Two-Way Data Binding in Angular 2
- Understanding Two-Way Data Binding
- Implementing Two-Way Data Binding with ngModel
- Benefits of Two-Way Data Binding in Angular 2
- Conclusion
- FAQ
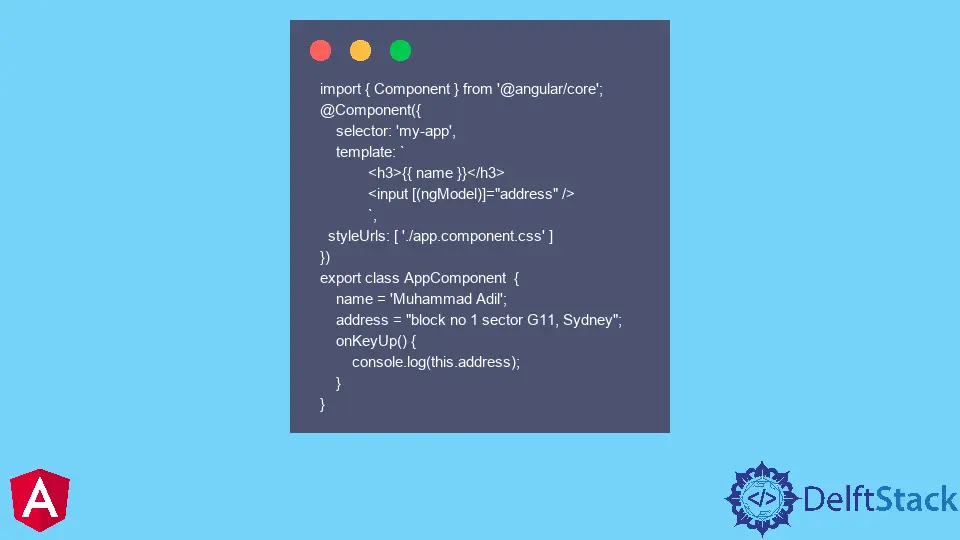
In the realm of web development, Angular 2 has emerged as a powerful framework, particularly known for its efficient data binding capabilities. Among these, two-way data binding stands out as a crucial feature that allows for seamless communication between the model and the view. This means that any changes made in the user interface are immediately reflected in the model and vice versa. To achieve this in Angular 2, we primarily utilize the ngModel
directive in conjunction with the ngModelChange
event.
In this article, we will explore how to effectively implement two-way data binding in Angular 2, ensuring a smooth and responsive user experience.
Understanding Two-Way Data Binding
Two-way data binding is a technique that allows automatic synchronization of data between the model and the UI components. In Angular 2, this is accomplished through the use of the ngModel
directive, which binds the value of an HTML input element to a property in the component. When the user modifies the input, the model updates automatically. Conversely, if the model changes, the UI reflects that change instantly. This dynamic interaction simplifies the development process and enhances user engagement.
Implementing Two-Way Data Binding with ngModel
To implement two-way data binding in Angular 2, the first step is to import the FormsModule
in your application module. This module provides the necessary directives for working with forms, including ngModel
. Once imported, you can use the ngModel
directive in your template to bind input elements to component properties.
Here’s a simple example demonstrating how to set up two-way data binding:
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: `
<input [(ngModel)]="username" (ngModelChange)="onUsernameChange($event)" />
<p>Your username is: {{ username }}</p>
`
})
export class AppComponent {
username: string = '';
onUsernameChange(event: string) {
console.log('Username changed to:', event);
}
}
In this example, we have an input field bound to a username
property in the component. The [(ngModel)]
syntax indicates two-way data binding. When the user types into the input field, the username
property updates automatically. The ngModelChange
event captures any changes and triggers the onUsernameChange
method, which logs the new username to the console.
Output:
Username changed to: new_username
This approach is straightforward and effective, enabling developers to create dynamic forms with ease. The combination of ngModel
and ngModelChange
facilitates real-time updates, enhancing the interactivity of Angular applications.
Benefits of Two-Way Data Binding in Angular 2
The advantages of two-way data binding in Angular 2 are manifold. Firstly, it simplifies the synchronization of data between the model and the view, reducing the amount of boilerplate code developers need to write. Instead of manually updating the model or the view, Angular takes care of it automatically. This leads to cleaner, more maintainable code.
Moreover, two-way data binding enhances user experience by providing immediate feedback. Users can see the results of their actions in real time, making applications feel more responsive and intuitive. This is particularly important in scenarios where user input is critical, such as in forms or interactive components.
Additionally, using ngModel
allows for easier validation of user input. As the model updates, developers can implement validation logic that responds to changes, ensuring that the data remains consistent and valid. This feature is invaluable in creating robust applications that prioritize data integrity.
Conclusion
Two-way data binding in Angular 2 is a powerful feature that streamlines the interaction between the model and the user interface. By utilizing the ngModel
directive and the ngModelChange
event, developers can create dynamic and responsive applications that provide real-time feedback to users. This not only enhances user experience but also simplifies the development process, allowing for cleaner and more maintainable code. As you dive deeper into Angular 2, mastering two-way data binding will undoubtedly elevate your web development skills.
FAQ
-
What is two-way data binding in Angular 2?
Two-way data binding in Angular 2 is a technique that allows automatic synchronization of data between the model and the UI components. -
How do I implement two-way data binding in Angular 2?
You can implement two-way data binding using thengModel
directive along with thengModelChange
event in your Angular templates. -
What are the benefits of using two-way data binding?
Benefits include reduced boilerplate code, enhanced user experience through real-time feedback, and easier validation of user input. -
Do I need to import any modules for two-way data binding?
Yes, you need to import theFormsModule
in your Angular module to use thengModel
directive. -
Can I use two-way data binding with custom components?
Yes, you can implement two-way data binding with custom components by using@Input()
and@Output()
decorators along withngModel
.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook