How to Get List of Files in Directory in C++
-
Use
std::filesystem::directory_iterator
to Get a List of Files in a Directory -
Use
opendir/readdir
Functions to Get a List of Files in a Directory -
Use
std::filesystem::recursive_directory_iterator
to Get a List of Files in All Subdirectories - Use Windows API Functions to Get a List of Files in a Directory (Windows)
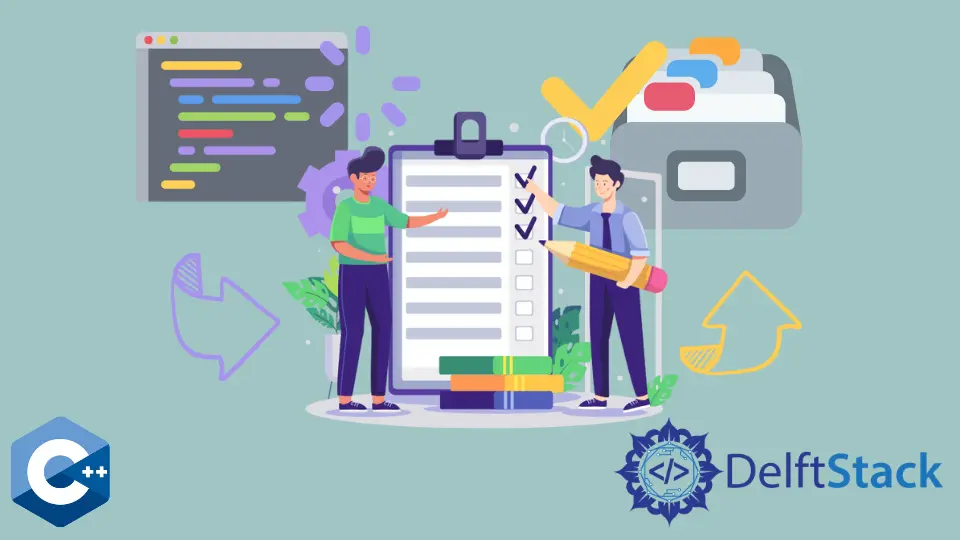
In this article, we will introduce ways to get a list of files in a specified directory in C++.
Use std::filesystem::directory_iterator
to Get a List of Files in a Directory
This method is part of the <filesystem>
library added in C++17. Note that some older compilers might not have support for this method, but this is the native C++ solution to get list of files in the directory.
directory_iterator
is used in a range-based for
loop, and it takes the path to the directory as an argument. In this example, we specify a path to the root directory, but you should modify this variable based on your system to pass a valid path.
Function Syntax
std::filesystem::directory_iterator
The directory_iterator
class provides a convenient way to iterate through the contents of a directory. It takes the path to the directory as its argument and can be used in a range-based for
loop to access the files within that directory.
Example Code
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::directory_iterator;
int main() {
string path = "/";
for (const auto& file : directory_iterator(path)) cout << file.path() << endl;
return EXIT_SUCCESS;
}
Output (Depends on the Directory):
Archive2| lib| .| ..| lib64| Archive| dev| var| bin| usr| etc| tmp| proc|
Use opendir/readdir
Functions to Get a List of Files in a Directory
This method is much more verbose, but it’s a reliable alternative for the file system manipulation.
To use the functions opendir
/readdir
, you should include the dirent.h
header file.
Function Syntax
DIR* opendir(const char* name);
struct dirent* readdir(DIR* dirp);
opendir
opens a directory specified by name and returns its pointer, which is of DIR*
.
readdir
operates on DIR*
argument and returns a special struct called dirent
(see full info).
Once we put readdir
call in a while
loop comparing to nullptr
, it acts as a directory iterator, and we can get access to filenames through each returned the dirent
struct.
Example Code
#include <dirent.h>
#include <iostream>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::vector;
int main() {
DIR *dir;
struct dirent *diread;
vector<char *> files;
if ((dir = opendir("/")) != nullptr) {
while ((diread = readdir(dir)) != nullptr) {
files.push_back(diread->d_name);
}
closedir(dir);
} else {
perror("opendir");
return EXIT_FAILURE;
}
for (auto file : files) cout << file << "| ";
cout << endl;
return EXIT_SUCCESS;
}
Notice that much of if
statements act as exception handling mechanisms since opendir
and readdir
functions return NULL
pointers on error/end of the directory.
Use std::filesystem::recursive_directory_iterator
to Get a List of Files in All Subdirectories
This method is useful when multiple subdirectories should be searched for specific filenames. The method stays the same as directory_iterator
. You just have to loop through it with range-based loop and operate on files as needed.
Function Syntax
std::filesystem::recursive_directory_iterator
This class allows you to traverse through all subdirectories and their contents recursively.
Example Code
#include <filesystem>
#include <iostream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::filesystem::recursive_directory_iterator;
int main() {
string path = "./";
for (const auto& file : recursive_directory_iterator(path))
cout << file.path() << endl;
return EXIT_SUCCESS;
}
This method is particularly useful when dealing with nested subdirectories and the need to obtain a comprehensive list of files across the entire directory structure.
Use Windows API Functions to Get a List of Files in a Directory (Windows)
Another method to retrieve a list of files in a directory, especially on Windows systems, involves using the Windows API functions. While this method is more specific to the Windows operating system, it can be quite powerful and gives you fine-grained control over file retrieval.
Function Syntax
The Windows API provides functions for working with directories and files. One such function is FindFirstFile()
, which searches for a file or subdirectory in a specified directory.
This function requires two arguments: the path to the directory (including a wildcard character) and a pointer to a structure that receives information about the first file or subdirectory found.
Once the initial search is performed, you can use the FindNextFile()
function to continue searching for subsequent files.
HANDLE FindFirstFile(LPCTSTR lpFileName, LPWIN32_FIND_DATA lpFindFileData);
BOOL FindNextFile(HANDLE hFindFile, LPWIN32_FIND_DATA lpFindFileData);
Here’s how you can use these functions to get a list of files in a directory:
Example Code
#include <windows.h>
#include <iostream>
int main() {
WIN32_FIND_DATA findFileData;
HANDLE hFind = FindFirstFile("path_to_directory\\*", &findFileData);
if (hFind != INVALID_HANDLE_VALUE) {
do {
if (findFileData.dwFileAttributes & FILE_ATTRIBUTE_NORMAL) {
std::cout << findFileData.cFileName << std::endl;
}
} while (FindNextFile(hFind, &findFileData) != 0);
FindClose(hFind);
}
return 0;
}
In the above example, the FindFirstFile()
function is used to initiate the search for files in the specified directory, and the FindNextFile()
function is used to continue the search for subsequent files. The WIN32_FIND_DATA
structure holds information about the found files, such as file names and attributes.
Explanation
- The
FindFirstFile()
function returns a handle to the search, which can be used for subsequent operations. - The
FindNextFile()
function continues the search for the next file in the directory. - The
INVALID_HANDLE_VALUE
is returned byFindFirstFile()
if the search fails. - The
FILE_ATTRIBUTE_NORMAL
attribute checks if the found item is a normal file.
While this method is specific to Windows, it provides a powerful way to interact with the file system and obtain detailed information about files in a directory.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook