How to Initialize Array of Structs in C
- Use List Notation to Initialize Array of Structs in C
- Use A Separate Function and Loop to Initialize Array of Structs in C
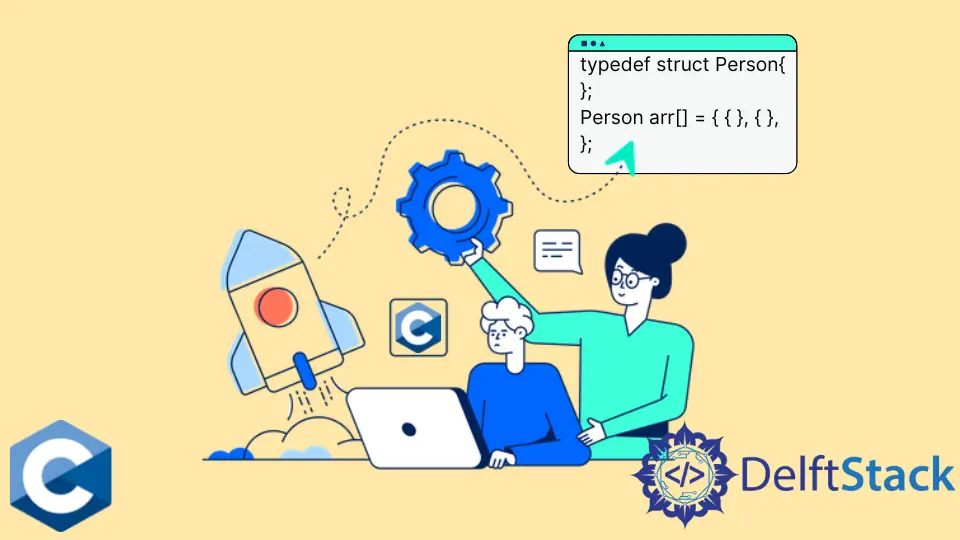
This article will demonstrate multiple methods about how to initialize an array of struct
in C.
Use List Notation to Initialize Array of Structs in C
Structures are derived data types that usually consist of multiple members. Note that the member declaration order in the struct
definition matters, and when the initializer list is used, it follows the same order. In the following example, we define a struct
named Person
, which includes 2 char
arrays, an int
and a bool
. Consequently, we declare an array of Person
structures and initialize it with curly braced lists as we would the single data type array. Then we output the initialized array elements using the for
loop. Mind though, char
arrays are printed out with %s
format specifier since we included \0
byte in each string literal in the initializer lists.
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Person {
char firstname[40];
char lastname[40];
int age;
bool alive;
} Person;
int printPerson(Person *p) {
if (p == NULL) return -1;
printf("Name: %s\nLast Name: %s\nAge: %d\nAlive: ", p->firstname, p->lastname,
p->age);
p->alive ? printf("Yes\n") : printf("No\n");
return 0;
}
int main(void) {
Person arr[] = {
{"John\0", "McCarthy\0", 24, 1},
{"John\0", "Kain\0", 27, 1},
};
size_t size = sizeof arr / sizeof arr[0];
for (int i = 0; i < size; ++i) {
printPerson(&arr[i]);
printf("\n");
}
exit(EXIT_SUCCESS);
}
Output:
Name: John
Last Name: McCarthy
Age: 24
Alive: Yes
Name: John
Last Name: Kain
Age: 27
Alive: Yes
Use A Separate Function and Loop to Initialize Array of Structs in C
The downside of the previous method is that array can be initialized with hard-coded values, or the bigger the array needs to be, the bigger the initialization statement will be. Thus, we should implement a single struct
element initialization function and call it from the iteration to do the struct
array. Note that, initPerson
function takes all struct
member values as arguments and assigns them to the Person
object that also has been passed as a parameter. Finally, we output each element of the array to the console using the printPerson
function. Notice that we pass the same Person
values to the initialization function for demonstration purposes only.
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Person {
char firstname[40];
char lastname[40];
int age;
bool alive;
} Person;
int printPerson(Person *p) {
if (p == NULL) return -1;
printf("Name: %s\nLast Name: %s\nAge: %d\nAlive: ", p->firstname, p->lastname,
p->age);
p->alive ? printf("Yes\n") : printf("No\n");
return 0;
}
int initPerson(Person *p, char *fn, char *ln, int age, bool alive) {
if (p == NULL) return -1;
memmove(&p->firstname, fn, strlen(fn) + 1);
memmove(&p->lastname, ln, strlen(ln) + 1);
p->age = age;
p->alive = alive;
return 0;
}
enum { LENGTH = 10 };
int main(void) {
Person me = {"John\0", "McCarthy\0", 24, 1};
Person arr[LENGTH];
for (int i = 0; i < LENGTH; ++i) {
initPerson(&arr[i], me.firstname, me.lastname, me.age, me.alive);
}
for (int i = 0; i < LENGTH; ++i) {
printPerson(&arr[i]);
printf("\n");
}
exit(EXIT_SUCCESS);
}
Output:
Name: John
Last Name: McCarthy
Age: 24
Alive: Yes
Name: John
Last Name: Kain
Age: 27
Alive: Yes
...
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook