How to Initialize 2D Array in Java
-
Initialize 2D Array in Java Using the
new
Keyword and thefor
Loop - Initialize 2D Array in Java Without Using the Initializer
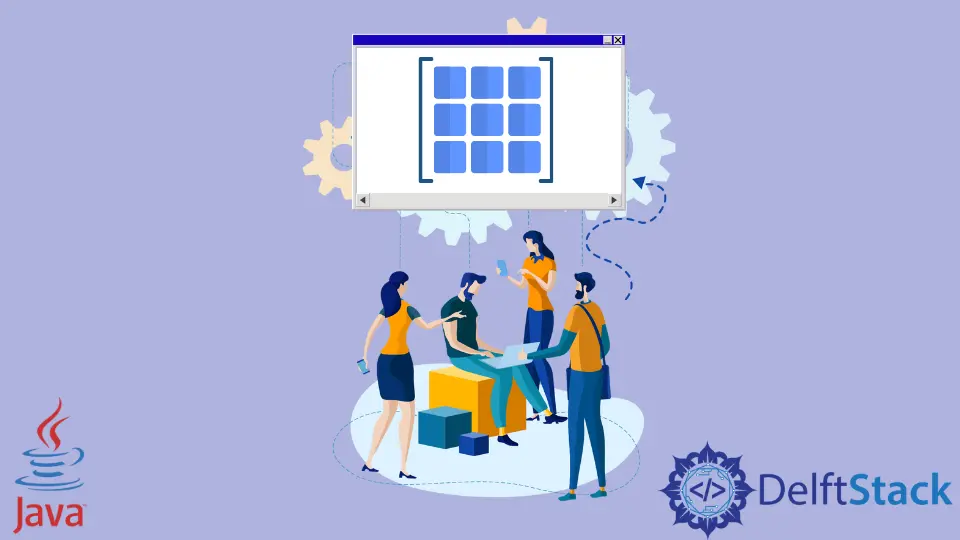
In this article, we will learn how to initialize a 2D array in Java. A 2D array is an array of one-dimensional arrays. The elements in a 2D array are arranged in rows and columns in the form of a matrix.
Initialize 2D Array in Java Using the new
Keyword and the for
Loop
In the code given below, we have a char
array - table
that is declared using the new
keyword. The 2-dimensional array table
has 3 rows and 3 columns.
All the elements in an array have their default values if no value is provided. In the case of an int
type 2-dimensional array, the default value, 0, is assigned to each element. Since we have a char
type 2-dimensional array, the default value will be null - \0
.
To iterate through each element of a 2-dimensional array, we need to use nested for
loops. We can visualize table
like 3 individual arrays of length 3. The expression table[row].length
denotes the number of columns, which is 3 in this case.
The expression '1' + row * 3 + col
where we vary row and column between 0 and 2 gives us a character from 1 to 9. This method works only for this case where the row and column length is 3.
Later, we print the values of the 2-dimensional array in a matrix form, as shown in the code below.
public class Array {
public static void main(String[] args) {
char[][] table = new char[3][3];
for (int row = 0; row < table.length; row++) {
for (int col = 0; col < table[row].length; col++) {
table[row][col] = (char) ('1' + row * 3 + col);
}
}
for (int row1 = 0; row1 < table.length; row1++) {
for (int col1 = 0; col1 < table[row1].length; col1++)
System.out.print(table[row1][col1] + " ");
System.out.println();
}
}
}
Output:
1 2 3
4 5 6
7 8 9
Initialize 2D Array in Java Without Using the Initializer
The most common way to declare and initialize a 2-dimensional array in Java is using a shortcut syntax with an array initializer. Here using {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}}
, we enclose each row’s initialization list in its own set of braces.
The expression above describes that we have a 2-dimensional array with 3 rows and 3 columns. In this way, we have declared and initialized a 2-dimensional array in a single line of code. The 2-dimensional array is then printed using a nested for
loop, as shown below.
public class Array {
public static void main(String[] args) {
int[][] arr = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int row = 0; row < 3; row++) {
for (int col = 0; col < 3; col++) System.out.print(arr[row][col] + " ");
System.out.println();
}
}
}
Output:
1 2 3
4 5 6
7 8 9
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn