How to Find Square Root Using Babylonian Method in C++
- Babylonian Method for Square Root in C++
- Implement the Babylonian Method to Find the Square Root in C++
- Conclusion
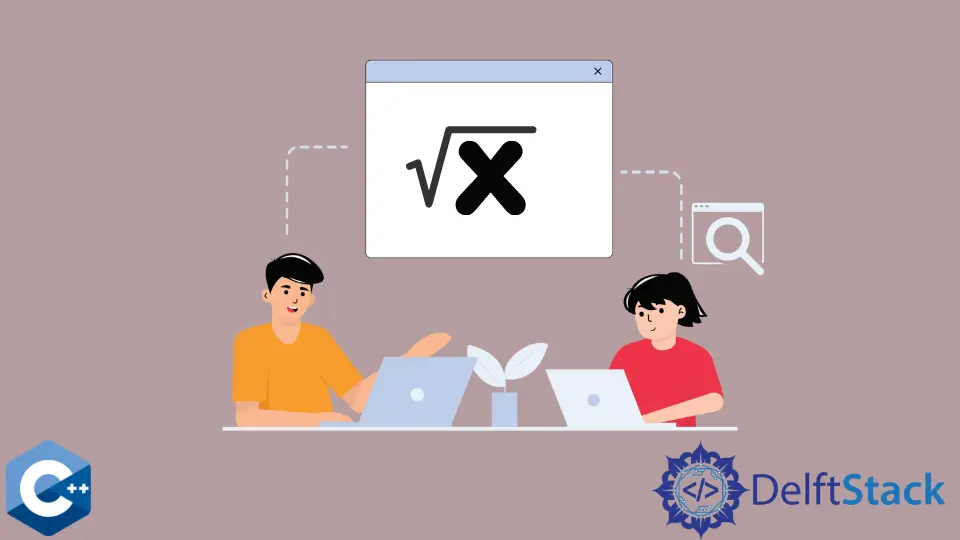
The following article explores the Babylonian method in square root calculations, emphasizing its efficiency and simplicity through a detailed examination of a practical C++ implementation.
Babylonian Method for Square Root in C++
The Babylonian method is chosen in C++ for square root calculation due to its simplicity and efficiency, which in turn is derived from the Newton-Raphson approach for the solution of non-linear equations. It offers a fast convergence rate, providing accurate approximations in a few iterations.
This iterative algorithm is well-suited for computing square roots programmatically, making it suitable for applications where real-time or efficient numerical solutions are essential. Additionally, its straightforward implementation facilitates easy integration into C++ programs, making it a practical choice for developers seeking a balance between simplicity and computational effectiveness in square root calculations.
Implement the Babylonian Method to Find the Square Root in C++
In the realm of numerical methods, the Babylonian method stands as a reliable and efficient approach for approximating square roots. Its significance lies in its iterative nature, offering a balance between simplicity and computational efficiency.
This article unveils the intricacies of the Babylonian method in C++, demonstrating how it swiftly and accurately computes square roots, making it a valuable tool for various applications.
Code Example:
#include <cmath>
#include <iostream>
double babylonianSquareRoot(int n, double epsilon = 0.0001) {
if (n < 0) {
std::cerr << "Cannot find square root of a negative number." << std::endl;
return -1.0;
}
double guess = n / 2.0;
while (std::abs(guess * guess - n) > epsilon) {
guess = 0.5 * (guess + n / guess);
}
return guess;
}
int main() {
int num;
std::cout << "Enter a non-negative integer: ";
std::cin >> num;
double result = babylonianSquareRoot(num);
if (result >= 0.0) {
std::cout << "Square root is approximately: " << result << std::endl;
}
return 0;
}
The Babylonian method, as illustrated in the provided C++ code, operates through a systematic step-by-step process. Initially, the function babylonianSquareRoot
is defined to accept an integer n
and an optional convergence tolerance parameter epsilon
.
It incorporates a check for negative input values. Subsequently, the algorithm starts with an initial guess set at half of the input value (n / 2.0
).
Through iterative refinement, facilitated by a while
loop, the guess is continuously updated using the Babylonian formula until the absolute difference between the square of the guess and n
is below the specified epsilon.
In the main
function, users input a non-negative integer, initiating the Babylonian method to compute the square root, with the result displayed if it is non-negative.
Output:
This comprehensive and concise explanation elucidates the inner workings of the Babylonian method, emphasizing its simplicity and efficiency in square root calculations.
The Babylonian method elegantly marries simplicity with efficiency, providing a robust means to calculate square roots. The demonstrated C++ implementation showcases its practicality, offering a valuable tool for numerical computations in diverse programming scenarios.
Conclusion
The Babylonian method emerges as a powerful and efficient tool for approximating square roots in C++. Its iterative nature, showcased in the provided code example, elegantly balances simplicity and computational effectiveness.
By progressively refining an initial guess through the iterative process, the Babylonian method swiftly converges to an accurate solution. This approach not only makes it a valuable asset in numerical computations but also highlights its versatility for diverse programming applications.
Incorporating the Babylonian method into C++ provides a clear and concise solution for obtaining precise square root approximations, making it a noteworthy technique in the programmer’s toolkit.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn