How to Center a JLabel in Swing
Sheeraz Gul
Feb 02, 2024
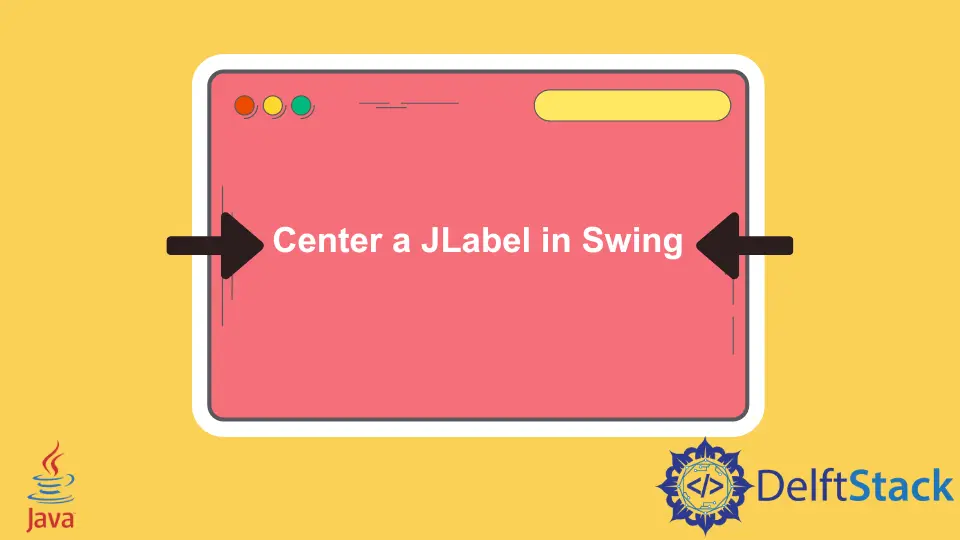
This tutorial demonstrates how to center a JLabel
in Java Swing.
Center a JLabel
in Swing
We can use the LayoutManager GridBagLayout
to center the components in Swing. Similarly, we can center a JLabel
using GridBagLayout
.
Follow the steps below to center a JLabel
in Java Swing.
-
First of all, create a
JFrame
. -
Then, create a
JPanel
. -
Now, create the
JFrame
, which will be centered. -
Set layout in the
JPanel
asGridBagLayout
. -
Set the borders of
JPanel
. -
Finally, get it done with the closing of
JFrame
.
Let’s try an example in Java based on the steps above.
package delftstack;
import java.awt.GridBagLayout;
import javax.swing.BorderFactory;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JTextArea;
import javax.swing.WindowConstants;
public class Center_Jlabel {
public static void main(String[] args) {
JFrame J_Frame = new JFrame("Center Frame");
JPanel J_Panel = new JPanel();
JLabel J_Label = new JLabel("Centered Label for Name Field: ");
JTextArea text = new JTextArea();
text.setText("Add Name");
J_Panel.setLayout(new GridBagLayout());
J_Panel.add(J_Label);
J_Panel.add(text);
J_Panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
J_Frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
J_Frame.add(J_Panel);
J_Frame.setSize(700, 300);
J_Frame.setVisible(true);
}
}
The code above will center a JLabel
using Java Swing. See output:
Author: Sheeraz Gul
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn FacebookRelated Article - Java Swing
- How to Clear Text Field in Java
- How to Create Canvas Using Java Swing
- How to Use of SwingUtilities.invokeLater() in Java
- How to Change the JLabel Text in Java Swing
- Java Swing Date