How to Declare a Global Variable in C++
- Declare a Global Variable in a Single Source File in C++
- Declare a Global Variable in Multiple Source Files in C++
- Advantages of Using Global Variables
- Disadvantages of Using Global Variables
- Best Practices for Using Global Variables
- Conclusion
- FAQ
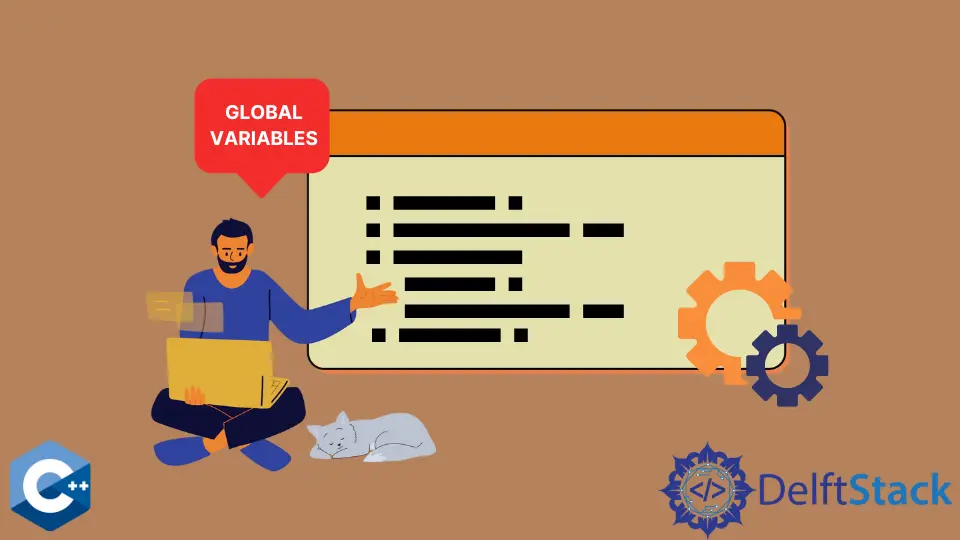
Declaring a global variable in C++ is a fundamental concept that every programmer should master. Global variables are accessible from any function within the program, making them a powerful tool for sharing data across different parts of your code. However, with great power comes great responsibility. Improper use of global variables can lead to code that is difficult to maintain and debug.
In this article, we will explore the process of declaring global variables in C++, discuss their advantages and disadvantages, and provide clear examples to illustrate how they work in practice. Whether you are a beginner or an experienced developer, understanding global variables will enhance your coding skills and improve your programming efficiency.
Declare a Global Variable in a Single Source File in C++
We can declare a global variable with the statement that is placed outside of every function. In this example, we assume the int
type variable and initialize it to an arbitrary 123
value. The global variable can be accessed from the scope of the main
function as well as any inner construct (loop or if statement) inside it. Modifications to the global_var
are also visible to each part of the main
routine, as demonstrated in the following example.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
int main() {
global_var += 1;
cout << global_var << endl;
for (int i = 0; i < 4; ++i) {
global_var += i;
cout << global_var << " ";
}
cout << endl;
return EXIT_SUCCESS;
}
Output:
124
124 125 127 130
On the other hand, if additional functions are also defined in the same source file, they can directly access the global_var
value and modify it in the function scope. The global variables can also be declared with a const
specifier, which forces them to only be accessible through the current translation unit (source file with all included headers).
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
void tmpFunc() {
global_var += 1;
cout << global_var << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
Output:
124
Declare a Global Variable in Multiple Source Files in C++
Alternatively, there may be global variables declared in different source files, and needed to be accessed or modified. In this case, to access the global variable, it needs to be declared with an extern
specifier, which tells the compiler (more precisely the linker) where to look for the glob_var1
definition.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
extern int glob_var1; // Defined - int glob_var1 = 44; in other source file
void tmpFunc() {
glob_var1 += 1;
cout << glob_var1 << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
There might be global variables declared with a static
specifier in the different source files in some cases. These variables are accessible only within the file where they are defined and can’t be reached from other files. If you still try to declare them with extern
in the current file, the compiler error will occur.
#include <iostream>
using std::cout;
using std::endl;
int global_var = 123;
extern int
glob_var2; // Defined - static int glob_var2 = 55; in other source file
void tmpFunc() {
glob_var2 += 1;
cout << glob_var2 << endl;
}
int main() {
tmpFunc();
return EXIT_SUCCESS;
}
Advantages of Using Global Variables
Global variables come with several advantages that can simplify coding in certain situations. One of the main benefits is ease of access. Since global variables can be accessed from any function within the file, you don’t have to pass them as parameters. This reduces the complexity of function calls, especially in larger programs.
Another advantage is that global variables can help maintain state across function calls. For instance, if you have a counter that needs to be incremented in multiple functions, using a global variable can make this process straightforward.
However, it’s essential to use global variables judiciously. Overusing them can lead to code that is hard to follow and maintain. Developers might find it challenging to track where and how global variables are modified, leading to bugs that are difficult to diagnose.
Disadvantages of Using Global Variables
While global variables offer convenience, they also come with significant drawbacks. One of the primary concerns is that they can lead to unintended side effects. Since any function can modify a global variable, it becomes challenging to predict how changes in one part of the code will affect other parts. This can introduce bugs that are hard to trace.
Additionally, global variables can hinder code reusability. Functions that rely on global variables are less modular, making it difficult to use them in different contexts without bringing along the global state. This can lead to tightly coupled code, which is harder to maintain and test.
Another issue is that global variables can create challenges in multi-threaded applications. If two threads attempt to modify a global variable simultaneously, it can lead to race conditions, resulting in unpredictable behavior.
Best Practices for Using Global Variables
To effectively use global variables while minimizing their downsides, consider these best practices:
-
Limit Scope: Only declare global variables when necessary. If a variable is only needed in a specific function, consider declaring it as a local variable instead.
-
Use Constants: If a global variable does not need to change, declare it as a constant. This prevents accidental modifications and enhances code clarity.
-
Encapsulation: Use classes or structures to encapsulate global variables. This way, you can control access and modifications, reducing the risk of unintended side effects.
-
Documentation: Clearly document the purpose and usage of global variables. This helps other developers understand their role in the codebase and reduces the likelihood of misuse.
-
Avoid Global State: Whenever possible, try to avoid relying on global state. Instead, pass variables as parameters to functions to make dependencies explicit.
By following these best practices, you can leverage the advantages of global variables while mitigating their potential pitfalls.
Conclusion
Declaring global variables in C++ is a straightforward process that can significantly enhance your programming capabilities. While they offer convenience and ease of access, it’s crucial to use them wisely to avoid complications in your code. By understanding the advantages and disadvantages of global variables, as well as implementing best practices, you can write cleaner, more maintainable code. As you continue to develop your skills in C++, keep global variables in mind, but always consider their impact on your program’s structure and readability.
FAQ
-
What is a global variable in C++?
A global variable is defined outside of any function and is accessible from any function within the program. -
Can I modify a global variable inside a function?
Yes, you can modify a global variable inside any function, which can lead to unintended side effects if not managed carefully. -
What are the disadvantages of using global variables?
Global variables can lead to unintended side effects, hinder code reusability, and create challenges in multi-threaded applications. -
How can I minimize the risks associated with global variables?
Limit their scope, use constants, encapsulate them in classes, document their purpose, and avoid relying on global state when possible. -
Are there alternatives to using global variables?
Yes, you can pass variables as parameters to functions, use local variables, or encapsulate them within classes to maintain modularity and reduce dependencies.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook