How to Implement the sleep() Function in Angular
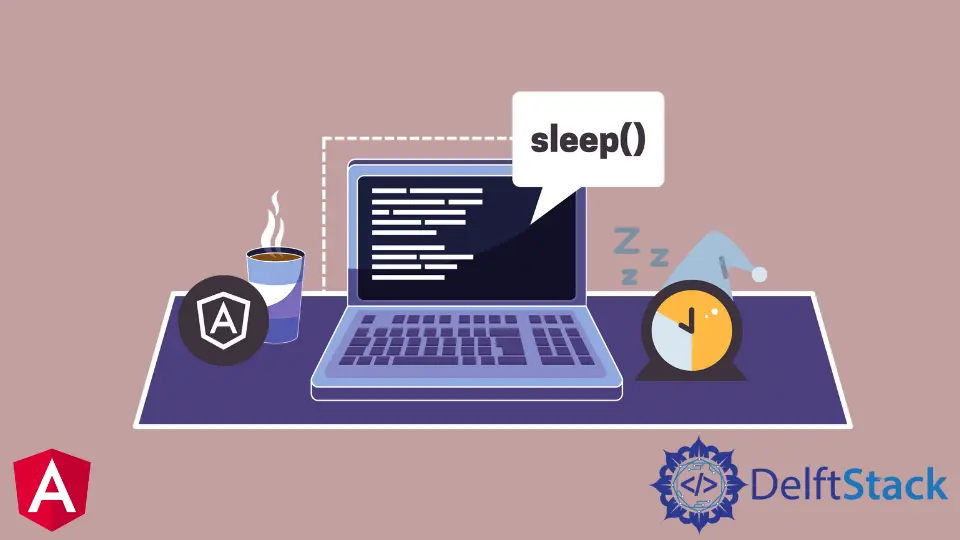
This article will discuss and provide the steps to implement the sleep()
function in Angular.
the sleep()
Function in TypeScript
The Angular sleep()
function is a new feature that will make your app more useful. In TypeScript, the Angular sleep()
function can pause the execution of a program for a specified number of milliseconds.
This pause can make the application more responsive and stop the application from running any unnecessary code. The sleep()
function in TypeScript is similar to JavaScript’s setTimeout()
and setInterval()
functions in its syntax, but it differs in how it works.
The process of delaying tasks is often done using JavaScript’s native setTimeout()
or setInterval()
functions. However, these functions have drawbacks when it comes to performance and responsiveness.
Hence, the Angular team introduced its version of this function, called sleep()
.
Steps to Implement a sleep()
Function in Angular
Let’s look at the steps to implement the Angular sleep()
function in TypeScript.
But before that, we need to import the RxJS library. This library is essential, and it has many functions that we can use in our application.
After that, we will create a new TypeScript script file called sleep.ts
and implement the following steps:
- Import the necessary modules.
- Implement the Angular
sleep()
function. - Test the function.
- Export it to a TypeScript file.
Example TypeScript Code:
import { Component } from '@angular/core';
import { interval } from 'rxjs';
import { take } from 'rxjs/operators';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title: string;
ngOnInit() {
const delay = 1000;
this.sleep(delay)
.then(() => this.title = '1')
.then(() => this.sleep(delay))
.then(() => this.title += '2')
.then(() => this.sleep(delay))
.then(() => this.title += '3')
.then(() => this.sleep(delay))
.then(() => this.title += '4')
.then(() => this.sleep(delay))
.then(() => this.title += '5')
}
sleep(milliseconds: number) {
let resolve: { (): any; (value: unknown): void; };
let promise = new Promise((_resolve) => {
resolve = _resolve;
});
setTimeout(() => resolve(), milliseconds);
return promise;
}
}
Click here to check the live demonstration of the code as mentioned above.
Muhammad Adil is a seasoned programmer and writer who has experience in various fields. He has been programming for over 5 years and have always loved the thrill of solving complex problems. He has skilled in PHP, Python, C++, Java, JavaScript, Ruby on Rails, AngularJS, ReactJS, HTML5 and CSS3. He enjoys putting his experience and knowledge into words.
Facebook