How to Create Java Inline Function
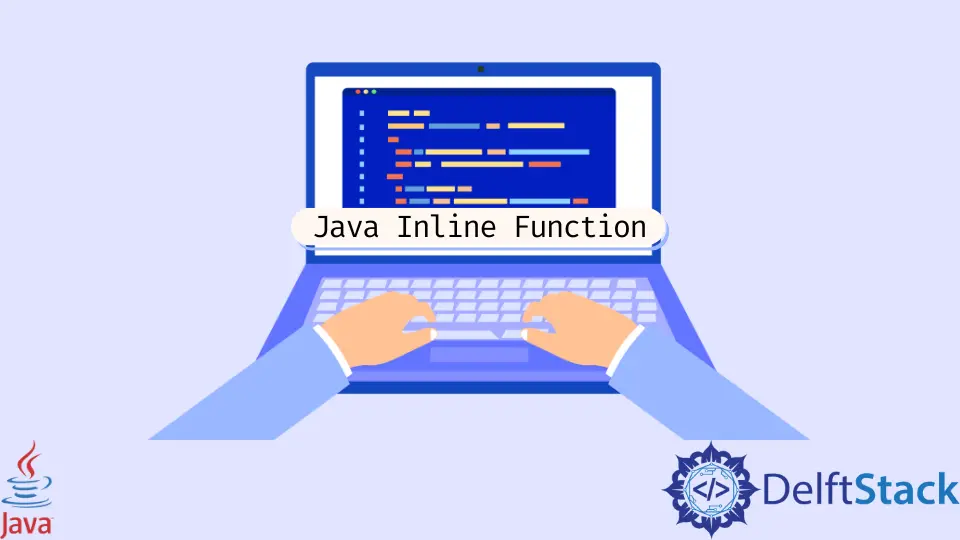
This tutorial demonstrates how to implement an inline function in Java.
Java Inline Function
When the compiler copies the code of a function and places it anywhere, it is considered an inline function. If we change the code of an inline function, the compiler would have to change the code in all the copied versions.
The HotSpot JVM just-in-time (JIT) compiler can perform a lot of optimizations which also include inlining, which is enabled by default, and we can use the following flags to enable or disable the inlining in JVM:
-XX:+Inline (+ means true, which enables the inlining)
-XX:-Inline (- means false, which disables the inlining)
Java doesn’t provide inline functions, but the JVM performs this operation at the execution time. As the compiler performs, we don’t have to use the inline function in Java.
To understand how it works, let’s try to create the inline function functionality in Java. See the example:
package delftstack;
public class Inline_Example {
public static final class Points {
private int a = 0;
private int b = 0;
public int getA() {
return a;
}
public void setA(int a) {
this.a = a;
}
public int getB() {
return b;
}
public void setB(int b) {
this.b = b;
}
@Override
public String toString() {
return String.format("%s[a=%d, b=%d]", getClass().getSimpleName(), a, b);
}
}
public static void main(String[] args) {
final Points NotInlinedPoints = new Points();
NotInlinedPoints.setA(NotInlinedPoints.getA() + 2);
NotInlinedPoints.setB(NotInlinedPoints.getA() * 6 / 2);
final Points InlinedPoints = new Points();
InlinedPoints.a = InlinedPoints.a + 2;
InlinedPoints.b = InlinedPoints.a * 6 / 2;
System.out.println("The Non Inlined Points " + NotInlinedPoints);
System.out.println("The Inlined Points " + InlinedPoints);
}
}
The code above shows the method inlining. The optimization is performed, and the method call is replaced with simple statements, as shown in our code.
Both NotInlinedPoints
and InlinedPoints
are performing the same operation, as shown in the output:
The Non Inlined Points Points[a=2, b=6]
The Inlined Points Points[a=2, b=6]
As we can see here, we don’t have to perform the inlining by declaring an inline function in Java. The JVM does it with some complex structure behind the scene.
By default, the inlining is enabled in JVM. Let’s try to run the code by disabling the inlining by using the above flags.
Run the above code in cmd:
C:\Users\DelftStack>javac Inline_Example.java
C:\Users\DelftStack> Java -XX:-Inline Inline_Example.java
The Non Inlined Points Points[a=2, b=6]
The Inlined Points Points[a=2, b=6]
As we can see, the code doesn’t show any difference in the result after disabling the inlining, but the process ids and the time elapsed will be more for this compilation.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook