean.parseBoolean(exampleString);
Boolean boolObj = Boolean.parseBoolean(exampleString);
System.out.println("Primitive boolean: " + bool);
System.out.println("Boolean object: " + boolObj);
}
}
Output:
Primitive boolean: false
Boolean object: false
Convert a String to boolean
or Boolean
Using Boolean.valueOf(string)
in Java
Another static function of the Boolean
class to convert a string to boolean is valueOf()
. It takes the string as an argument and returns a Boolean
value that represents the string. Below we can see that exampleString
is initialized with true
as a string, and we pass it to the valueOf(string)
method.
public class StringToBoolean {
public static void main(String[] args) {
String exampleString = "true";
boolean bool = Boolean.valueOf(exampleString);
Boolean boolObj = Boolean.valueOf(exampleString);
System.out.println("Primitive boolean: " + bool);
System.out.println("Boolean object: " + boolObj);
}
}
Output:
Primitive boolean: true
Boolean object: true
Convert a String to boolean
and Boolean
Using BooleanUtils.toBoolean(string)
in Java
In both above methods, functions always return false
if the string value is anything other than true
or false
. This issue can be solved using the BooleanUtils.toBoolean()
method of the apache common library.
We use the following dependency in our project to include the library.
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.11</version>
</dependency>
In the following example, we have three strings: yes
, no
, and true
. And to convert all these values to a boolean, we will use BooleanUtils.toBoolean(string)
and pass the string as an argument. In the output, we can see that all the values were parsed correctly. yes
is converted to true
, and no
is converted to false
.
import org.apache.commons.lang3.BooleanUtils;
public class StringToBoolean {
public static void main(String[] args) {
String exampleString = "yes";
String exampleString1 = "no";
String exampleString2 = "true";
boolean bool1 = BooleanUtils.toBoolean(exampleString);
Boolean boolObj1 = BooleanUtils.toBoolean(exampleString);
boolean bool2 = BooleanUtils.toBoolean(exampleString1);
Boolean boolObj2 = BooleanUtils.toBoolean(exampleString1);
boolean bool3 = BooleanUtils.toBoolean(exampleString2);
Boolean boolObj3 = BooleanUtils.toBoolean(exampleString2);
System.out.println("Primitive boolean 1: " + bool1);
System.out.println("Boolean object 1: " + boolObj1);
System.out.println("Primitive boolean 2: " + bool2);
System.out.println("Boolean object 2: " + boolObj2);
System.out.println("Primitive boolean 3 : " + bool3);
System.out.println("Boolean object 3: " + boolObj3);
}
}
Output:
Primitive boolean 1: true
Boolean object 1: true
Primitive boolean 2: false
Boolean object 2: false
Primitive boolean 3 : true
Boolean object 3: true
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedInRelated Article - Java Boolean
- How to Toggle a Boolean Variable in Java
- How to Print Boolean Value Using the printf() Method in Java
- How to Initialise Boolean Variable in Java
- How to Convert Boolean to Int in Java
- How to Convert Boolean to String in Java