How to Convert List to Array in Java
-
Using the
toArray()
Method - Using toArray() Without Arguments
- Using Java Streams
- Summary of Methods
- Conclusion
- FAQ
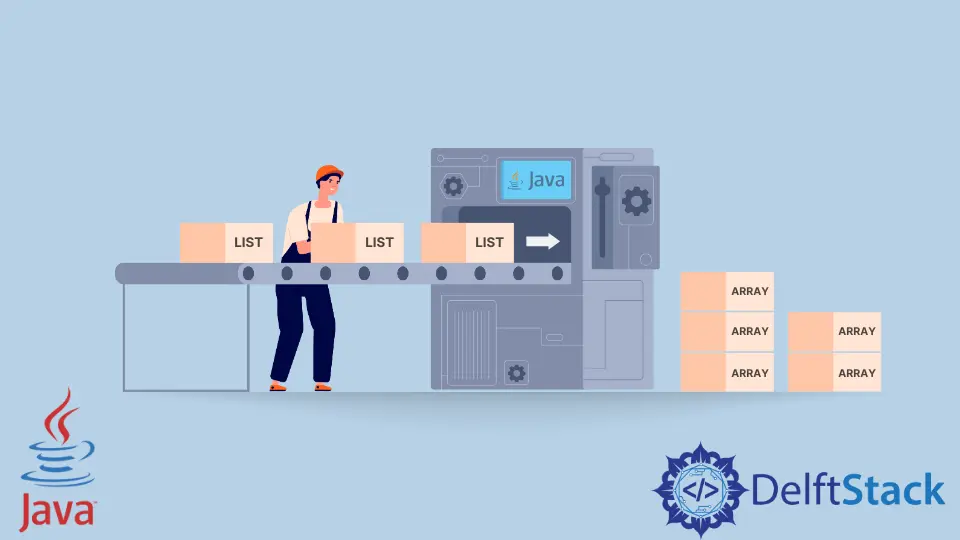
Converting a list to an array in Java is a common task that developers encounter frequently. Whether you’re working with data structures or handling collections, knowing how to efficiently transform a list into an array can streamline your code and improve performance.
In this article, we will explore various methods to achieve this conversion, focusing on the built-in capabilities of Java’s Collections Framework. From using the toArray()
method to leveraging streams, we will cover the essentials you need to know. So, if you’re ready to enhance your Java skills and make your code more efficient, let’s dive in!
Using the toArray()
Method
One of the simplest ways to convert a list to an array in Java is by using the toArray()
method provided by the List
interface. This method is straightforward and efficient, making it a popular choice among Java developers.
Here’s how it works:
import java.util.ArrayList;
import java.util.List;
public class ListToArrayExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Java");
list.add("Python");
list.add("JavaScript");
String[] array = list.toArray(new String[0]);
for (String language : array) {
System.out.println(language);
}
}
}
Output:
Java
Python
JavaScript
In this example, we first create a list of programming languages. By calling toArray(new String[0])
, we convert the list into an array of strings. The parameter new String[0]
serves as a template for the array size, allowing Java to allocate the appropriate amount of memory. The for
loop then iterates through the newly created array and prints each language. This method is efficient and works well for most scenarios.
Using toArray() Without Arguments
Another variant of the toArray()
method allows you to convert a list to an array without passing any arguments. However, this approach has its nuances that you should be aware of.
Here’s how you can implement it:
import java.util.ArrayList;
import java.util.List;
public class ListToArrayExample {
public static void main(String[] args) {
List<Integer> list = new ArrayList<>();
list.add(1);
list.add(2);
list.add(3);
Integer[] array = list.toArray(new Integer[0]);
for (Integer number : array) {
System.out.println(number);
}
}
}
Output:
1
2
3
In this example, we create a list of integers. By calling toArray()
without any parameters, Java creates a new array of the same type as the list’s elements. This method is convenient but can lead to an ArrayStoreException
if the array type doesn’t match the list’s element type. Therefore, it’s best practice to always specify the type when using toArray()
to avoid potential issues.
Using Java Streams
If you’re working with Java 8 or later, you can take advantage of the Stream API to convert a list to an array. This method is not only elegant but also allows for additional processing during the conversion.
Here’s an example:
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
public class ListToArrayExample {
public static void main(String[] args) {
List<String> list = new ArrayList<>();
list.add("Apple");
list.add("Banana");
list.add("Cherry");
String[] array = list.stream().toArray(String[]::new);
for (String fruit : array) {
System.out.println(fruit);
}
}
}
Output:
Apple
Banana
Cherry
In this code, we first create a list of fruits. By using list.stream().toArray(String[]::new)
, we convert the list into an array. This method is particularly powerful because it allows for further operations, such as filtering or mapping, before the final conversion. The syntax may seem a bit complex at first, but it offers a lot of flexibility and can lead to more readable code when used correctly.
Summary of Methods
To summarize, there are several effective methods to convert a list to an array in Java:
- Using the
toArray()
method with a type-specific array. - Using
toArray()
without arguments, while being cautious of type mismatches. - Leveraging Java Streams for a more functional approach.
Each method has its own advantages and can be chosen based on the specific requirements of your project.
Conclusion
Converting a list to an array in Java is a valuable skill that can enhance your programming efficiency. Whether you choose the traditional toArray()
method or the more modern Stream API, understanding these methods will empower you to write cleaner and more effective Java code. As you continue to work with Java, keep these techniques in mind to handle data transformations seamlessly. Happy coding!
FAQ
-
What is the difference between toArray() and toArray(T[] a)?
ThetoArray()
method returns an array ofObject
, whiletoArray(T[] a)
returns an array of the specified type. -
Can I convert a list of different types to an array?
No, Java arrays are homogenous, meaning all elements must be of the same type. -
What happens if I pass a smaller array to toArray()?
If the provided array is smaller than the list, a new array of the same type will be created. -
Is using streams for conversion more efficient?
Streams can be more flexible and readable, but they may introduce overhead compared to direct methods. -
Can I convert a list of primitives to an array?
You cannot directly create an array of primitives from a list. You need to use their wrapper classes (e.g., Integer for int).