How to Convert String Array Into Int Array in Java
-
Convert
string
Array toint
Array by Using theparseInt()
Method in Java -
Convert
string
Array toint
Array by Using theStream
API in Java -
Convert
string
Array toint
Array by Using theStream
API in Java
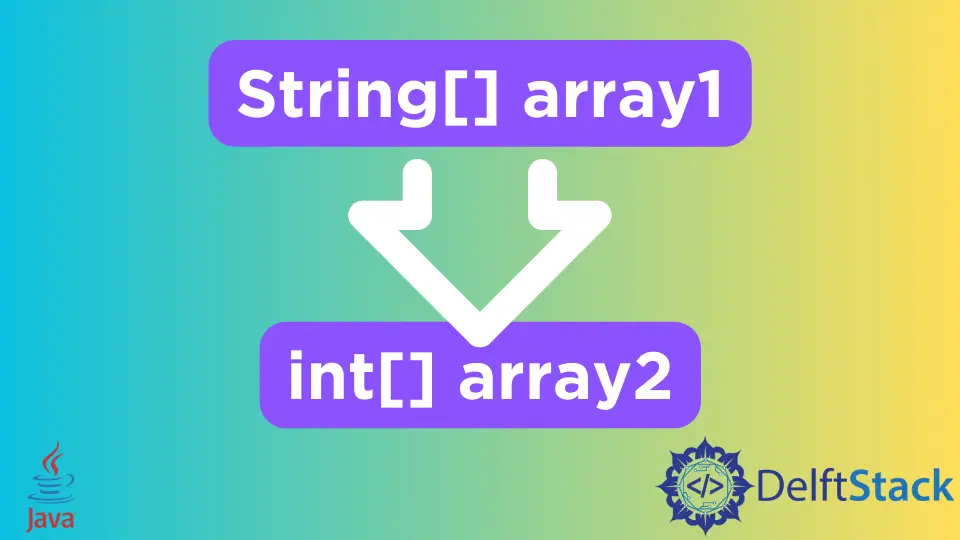
This tutorial introduces how to convert string
arrays to int
arrays in Java. You’ll see some example programs so that you can understand this concept better.
An array that holds string values is a string
array; similarly, an int
array contains only integer values. In this article, you’ll learn how to convert a string array into an int array by using some built-in methods in Java, such as the parseInt()
function and the Stream
API.
Convert string
Array to int
Array by Using the parseInt()
Method in Java
In this example, we use the parseInt()
method of the Integer
class that returns the integer after the conversion. Since it works with a single value, we use a for
loop to convert all the elements of the string
array into int
and assign them to an int
array simultaneously. We used the second loop to check if the conversion taking place is fine. See the example below:
public class SimpleTesting {
public static void main(String[] args) {
String[] arr = new String[] {"2", "34", "55"};
int[] arr2 = new int[3];
for (int i = 0; i < arr.length; i++) {
arr2[i] = Integer.parseInt(arr[i]);
}
for (int i = 0; i < arr2.length; i++) {
System.out.println(arr2[i]);
}
}
}
Output:
2
34
55
Convert string
Array to int
Array by Using the Stream
API in Java
If you’re working with Java 8 or a higher version and familiar with the Stream
API, you can use the code below. In this example, we used the toArray()
method, which returns an integer array. Here’s the sample program:
import java.util.stream.Stream;
public class SimpleTesting {
public static void main(String[] args) {
String[] arr = new String[] {"2", "34", "55"};
Integer[] arr2 = Stream.of(arr).mapToInt(Integer::parseInt).boxed().toArray(Integer[] ::new);
for (int i = 0; i < arr2.length; i++) {
System.out.println(arr2[i]);
}
}
}
Output:
2
34
55
Convert string
Array to int
Array by Using the Stream
API in Java
This example is similar to the code block above, except that it returns an array of the primitive int
value. The previous example returns an array of integers(wrapper class).
import java.util.Arrays;
public class SimpleTesting {
public static void main(String[] args) {
String[] arr = new String[] {"2", "34", "55"};
int[] arr2 = Arrays.stream(arr).mapToInt(Integer::parseInt).toArray();
for (int i = 0; i < arr2.length; i++) {
System.out.println(arr2[i]);
}
}
}
Output:
2
34
55
Related Article - Java Array
- How to Concatenate Two Arrays in Java
- How to Convert Byte Array in Hex String in Java
- How to Remove Duplicates From Array in Java
- How to Count Repeated Elements in an Array in Java
- Natural Ordering in Java
- How to Slice an Array in Java