How to Call Webservices in Java
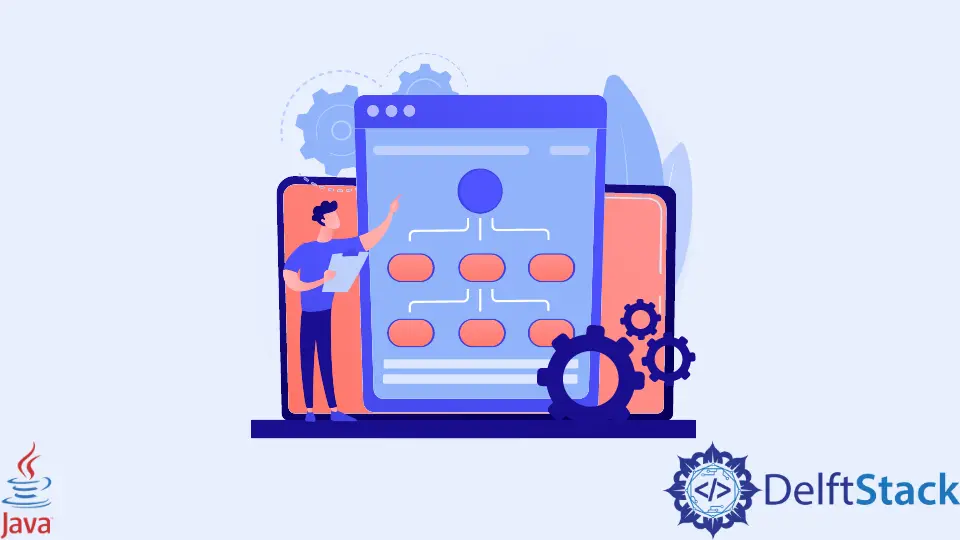
This article will teach us how to do a SOAP
Web Service call from a Java class.
SOAP
Web Services
The SOAP
stands for Simple Object Access Protocol
. Web services may be accessed via this XML
-based interface.
The W3C
recommends SOAP
for communication between two apps. The SOAP
protocol is XML-based.
It has no preference for any particular platform or language. You may communicate with other programming language apps by utilizing SOAP
.
SOAP
Web Service Call From a Java Class
In some situations, it may be quicker and more helpful to utilize Java classes rather than the web service
library to invoke the SOAP
service. For instance, you can run into issues when using a web service
library to generate a client proxy or if you want a few small, specialized portions of the response.
It is simply a SOAP
request via the HTTP
or HTTPS
protocol from a simple piece of Java code without utilizing any Java libraries.
These are the following steps to call SOAP Web service
from a Java class.
the WSDL
First, open the WSDL
file to make a SOAP
web service call from a Java class. Check for the XSD
, SOAP Operation
, and SOAP address
locations in the WSDL
file.
The SOAP
requests and responses have the names getUserDetailsRequest
and getUserDetailsResponse
, respectively. Additionally, there is the request’s input parameter(s).
Only one argument is present here, name
, and it is a string
.
...
<xs:element name="getUserDetailsRequest">
<xs:complexType>
<xs:sequence>
<xs:element name="name" type="xs:string" />
</xs:sequence>
</xs:complexType>
</xs:element>
<xs:element name="getUserDetailsResponse">
<xs:complexType>
<xs:sequence>
<xs:element name="users" type="tns:user" minOccurs="0"
maxOccurs="unbounded" />
</xs:sequence>
</xs:complexType>
</xs:element>
...
Request XML
Java code is not required to create the response XML
structure since we will get it from the server. However, Java code is required to create the request XML
structure because we must submit it as input to the SOAP service
.
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/">
<soapenv:Header/>
<soapenv:Body>
<getUserDetailsRequest xmlns="https://roytuts.com/UserService">
<name>User Name</name>
</getUserDetailsRequest>
</soapenv:Body>
</soapenv:Envelope>
SOAP
Address
Find the SOAP
address location, which serves as the SOAP
service’s endpoint URL. We will connect to this endpoint using Java’s HttpURLConnection
API.
SOAP
Operation
The operation name, which will be utilized as a SOAP
action, must then be located. getUserDetails
is located here.
Create SOAP
Client
We have determined all the necessities and are prepared to develop the custom Java class.
package com.roytuts.soap.client;
import java.io.BufferedReader;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStream;
import java.io.StringReader;
import java.io.StringWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLConnection;
import java.nio.charset.Charset;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import javax.xml.transform.OutputKeys;
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.TransformerFactory;
import javax.xml.transform.dom.DOMSource;
import javax.xml.transform.stream.StreamResult;
import org.w3c.dom.Document;
import org.xml.sax.InputSource;
import org.xml.sax.SAXException;
public class SoapClient {
public static void main(String[] args) throws IOException {
// Webservice HTTP request configurations
String wsEndPoint = "http://localhost:9999/ws";
URL url = new URL(wsEndPoint);
URLConnection connection = url.openConnection();
HttpURLConnection http = (HttpURLConnection) connection;
ByteArrayOutputStream bout = new ByteArrayOutputStream();
String xmlInput = "<soapenv:Envelope xmlns:soapenv=" http
: // schemas.xmlsoap.org/soap/envelope/"><soapenv:Header/><soapenv:Body><getUserDetailsRequest
// xmlns="https://roytuts.com/UserService"><name>User
// Name</name></getUserDetailsRequest></soapenv:Body></soapenv:Envelope>";
byte[] buffer = new byte[xmlInput.length()];
buffer = xmlInput.getBytes();
bout.write(buffer);
byte[] b = bout.toByteArray();
String stringResponse = "";
String stringOutput = "";
String SOAPAction = "getUserDetails";
http.setRequestProperty("Content-Length", String.valueOf(b.length));
http.setRequestProperty("Content-Type", "text/xml; charset=utf-8");
http.setRequestProperty("SOAPAction", SOAPAction);
http.setRequestMethod("POST");
http.setDoOutput(true);
http.setDoInput(true);
OutputStream out = http.getOutputStream();
out.write(b);
out.close();
InputStreamReader isr = new InputStreamReader(http.getInputStream(), Charset.forName("UTF-8"));
BufferedReader in = new BufferedReader(isr);
while ((stringResponse = in.readLine()) != null) {
stringOutput = stringOutput + stringResponse;
}
String formattedSOAPResponse = formatXML(stringOutput);
System.out.println(formattedSOAPResponse);
}
private static String formatXML(String unformattedXml) {
try {
Document document = parseXmlFile(unformattedXml);
TransformerFactory transformerFactory = TransformerFactory.newInstance();
transformerFactory.setAttribute("indent-number", 3);
Transformer transformer = transformerFactory.newTransformer();
transformer.setOutputProperty(OutputKeys.INDENT, "yes");
DOMSource source = new DOMSource(document);
StreamResult xmlOutput = new StreamResult(new StringWriter());
transformer.transform(source, xmlOutput);
return xmlOutput.getWriter().toString();
} catch (TransformerException e) {
throw new RuntimeException(e);
}
}
private static Document parseXmlFile(String in) {
try {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
DocumentBuilder db = dbf.newDocumentBuilder();
InputSource is = new InputSource(new StringReader(in));
return db.parse(is);
} catch (IOException | ParserConfigurationException | SAXException e) {
throw new RuntimeException(e);
}
}
}
HTTPS Call
Use the idea in the code sample below to call a SOAP
web service over an HTTPS
connection. This example demonstrates how to contact a SOAP
web service from an ordinary Java code using the GET
and POST
methods.
The below code serves as an illustration of how to use the HTTPS
protocol. Therefore, attempt to consider all programming standards while developing the code for your application.
package com.roytuts.java.soap.https.connection;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.net.HttpURLConnection;
import java.net.URL;
import javax.net.ssl.HttpsURLConnection;
public class JavaSoapHttpsClient {
public static void main(String[] args) throws Exception {
invokeSoapService(
"https://postman-echo.com/post", "POST", "<xml><body>SAOP request</body></xml>");
invokeSoapService("https://www.google.com", "GET", null);
}
public static void invokeSoapService(
final String url, final String httpMethod, final String requestXML) throws IOException {
URL myUrl = new URL(url);
HttpURLConnection http = (HttpsURLConnection) myUrl.openConnection();
http.setDoOutput(true);
http.setRequestMethod(httpMethod);
OutputStreamWriter out = null;
if ("POST".equals(httpMethod) && requestXML != null) {
out = new OutputStreamWriter(conn.getOutputStream());
out.append(requestXML);
}
BufferedReader br = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String inputLine;
System.out.println("Response Code: " + conn.getResponseCode());
System.out.print("Response Text: ");
while ((inputLine = br.readLine()) != null) {
System.out.println(inputLine);
}
if (out != null) {
out.flush();
out.close();
}
br.close();
}
}
This article taught us how to do a SOAP
web service call from a Java class. Various steps are discussed in this article.
Users can follow the steps to call a SOAP
web service call from the Java class.