Python sys.platform Variable
-
Purpose of
sys.platform
in Python -
Syntax of Python
sys.platform
Variable -
Example 1: Use the
sys.platform
on LINUX -
Example 2: Use the
sys.platform
on Windows OS -
Example 3: Use the
sys.platform
Variable With Other Similar Variables and Methods - Example 4: Platform-Dependent Execution
- Example 5: Platform-Specific Path Handling
- Example 6: Platform-Specific Libraries/Modules Import
- Use Cases and Considerations
- Conclusion
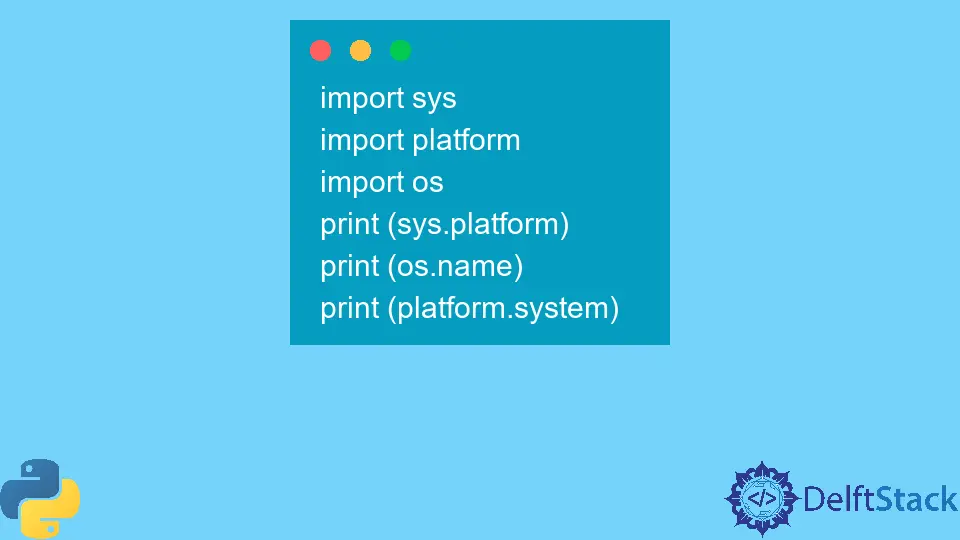
The sys.platform
variable in Python is a part of the sys
module, which provides access to system-specific parameters and functions. This variable stores information about the platform or operating system where the Python interpreter is executing.
It is immensely valuable when writing platform-independent code or when certain functionalities need to adapt based on the underlying operating system.
Python sys.platform
is an efficient way to get information about the platform used. It also returns the platform identifier we can use to change and edit some OS-specific components like sys.path
, for instance.
Purpose of sys.platform
in Python
The sys.platform
serves as a reliable means to identify the underlying operating system or platform. It returns a string that specifies the platform’s identifier, allowing developers to write code that can adapt or execute specific behaviors based on the detected platform.
This is especially useful when dealing with functionalities that have different implementations or requirements across different operating systems.
Syntax of Python sys.platform
Variable
sys.platform
Parameters
The sys.platform
has no parameters. It is a non-callable object.
Return Value
The variable platform
will contain a string indicating the current platform where the Python interpreter is running. The returned string corresponds to specific identifiers for various operating systems.
The sys.platform
return type is a string containing a platform identifier for the OS in use. The list below mentions some of the OS platforms and their returned value:
System | Platform Value |
---|---|
Linux | linux or linux2 |
Windows | win32 |
Windows/Cygwin | cygwin |
Windows/MSYS2 | msys |
Mac OS X | darwin |
This information enables conditional execution of code blocks tailored to different platforms.
Example 1: Use the sys.platform
on LINUX
This basic example uses the sys.platform
attribute from the sys
module in Python to retrieve and print the identifier of the current operating system or platform where the Python interpreter is running.
When executed, this code will output a string indicating the identifier of the platform. For example:
- On a Windows system, it might print
'win32'
. - On macOS, it might display
'darwin'
. - On a Linux-based system, it could show
'linux'
.
import sys
print(sys.platform)
Output:
linux
The above code is executed on a LINUX platform, so the method returns that specific platform identifier.
Example 2: Use the sys.platform
on Windows OS
This is the same code as the above example but executed in Windows OS.
import sys
print(sys.platform)
Output:
win32
The above code is executed on a WINDOWS platform, so the method returns that specific platform identifier.
Example 3: Use the sys.platform
Variable With Other Similar Variables and Methods
This example demonstrates how to access and display information regarding the current operating system through various modules (sys
, platform
, and os
). It showcases different attributes or functions to fetch details such as the platform identifier, the module name in use for the OS, and the system name.
import sys
import platform
import os
print(sys.platform)
print(os.name)
print(platform.system)
Output:
win32
nt
<function system at 0x000001C2D2439750>
The sys.platform
gives us information about the build configuration. On the other hand, the os.name
checks for the availability of specific OS-specific modules such as POSIX
, nt
, etc.
The platform.system
method determines the system type at run time.
Example 4: Platform-Dependent Execution
This example employs sys.platform
in Python to identify the operating system and execute platform-specific code. It employs conditional statements (if
, elif
, else
) to discern macOS, Linux, Windows, or unidentified platforms.
Based on the detected platform, it triggers specific actions or outputs tailored messages for recognized or unrecognized operating systems. This use of sys.platform
facilitates conditional execution, allowing developers to adapt code behavior according to the underlying platform.
import sys
if sys.platform == "darwin":
print("Running on macOS.")
# Execute specific code for the macOS platform
elif sys.platform == "linux":
print("Running on a Linux-based system.")
# Execute specific code for the Linux platform
elif sys.platform.startswith("win"):
print("Running on a Windows system.")
# Execute specific code for the Windows platform
else:
print("Platform identification not recognized.")
# Execute default behavior or provide a message for unrecognized platforms
Output:
Running on a Linux-based system.
Example 5: Platform-Specific Path Handling
This code segment uses sys.platform
to differentiate between Windows and Unix-like operating systems.
Depending on the detected platform, it performs platform-specific operations, such as handling file paths using appropriate conventions (\\
for Windows and /
for Unix-like systems).
import sys
if sys.platform.startswith("win"):
# Windows-specific path handling
print("Running on a Windows system.")
# Perform actions specific to Windows file paths
# For instance: path = 'C:\\Users\\Username\\Documents\\file.txt'
else:
# Unix-like or other platform path handling
print("Running on a Unix-like system.")
# Perform actions specific to Unix-like file paths
# For instance: path = '/home/username/documents/file.txt'
Output:
Running on a Unix-like system.
Example 6: Platform-Specific Libraries/Modules Import
Here, sys.platform
helps import platform-specific libraries or modules based on the detected platform ('win32'
or others).
Depending on the platform, it imports different modules (ctypes
for Windows and fcntl
for Unix) to utilize platform-specific functionalities or system-level operations.
import sys
if sys.platform == "win32":
import ctypes
# Import Windows-specific library like ctypes for system-related functions
# Use ctypes library functions specific to Windows for certain functionalities
else:
import fcntl
# Import Unix-specific library like fcntl for file control
# Use fcntl library functions specific to Unix for similar functionalities
Use Cases and Considerations
- Cross-Platform Development:
sys.platform
is indispensable when writing code meant to be executed on multiple platforms. It allows developers to create platform-specific functionality or handle differences in behavior or requirements. - Platform-Specific Features: Certain functionalities or libraries might have specific implementations or limitations based on the operating system.
sys.platform
helps in identifying the platform and appropriately utilizing these features or handling limitations. - Conditional Execution: Developers can use conditional statements based on the detected platform to execute platform-specific code blocks or adjust program behavior.
Platform Identifiers
sys.platform
may return various platform identifiers beyond the common ones mentioned earlier. These identifiers are specific to different operating systems or platforms and should be consulted when implementing platform-specific logic or functionality.
Conclusion
The sys.platform
variable in Python is a valuable tool for identifying the underlying operating system or platform where Python code is being executed. It enables developers to write cross-platform code that adapts or behaves differently based on the detected platform.
By leveraging sys.platform
, developers can handle platform-specific functionalities, ensuring their applications run seamlessly across different operating systems.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn