How to Convert a String to Long in Java
-
Long.parseLong()
to Convert String to Long in Java -
new Long(str).longValue()
to Convert Sting to Long in Java -
Long.valueOf().longValue()
to Convert Sting to Long in Java
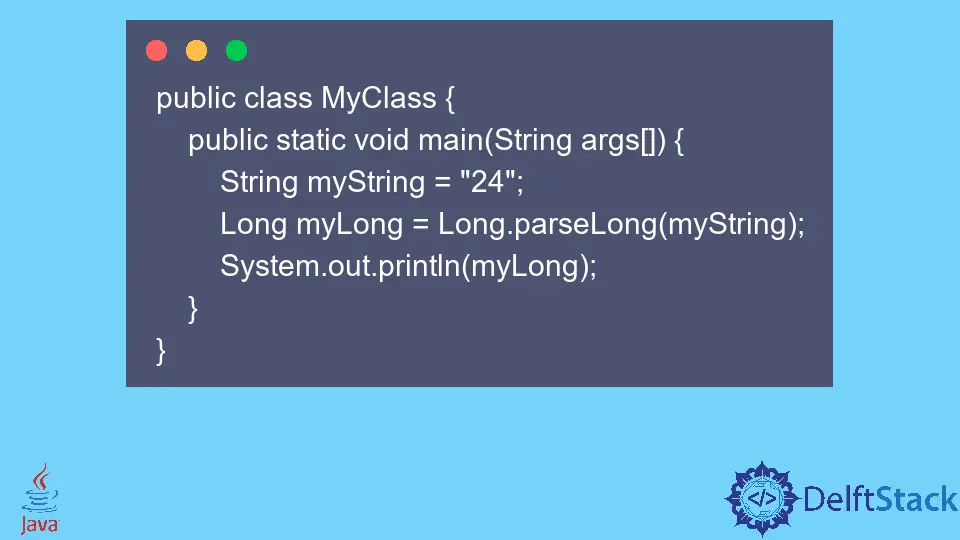
This tutorial discusses methods to convert a String
to a Long
in Java.
Long.parseLong()
to Convert String to Long in Java
We can use a built-in method of the Long
class to convert a String
representing a number to Long
.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "24";
Long myLong = Long.parseLong(myString);
System.out.println(myLong);
}
}
Output:
> 24
However, if the input String
does not represent a valid number, this method will throw an error.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "one";
Long myLong = Long.parseLong(myString);
System.out.println(myLong);
}
}
Output:
>
Exception in thread "main" java.lang.NumberFormatException: For input string: "one"
at java.base/java.lang.NumberFormatException.forInputString(NumberFormatException.java:65)
at java.base/java.lang.Long.parseLong(Long.java:692)
at java.base/java.lang.Long.parseLong(Long.java:817)
at MyClass.main(MyClass.java:4)
We can use a try-catch
block to avoid running into this issue.
public class MyClass {
public static void main(String args[]) {
String myString = "one";
Long myLong;
try {
myLong = Long.parseLong(myString);
System.out.println(myLong);
} catch (Exception e) {
System.out.println("The input string does not represent a valid number");
}
}
}
Output:
> The input string does not represent a valid number
new Long(str).longValue()
to Convert Sting to Long in Java
We can create a new Long
object which takes input a String
representing a number and returns a new Long
.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "1";
Long myLong;
try {
myLong = new Long(myString).longValue();
System.out.println(myLong);
} catch (Exception e) {
System.out.println("The input string does not represent a valid number");
}
}
}
Output:
1
Long.valueOf().longValue()
to Convert Sting to Long in Java
Long
class also provides another method to convert a String
representing a number to Long
.
The below example illustrates this:
public class MyClass {
public static void main(String args[]) {
String myString = "1";
Long myLong;
try {
myLong = Long.valueOf(myString).longValue();
System.out.println(myLong);
} catch (Exception e) {
System.out.println("The input string does not represent a valid number");
}
}
}
Out of the three discussed methods, we can use any of it to convert a valid String
to a Long
. We can skip the try-catch
block if we are sure that the given String
represents a valid number or if we do not want to handle exceptions gracefully.