How to Fixe Error: Java Char Cannot Be Dereferenced
-
Reasons for
Char Cannot Be Derefenced
Error -
Methods to Solve the
Char Cannot be Dereferenced
Error - Conclusion
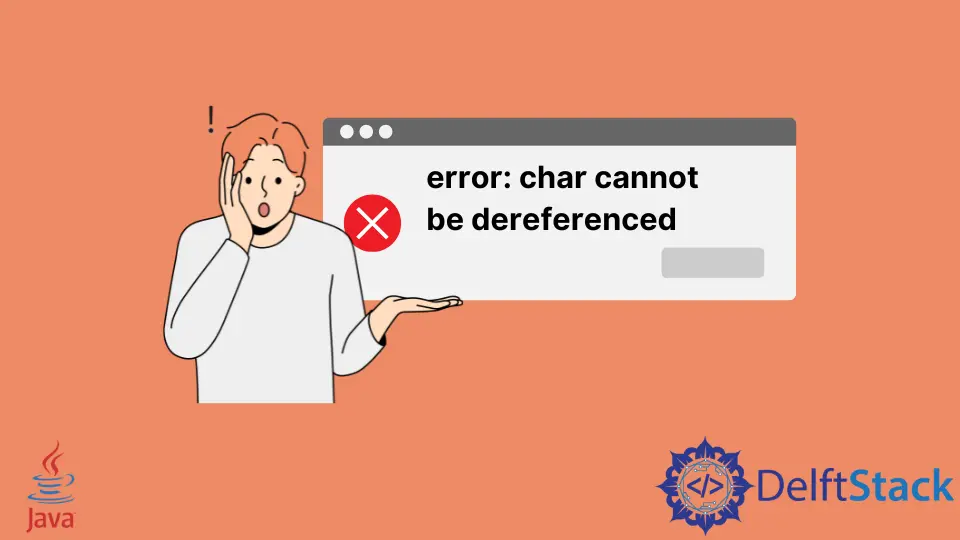
This tutorial demonstrates how to solve Java’s java char cannot be dereferenced
error.
Reasons for Char Cannot Be Derefenced
Error
The error char cannot be dereferenced
occurs in Java when you attempt to invoke a method or access a field on a primitive char
type as if it were an object. This happens because primitive types, including char
, do not have methods or fields like objects.
Attempting to Call a Method on a char
Primitive
Attempting to call a method on a char
primitive in Java results in the char cannot be dereferenced
error because primitive types lack the structure of objects. Unlike objects, primitives, including char
, do not have associated methods or fields.
As a result, treating a char
as an object and trying to invoke methods on it leads to this compilation error.
Using a Dot Notation on a char
Primitive
Using dot notation on a char
primitive results in the char cannot be dereferenced
error in Java because primitive types lack the properties and methods associated with objects. Dot notation is used to access members of objects, and since char
is a primitive, attempting to use dot notation implies treating it as an object, which is not valid for primitive types, leading to this compilation error.
Assuming char
Is an Object
Assuming a char
is an object results in the char cannot be dereferenced
error in Java because, although autoboxing may convert it to a Character
object, it remains a primitive. Attempting to access methods on this assumed object leads to the error, as primitives lack the structure of objects.
Trying to Access a Field on a char
Trying to access a field on a char
results in the char cannot be dereferenced
error in Java because primitive types, including char
, lack fields or properties. Unlike objects, which can have associated fields, primitives do not support direct access to fields.
This attempt to access a field on a char
as if it were an object leads to a compilation error.
Mixing Up char
and String
Operations
Mixing up char
and String
operations results in the char cannot be dereferenced
error in Java because primitive char
lacks the methods available for String
objects. Trying to call String-specific methods on a char
primitive confuses the compiler, leading to this compilation error.
Methods to Solve the Char Cannot be Dereferenced
Error
Wrapper Class
Using a wrapper class like Character
in Java allows treating primitive char
values as objects, providing access to useful methods and resolving limitations associated with direct manipulation of primitive types. The use of wrapper classes provides a convenient solution without sacrificing the efficiency of primitive types.
Code Example:
public class CharDereferenceExample {
public static void main(String[] args) {
char myChar = 'A';
// Using Character wrapper class to overcome "char cannot be dereferenced" error
Character charWrapper = Character.valueOf(myChar);
// Call methods on the Character wrapper
char convertedChar = charWrapper.charValue();
System.out.println("Original char: " + myChar);
System.out.println("Converted char: " + convertedChar);
}
}
In this code breakdown, we first declare a primitive char
variable, myChar
, initializing it with the character A
. To overcome the char cannot be dereferenced
error, we then create an instance of the Character
wrapper class using the valueOf
method, where the primitive char myChar
is passed as an argument.
With the resulting Character
object (charWrapper
), we proceed to dereference it by calling the charValue()
method, allowing us to retrieve the underlying char value. Finally, we print both the original char value (myChar
) and the converted char value (convertedChar
) to the console, resolving the dereferencing error using the Character
wrapper class.
Output:
The output demonstrates that we successfully converted and dereferenced the char
value using the Character
wrapper class, resolving the initial issue.
String Conversion
String conversion in Java transforms primitive char
values into strings, enabling seamless access to string methods and overcoming restrictions associated with direct manipulation of primitive types. This method provides an alternative solution to the use of wrapper classes, offering flexibility in handling character data.
Code Example:
public class CharToStringExample {
public static void main(String[] args) {
char myChar = 'B';
// Convert char to String to overcome "char cannot be dereferenced" error
String charAsString = String.valueOf(myChar);
// Call methods on the String representation of the char
int charLength = charAsString.length();
char charAtZero = charAsString.charAt(0);
System.out.println("Original char: " + myChar);
System.out.println("String representation: " + charAsString);
System.out.println("Length of the string: " + charLength);
System.out.println("Character at index 0: " + charAtZero);
}
}
In this code explanation, we begin by declaring and initializing a primitive char
variable, myChar
, set to the character B
. To address the char cannot be dereferenced
error, we then convert the primitive char
to a String
using the valueOf
method from the String
class, which takes the char
as input and returns its string representation.
Subsequently, with the obtained String
representation (charAsString
), we treat it as an object and invoke methods on it, utilizing length()
to retrieve the string length and charAt(0)
to obtain the character at index 0. Finally, we print the original char
value, the string representation, the string length, and the character at index 0 to the console.
Output:
The output illustrates that we successfully converted the char
to a String and performed operations on it without encountering the dereferencing error.
Direct Comparison Method
By comparing primitive char
values directly, developers avoid the need for complex conversions or wrapper classes, ensuring a straightforward and efficient solution to the dereferencing issue.
Directly comparing primitive char
values provides a simple and effective solution to the char cannot be dereferenced
error. By bypassing the need for wrapper classes or conversions, this method allows developers to perform comparisons and boolean
operations directly on the primitive data type, maintaining code clarity and efficiency.
Code Example:
public class CharComparisonExample {
public static void main(String[] args) {
char firstChar = 'C';
char secondChar = 'C';
// Directly compare chars to overcome "char cannot be dereferenced" error
boolean areCharsEqual = (firstChar == secondChar);
System.out.println("First char: " + firstChar);
System.out.println("Second char: " + secondChar);
System.out.println("Are chars equal? " + areCharsEqual);
}
}
In examining this code, we start by declaring and initializing two primitive char
variables, namely firstChar
and secondChar
, both set to the character C
. To address the char cannot be dereferenced
error, we directly compare these two char
values using the equality operator (==
).
The comparison assesses whether the characters are equal, and the result is stored in the boolean
variable areCharsEqual
. Subsequently, we print the original values of firstChar
and secondChar
, along with the outcome of the direct comparison, to the console.
Output:
The output demonstrates that the direct comparison of firstChar
and secondChar
using the equality operator successfully overcomes the dereferencing error, indicating that the two characters are indeed equal.
Conclusion
Understanding the char cannot be dereferenced
error is crucial for Java developers. By exploring methods such as using wrapper classes, string conversion, and direct comparison, we have versatile tools to address this issue efficiently.
Whether choosing the simplicity of direct comparisons, leveraging the functionality of wrapper classes, or employing string conversion, developers can navigate and resolve the challenge, ensuring smooth and error-free handling of primitive char
types in Java.
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Error
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix the Missing Server JVM Error in Java
- How to Fix the 'No Java Virtual Machine Was Found' Error in Eclipse
- How to Fix Javax.Net.SSL.SSLHandShakeException: Remote Host Closed Connection During Handshake
- How to Fix the Error: Failed to Create the Java Virtual Machine
- How to Fix Java.Lang.VerifyError: Bad Type on Operand Stack